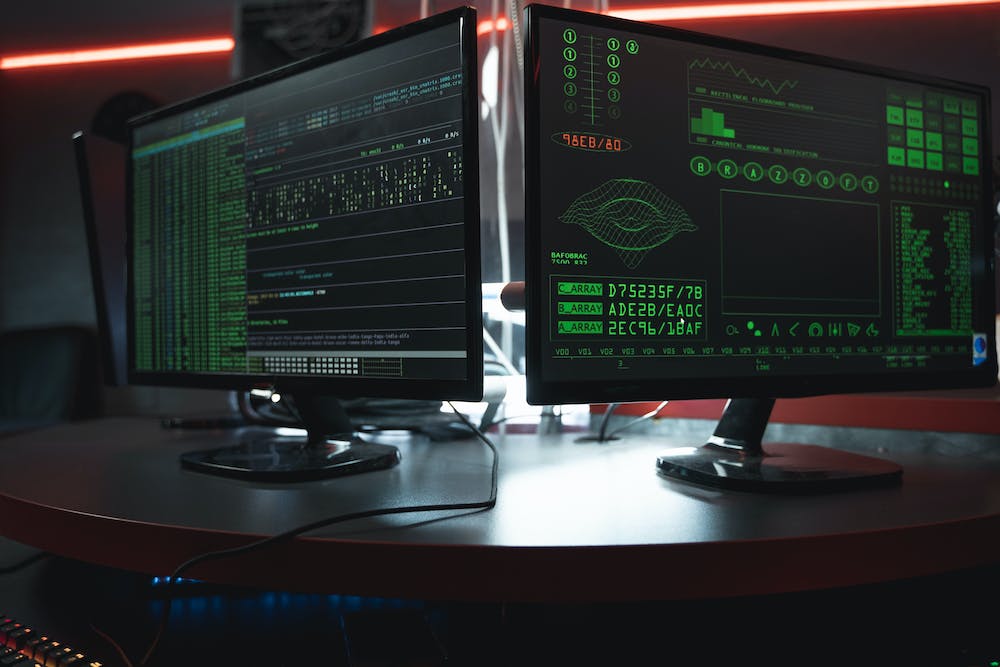
Python is a versatile, high-level programming language that is widely used in various fields such as web development, data science, machine learning, and more. If you want to master Python programming, real-life code examples can help you understand how to code in Python and apply IT to real-world scenarios.
In this article, we will provide you with real-life code examples to help you master Python programming. We will cover the basics of Python syntax, data types, control flow, functions, and more. By the end of this article, you will have a solid understanding of Python programming and be able to write your own Python code for real-life applications.
Python Basics
Before we dive into the real-life code examples, let’s cover the basics of Python programming. Python is known for its simple and easy-to-understand syntax, which makes IT a great language for beginners.
Here is a simple “Hello, World!” program in Python:
print("Hello, World!")
As you can see, the syntax is straightforward, and you don’t need to write a lot of code to achieve a simple output. This is one of the reasons why Python is popular among developers.
Real-Life Code Examples
Now, let’s explore some real-life code examples in Python to help you master the language:
1. Web Scraping
Web scraping is the process of extracting data from websites. Python has powerful libraries such as BeautifulSoup and Scrapy that make web scraping easy. Here’s an example of how to scrape a Website using BeautifulSoup:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
This code uses the requests library to send an HTTP request to the Website and the BeautifulSoup library to parse the HTML. IT then extracts all the links from the Website and prints them out. This is just a basic example, but you can use web scraping for various purposes such as data collection, monitoring, and more.
2. Data Analysis
Python is widely used for data analysis and manipulation. The pandas library is one of the most popular libraries for data analysis in Python. Here’s an example of how to use pandas to analyze a dataset:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [25, 35, 30, 28],
'City': ['New York', 'Chicago', 'Los Angeles', 'Boston']}
df = pd.DataFrame(data)
print(df)
This code creates a simple DataFrame using pandas and then prints IT. With pandas, you can perform various data operations such as filtering, grouping, and visualizing the data. IT’s a powerful library for data analysis in Python.
3. Machine Learning
Python is also widely used in machine learning and artificial intelligence. The scikit-learn library provides various machine learning algorithms and tools in Python. Here’s an example of how to use scikit-learn to train a simple machine learning model:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
iris = datasets.load_iris()
X, y = iris.data, iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
print('Accuracy:', accuracy_score(y_test, y_pred))
This code uses the iris dataset from scikit-learn to train a decision tree classifier and then evaluates its accuracy. With scikit-learn, you can easily build and train machine learning models in Python.
Conclusion
Python is a powerful and versatile programming language that is widely used in various fields. By mastering Python programming and learning from real-life code examples, you can build your own applications, analyze data, and even create machine learning models. The examples provided in this article are just the tip of the iceberg, and there are countless other real-life applications where Python can be used.
Whether you are a beginner or an experienced developer, mastering Python programming can open up a world of opportunities and help you succeed in your career.
FAQs
1. What are some other real-life applications of Python?
Python can be used for web development, automation, scientific computing, game development, and more. Its versatility makes IT a popular choice for a wide range of applications.
2. Is Python a good language for beginners?
Yes, Python is considered a great language for beginners due to its simple and easy-to-understand syntax. IT’s also a popular language in the industry, which makes IT a valuable skill to learn.
3. Where can I find more real-life code examples in Python?
You can find real-life code examples in Python on various platforms such as GitHub, Stack Overflow, and Python documentation. These resources can provide you with practical examples and solutions to real-world problems.
Mastering Python programming with real-life code examples can significantly enhance your skills and open up new opportunities in your career. Whether you’re interested in web development, data analysis, machine learning, or any other field, Python has something to offer. Start practicing with these examples and explore the endless possibilities of Python programming!