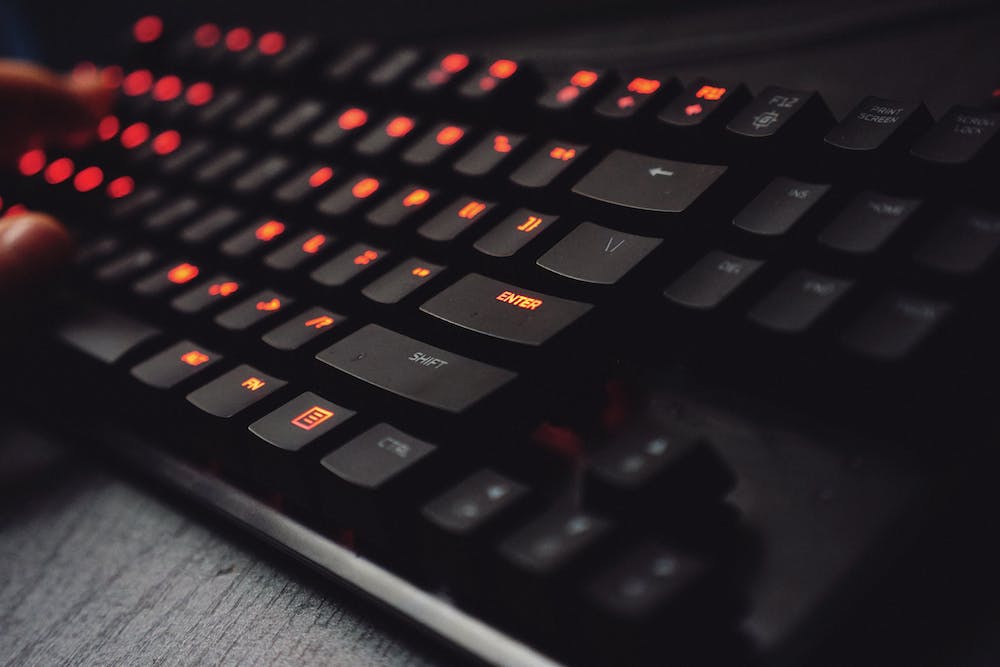
PHP is a versatile programming language widely used for web development. One of its key features is the ability to manipulate strings and convert them into arrays for easier data processing. In this article, we will explore various techniques for manipulating PHP strings to create arrays, along with examples and best practices.
Exploding Strings into Arrays
The explode()
function in PHP is used to split a string into an array based on a specified delimiter. The syntax for using explode()
is as follows:
$string = "apple,banana,orange";
$fruits = explode(",", $string);
print_r($fruits);
In this example, the string "apple,banana,orange"
is split into an array using the comma (,) as the delimiter. The resulting array $fruits
will contain ['apple', 'banana', 'orange']
.
Imploding Arrays into Strings
On the other hand, the implode()
function in PHP is used to join elements of an array into a string. The syntax for using implode()
is as follows:
$fruits = ['apple', 'banana', 'orange'];
$string = implode(",", $fruits);
echo $string;
In this example, the array of fruits $fruits
is merged into a single string using the comma (,) as the glue. The resulting string $string
will be "apple,banana,orange"
.
Using Regular Expressions to Split Strings
Regular expressions can be employed to split a string into an array based on more complex patterns. PHP provides the preg_split()
function for this purpose. The syntax for using preg_split()
is as follows:
$string = "The quick brown fox jumps over the lazy dog";
$words = preg_split("/\s+/", $string);
print_r($words);
In this example, the string "The quick brown fox jumps over the lazy dog"
is split into an array using a regular expression pattern that matches one or more whitespace characters. The resulting array $words
will contain all the individual words in the original string.
Converting Strings to Arrays of Characters
PHP allows for easy conversion of a string into an array of its individual characters using the str_split()
function. The syntax for using str_split()
is as follows:
$string = "Hello, world!";
$chars = str_split($string);
print_r($chars);
In this example, the string "Hello, world!"
is converted into an array where each element represents a single character in the original string. The resulting array $chars
will contain all the characters in the original string as separate elements.
Optimizing PHP String Manipulation for Performance
When dealing with large strings and complex manipulations, IT is important to optimize PHP string operations for performance. Here are a few best practices to consider:
- Use single quotes for string literals: When working with strings that do not require variable interpolation, it is recommended to use single quotes instead of double quotes for better performance.
- Avoid excessive concatenation: Concatenating multiple strings with the dot (.) operator can be inefficient, especially within loops. Consider using
implode()
for joining array elements into a string instead. - Cache repeated regular expressions: If you are using regular expressions repeatedly within a script, consider caching the compiled regular expressions using
preg_match()
for better performance. - Handle multibyte strings carefully: When working with multibyte character encodings such as UTF-8, be aware that some string functions may not behave as expected. Use the multibyte string functions provided by the
mbstring
extension for proper handling.
Conclusion
Manipulating PHP strings to create arrays is a fundamental aspect of data processing in web development. Whether it’s splitting a string into an array of substrings or joining array elements into a single string, PHP offers a variety of functions and techniques for efficient string manipulation. By understanding these capabilities and optimizing string operations for performance, developers can effectively work with textual data in their PHP applications.
FAQs
Q: Can I use regular expressions with explode()
?
A: No, the explode()
function only allows for simple string delimiters. If you need to use regular expressions, consider using the preg_split()
function instead.
Q: Are there any limitations to the size of strings that can be manipulated in PHP?
A: PHP imposes a memory_limit on the size of strings that can be processed, which can be configured in the php.ini configuration file. It is important to be mindful of memory usage when working with large strings.
Q: How can I efficiently join array elements into a string?
A: The implode()
function is the most efficient way to join array elements into a string in PHP, especially for large arrays.
Q: Does PHP have built-in support for multibyte string manipulation?
A: Yes, PHP provides the mbstring
extension for working with multibyte character encodings such as UTF-8. It offers a set of functions for handling multibyte strings and is essential for internationalization and localization of web applications.
Q: Is there a performance difference between using single quotes and double quotes for strings in PHP?
A: Yes, using single quotes for string literals is generally more efficient in PHP, as it avoids the overhead of parsing for variable interpolation. However, the difference in performance may be negligible for most applications.