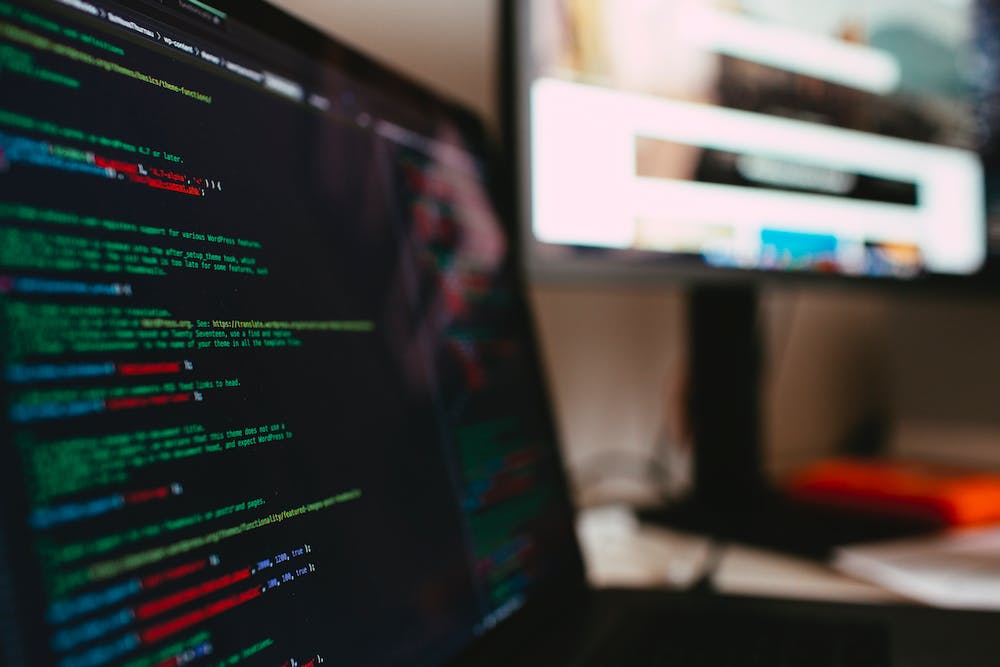
Python is a versatile and powerful programming language that can be used for a wide range of applications. From web development to data analysis, Python has become the go-to language for many developers. In this article, we will focus on one specific application of Python – creating a calculator program.
Why Python?
Python is chosen for this calculator program due to its simplicity and readability. IT‘s a beginner-friendly language with a clean syntax that makes IT easier to understand and write code. With just a few lines of code, we can create a fully functional calculator program!
The Algorithm
Before diving into the coding aspect, let’s quickly understand the algorithm behind the calculator program:
- Take input from the user for the first number.
- Take input from the user for the operator (+, -, *, /).
- Take input from the user for the second number.
- Perform the specified operation on the two numbers.
- Display the result to the user.
Implementing the Calculator Program
Now let’s delve into coding! Open your favorite Python IDE and create a new Python file.
# Python Calculator Program
# Step 1: User inputs the numbers
num1 = float(input("Enter the first number: "))
operator = input("Enter the operator (+, -, *, /): ")
num2 = float(input("Enter the second number: "))
# Step 2: Perform the calculation based on the operator
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
result = num1 / num2
else:
print("Invalid operator!")
# Step 3: Display the result to the user
print("The result is:", result)
With just a few lines of code, we have successfully implemented a calculator program in Python! Run the code to test IT out.
Conclusion
In conclusion, Python provides a simple and elegant solution for creating a calculator program. With its beginner-friendly syntax and versatility, Python enables us to implement complex programs with ease. By mastering this art of simplicity, you can unlock the potential of Python in developing various applications.
FAQs
Q1: Can I use this calculator program for more complex calculations?
A1: Absolutely! The current program performs basic arithmetic operations. However, you can extend IT by adding more operators (e.g., exponentiation, square root) or implementing complex mathematical functions.
Q2: How can I handle division by zero?
A2: In the current implementation, the program will return a ZeroDivisionError if the user attempts to divide by zero. To handle this error, you can include an additional conditional statement to check if the second number is zero before performing the division operation.
Q3: How do I round the result to a specific number of decimal places?
A3: Python provides a built-in function called round() for rounding numbers. You can use this function to round the result to the desired number of decimal places.
Q4: Can I create a graphical user interface (GUI) for this calculator program?
A4: Definitely! Python offers various GUI libraries such as Tkinter and PyQt that allow you to create intuitive and user-friendly interfaces for your calculator program.
Q5: Can I store and retrieve previous calculations in this calculator program?
A5: Yes, you can implement a history feature to store previous calculations using Python data structures such as lists or databases. With this feature, users can review and reuse their calculations whenever needed.