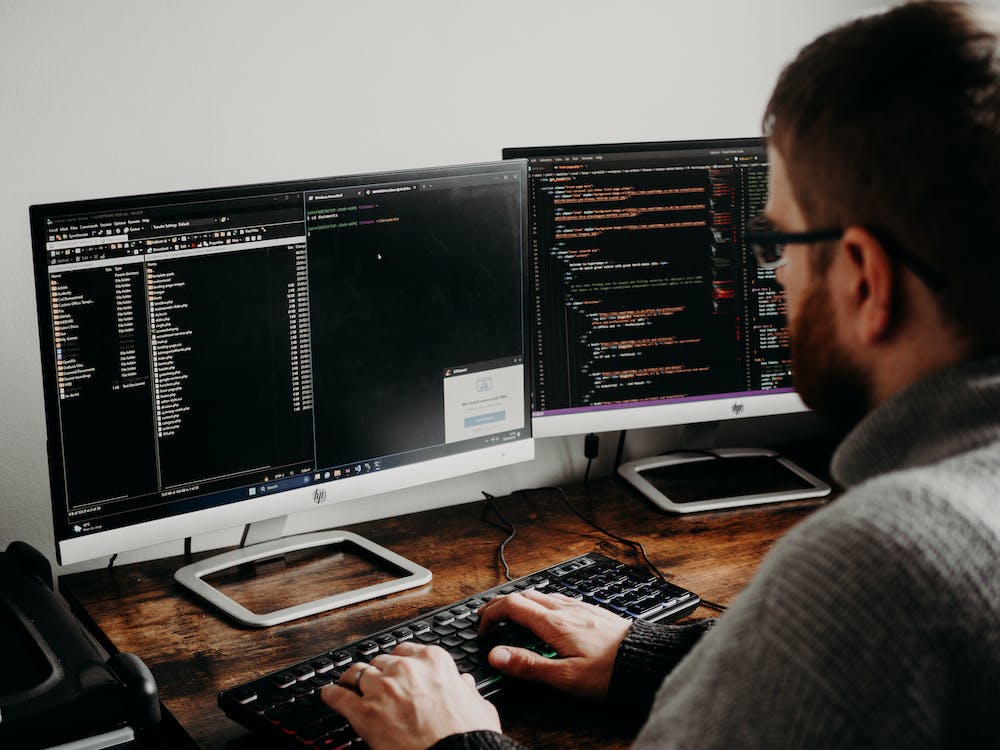
If you’re looking to become a proficient Python programmer, you’re in the right place. Python is a high-level programming language known for its simplicity and elegance, making IT a popular choice for beginners and experienced developers alike. To master Python programming, you need to practice and build your skills through fun and easy programming exercises. In this article, we’ll explore some practice programs that are perfect for beginners to enhance their Python programming skills.
1. Basic Calculator
One of the fundamental programs to start with is a basic calculator. It’s simple yet effective in helping you understand the basic syntax and operations in Python. You can create a program that takes two numbers as input and performs basic arithmetic operations such as addition, subtraction, multiplication, and division. Here’s an example:
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
sum = num1 + num2
difference = num1 - num2
product = num1 * num2
quotient = num1 / num2
print("Sum: ", sum)
print("Difference: ", difference)
print("Product: ", product)
print("Quotient: ", quotient)
By creating this program, you will grasp the concepts of input/output, variable declaration, and basic arithmetic operations in Python.
2. Guess the Number Game
Another fun and interactive program to practice Python programming is the “Guess the Number” game. This program involves generating a random number and asking the user to guess it within a certain range. Here’s an example of how it can be implemented:
import random
number_to_guess = random.randint(1, 100)
guessed = False
while not guessed:
guess = int(input("Guess the number between 1 and 100: "))
if guess == number_to_guess:
print("Congratulations! You guessed the correct number!")
guessed = True
elif guess < number_to_guess:
print("Try guessing a higher number.")
else:
print("Try guessing a lower number.")
This program allows you to practice using loops, conditional statements, and random number generation in Python.
3. To-Do List Application
Building a to-do list application is a great way to practice Python programming by creating a simple user interface and managing tasks. You can use the “tkinter” library to create a graphical user interface (GUI) for the to-do list application. Here’s an example:
import tkinter as tk
def add_task():
task = entry.get()
if task:
tasks.append(task)
update_listbox()
def delete_task():
task = tasks_listbox.get(tk.ACTIVE)
if task in tasks:
tasks.remove(task)
update_listbox()
def update_listbox():
tasks_listbox.delete(0, tk.END)
for task in tasks:
tasks_listbox.insert(tk.END, task)
tasks = []
root = tk.Tk()
tasks_listbox = tk.Listbox(root)
tasks_listbox.pack()
entry = tk.Entry(root)
entry.pack()
add_button = tk.Button(root, text="Add Task", command=add_task)
add_button.pack()
delete_button = tk.Button(root, text="Delete Task", command=delete_task)
delete_button.pack()
root.mainloop()
By creating this program, you will learn about creating a GUI, event handling, and list manipulation in Python.
4. Word Count Tool
Practicing Python programming also involves dealing with file handling and string manipulation. A word count tool is a simple program that counts the occurrences of each word in a text file. Here’s an example of how you can implement it:
text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur."
words = text.split()
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
for word, count in word_count.items():
print(f"{word}: {count}")
By creating this program, you will understand string manipulation, dictionary data structure, and file handling in Python.
5. Web Scraper
Web scraping is a useful skill to have as a Python programmer. You can practice web scraping by creating a program that extracts data from a Website. For example, you can create a web scraper that extracts the top headlines from a news website. Here’s an example using the “Beautiful Soup” library:
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com/news"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
headlines = soup.find_all("h2", class_="headline")
for headline in headlines:
print(headline.text)
By creating this program, you will learn about making HTTP requests, parsing HTML content, and extracting data from websites using Python.
Conclusion
Practicing Python programming through fun and easy programs is an effective way to improve your skills and become proficient in the language. By working on basic calculator, guess the number game, to-do list application, word count tool, and web scraper, you will develop a solid understanding of fundamental programming concepts such as variables, loops, conditional statements, file handling, and data manipulation. These exercises will also help you in building more complex and real-world applications in Python.
FAQs
Q: Are these practice programs suitable for beginners?
A: Yes, these practice programs are specifically designed for beginners to gradually build their Python programming skills.
Q: Do I need any prior programming experience to practice these programs?
A: No, these programs are meant to be beginner-friendly and do not require any prior programming experience.
Q: Can I find additional resources to practice Python programming?
A: Yes, there are numerous online platforms, tutorials, and practice websites that offer a wide range of Python programming exercises for beginners.
Q: How can I track my progress in mastering Python programming?
A: You can track your progress by solving more complex programming challenges, participating in coding competitions, and building your own projects using Python.