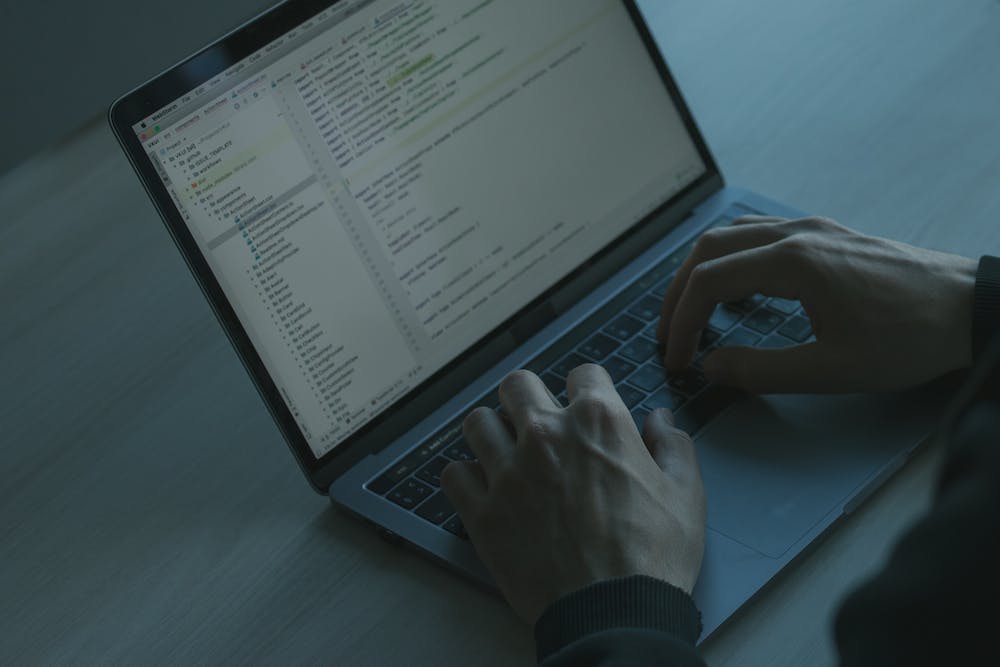
In the world of software development, error detection and correction play a crucial role in ensuring the reliability and integrity of data transmission. One of the most popular techniques for error detection and correction is Hamming code, a simple yet powerful algorithm that can detect and correct single-bit errors in data. In this article, you will learn how to master the art of error detection and correction with Hamming code in Python, a must-know skill for all developers.
Understanding Hamming Code
Hamming code is a linear error-correcting code that adds extra parity bits to the original data bits in order to detect and correct errors. The key idea behind Hamming code is to calculate the parity bits based on the positions of the data bits, and use these parity bits to check for errors and correct them if necessary. With the use of Hamming code, developers can ensure that data is transmitted and stored without any errors, thus improving the overall reliability of the system.
Implementing Hamming Code in Python
To implement Hamming code in Python, you can use the power of programming to create a robust error detection and correction system. By leveraging Python’s built-in data structures and functions, you can easily manipulate data bits and calculate parity bits to detect and correct errors in data transmission. Below is a simple example of how to implement Hamming code in Python:
def hamming_code(data):
# Calculate the number of parity bits required
m = len(data)
r = 0
while 2**r < m + r + 1:
r += 1
code = [0] * (m + r)
j = 0
for i in range(1, m + r + 1):
if i & (i - 1) == 0:
code[i - 1] = 0
else:
code[i - 1] = int(data[j])
j += 1
for i in range(r):
p = 2**i
for j in range(1, m + r + 1):
if j & p == p and j != p:
code[p - 1] ^= code[j - 1]
return code
In this example, the hamming_code
function takes the input data and calculates the parity bits required to detect and correct errors. By using bitwise operations and loops, the function creates a Hamming code for the input data, which can then be transmitted and decoded on the receiving end.
Testing Hamming Code in Python
After implementing the Hamming code in Python, IT‘s important to test the system to ensure that it can effectively detect and correct errors in data transmission. Below is an example of how to test the Hamming code in Python:
data = "1101"
hamming = hamming_code(data)
error = 3
hamming[error - 1] ^= 1
print("Received data:", hamming)
error_position = 0
for i in range(len(hamming)):
if hamming[i] != 0:
error_position += 2**i
print("Error position:", error_position)
if error_position > 0:
hamming[error_position - 1] ^= 1
print("Corrected data:", hamming)
In this test, the input data is “1101”, and we introduce an error at position 3 to simulate a single-bit error in data transmission. The Hamming code is then used to detect and correct the error, and the corrected data is printed to the console. By running this test, you can verify that the Hamming code in Python is capable of detecting and correcting errors in data transmission.
Benefits of Using Hamming Code in Python
There are several benefits to mastering the art of error detection and correction with Hamming code in Python. Some of the key benefits include:
- Reliability: Hamming code can detect and correct single-bit errors, thus improving the overall reliability of data transmission.
- Efficiency: By using Python to implement Hamming code, developers can create a fast and efficient error detection and correction system.
- Scalability: Hamming code can be easily scaled to handle larger data sets, making it suitable for a wide range of applications and industries.
Conclusion
Mastering the art of error detection and correction with Hamming code in Python is a must-know skill for all developers. By understanding the principles of Hamming code and implementing it in Python, developers can create robust error detection and correction systems that improve the reliability and integrity of data transmission. With the ability to detect and correct single-bit errors, Hamming code in Python is a powerful tool that should be in every developer’s toolkit.
FAQs
What is Hamming code?
Hamming code is a linear error-correcting code that adds extra parity bits to the original data bits in order to detect and correct errors.
Why is Hamming code important for developers?
Hamming code is important for developers because it can detect and correct single-bit errors, thus improving the reliability of data transmission.
How can I implement Hamming code in Python?
You can implement Hamming code in Python by using bitwise operations and loops to calculate the parity bits required for error detection and correction.