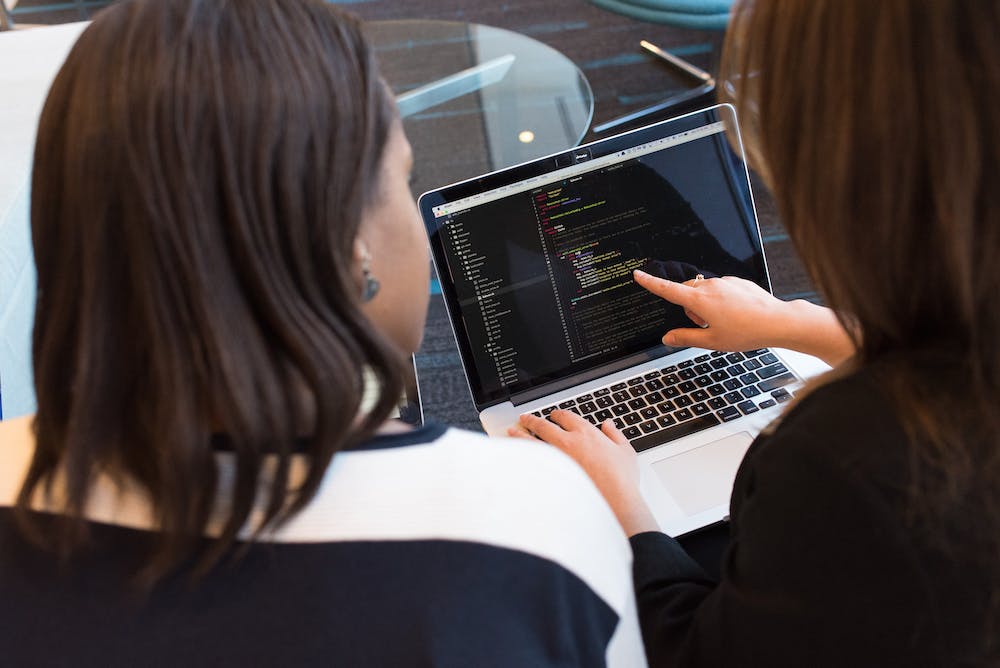
Are you interested in learning how to create your own Tic Tac Toe game in Python? Whether you’re a beginner programmer or just looking to try your hand at game development, creating a simple Tic Tac Toe game is a great way to practice your skills. In this article, we’ll walk you through the process of creating your own Tic Tac Toe game in Python, step by step. By the end of this tutorial, you’ll have a fully functioning Tic Tac Toe game that you can play with your friends and family!
Why Learn to Create a Tic Tac Toe Game in Python?
Creating a Tic Tac Toe game in Python is a great way to learn the basics of programming. IT‘s a simple yet fun game that can be implemented using fundamental programming concepts such as loops, conditionals, and functions. By creating a Tic Tac Toe game, you’ll gain a better understanding of these concepts and improve your programming skills.
Additionally, creating a Tic Tac Toe game can also serve as a starting point for more complex game development projects. Once you’ve mastered the basics of creating a simple game like Tic Tac Toe, you can use the same principles to create more advanced games in the future.
Prerequisites
Before we dive into creating our Tic Tac Toe game, there are a few prerequisites that you’ll need to have in place. Make sure you have the following:
- A basic understanding of Python programming. If you’re new to Python, you can start by learning the basics of the language through online tutorials or courses.
- A code editor such as Visual Studio Code, Sublime Text, or PyCharm. This will be used to write and run your Python code.
Step 1: Setting Up Your Development Environment
First, open your code editor and create a new Python file for your Tic Tac Toe game. Save the file with a meaningful name, such as “tic_tac_toe.py”. This will be the main file where you’ll write your game code.
Step 2: Designing the Game Grid
The first step in creating our Tic Tac Toe game is to design the game grid. In Tic Tac Toe, the game is played on a 3×3 grid, and each grid cell can be either empty, marked with an “X”, or marked with an “O”. To represent the game grid in our Python code, we can use a 2D list.
# Create the game grid
game = [
["_", "_", "_"],
["_", "_", "_"],
["_", "_", "_"]
]
In the above code, we’ve created a 2D list called “game” to represent the game grid. We’ve initialized all the cells with the “_” character to indicate that they are empty.
Step 3: Displaying the Game Grid
Next, we’ll write a function to display the game grid to the players. This function will iterate through the 2D list and print the contents of each cell to the console. We’ll also number the rows and columns to make it easier for the players to choose their moves.
def display_game():
for row in game:
print("|".join(row))
Now, whenever we want to display the game grid, we can simply call the “display_game()” function, and it will print the grid to the console.
Step 4: Accepting Player Input
After displaying the game grid, we need to write code to accept the players’ input for their moves. We’ll allow the players to enter the row and column number (between 1 and 3) where they want to place their mark.
def get_player_move(player):
print(f"Player {player}'s turn")
row = int(input("Enter the row number (1-3): ")) - 1
col = int(input("Enter the column number (1-3): ")) - 1
return (row, col)
In the “get_player_move()” function, we prompt the player to enter the row and column numbers and then return the coordinates as a tuple.
Step 5: Validating Player Moves
Before allowing a player to make a move, we need to validate that the chosen cell is empty. We’ll write a function to check if the chosen cell is empty and return a boolean value indicating whether the move is valid or not.
def is_valid_move(row, col):
if 0 <= row <= 2 and 0 <= col <= 2 and game[row][col] == "_":
return True
else:
print("Invalid move. Please try again.")
return False
The “is_valid_move()” function checks that the row and column numbers are within the valid range (0-2) and that the chosen cell is empty. If these conditions are met, the function returns True, indicating a valid move. Otherwise, it returns False and prompts the player to try again.
Step 6: Implementing the Game Logic
Now that we have the basic functions in place, we can write the main game logic. We’ll create a loop that allows the players to take turns making their moves, validate each move, and check for a winner after each turn.
def tic_tac_toe():
display_game()
turn = 1
while True:
if turn % 2 != 0:
player = 1
mark = "X"
else:
player = 2
mark = "O"
print("\n")
row, col = get_player_move(player)
if is_valid_move(row, col):
game[row][col] = mark
display_game()
if check_winner(mark):
print(f"Player {player} wins!")
break
if turn == 9:
print("It's a tie!")
break
turn += 1
The “tic_tac_toe()” function includes the main game loop, where players take turns making their moves and the game grid is displayed after each turn. The “check_winner()” function, which we’ll implement next, is called to check if a player has won the game. The game loop continues until a player wins or the game ends in a tie.
Step 7: Checking for a Winner
To determine if a player has won the game, we need to check each row, column, and diagonal for matching marks. We’ll write a function to perform these checks and return True if a player has won.
def check_winner(mark):
for i in range(3):
# Check rows
if game[i][0] == game[i][1] == game[i][2] == mark:
return True
# Check columns
if game[0][i] == game[1][i] == game[2][i] == mark:
return True
# Check diagonals
if game[0][0] == game[1][1] == game[2][2] == mark or game[0][2] == game[1][1] == game[2][0] == mark:
return True
return False
The “check_winner()” function iterates through the rows, columns, and diagonals of the game grid to check for matching marks. If it finds a matching sequence, it returns True, indicating that a player has won the game.
Step 8: Playing the Game
Now that we’ve implemented all the game logic, we can call the “tic_tac_toe()” function to start playing the game. In your main Python file, add the following code at the end:
if __name__ == "__main__":
tic_tac_toe()
When you run your Python file, the Tic Tac Toe game will start, and players can take turns playing until a player wins or the game ends in a tie.
Conclusion
Congratulations! You’ve successfully created your own Tic Tac Toe game in Python. By following this step-by-step tutorial, you’ve learned how to design the game grid, accept player input, validate moves, implement game logic, and check for a winner. You can now play your Tic Tac Toe game with friends and family, or further customize it by adding features such as a graphical interface, AI opponents, or networked multiplayer support.
Creating a simple game like Tic Tac Toe is a great way for beginners to practice their programming skills and gain confidence in their ability to create fun and interactive applications. If you enjoyed creating this game, consider exploring more complex game development projects to further expand your skills and knowledge.
FAQs
Q: Can I customize the game grid size?
A: Yes, you can modify the size of the game grid by changing the dimensions of the 2D list that represents the grid. Keep in mind that you’ll also need to adjust the game logic and winner-checking functions to accommodate the new grid size.
Q: How can I add a graphical interface to the game?
A: You can use Python libraries such as Tkinter or Pygame to create a graphical interface for your Tic Tac Toe game. These libraries provide tools for creating windows, buttons, and other graphical elements that can enhance the user experience.
Q: Is it possible to add AI opponents to the game?
A: Yes, you can implement AI opponents by creating algorithms that determine the AI’s moves based on the current game state. This can add a new level of challenge to the game by allowing players to compete against computer-controlled opponents.
Q: Where can I find more resources on game development in Python?
A: You can find a wealth of tutorials, courses, and community forums dedicated to game development in Python. Websites like Codecademy, Coursera, and Stack Overflow are great places to start exploring more advanced game development concepts and techniques.
Now that you’ve learned how to create your own Tic Tac Toe game in Python, take the next step in your programming journey by exploring new projects, learning from experienced developers, and continuously challenging yourself to improve your skills. Happy coding!