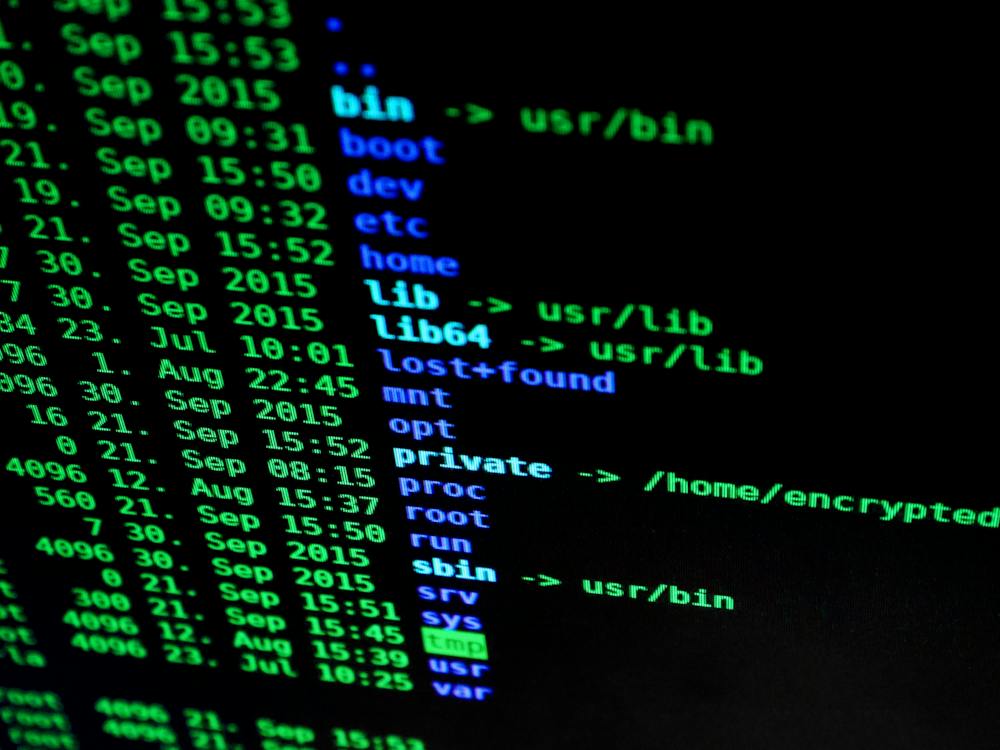
Creating folders in PHP can be a crucial aspect of web development, especially when IT comes to organizing files and data. The mkdir function in PHP provides a simple and effective way to create directories, but there are a few secret tricks that can make the process even easier and more efficient.
Understanding the Basics of mkdir
Before diving into the secret trick, let’s first understand the basics of the mkdir function in PHP. This function is used to create a directory, and it takes two parameters – the directory path and the optional permissions.
The basic syntax of the mkdir function is:
bool mkdir ( string $directory, int $permissions = 0777, bool $recursive = false, resource $context = null )
The $directory parameter specifies the path of the new directory that will be created. The $permissions parameter is optional and specifies the permissions of the new directory. If not specified, the default permissions are set to 0777. The $recursive parameter is also optional and allows creating the specified directory path recursively. Finally, the $context parameter is also optional and is used to modify the behavior of the mkdir function.
The Secret Trick to Creating Folders with PHP mkdir
Now, let’s unveil the secret trick to making the folder creation process in PHP even easier. The trick is to use the mkdir function along with the dirname function to create nested directories in a single line of code.
The dirname function in PHP returns the parent directory’s path of a specified path. When combined with the mkdir function, it allows for the creation of nested directories without the need to create each directory individually.
Here’s an example of how to use the secret trick to create nested directories:
$path = 'images/photos';
mkdir(dirname($path), 0777, true);
In this example, the mkdir function is used alongside the dirname function to create a nested directory structure. The dirname function returns the parent directory of the specified path ‘images/photos’, which in this case is ‘images’. The mkdir function then creates the ‘images’ directory if it does not already exist, and the ‘photos’ directory is created inside it due to the true value of the $recursive parameter.
By using this secret trick, you can significantly simplify the process of creating nested directories in PHP and improve the efficiency of your code.
Best Practices for Using the mkdir Function
While the mkdir function provides a convenient way to create directories in PHP, it’s essential to follow best practices to ensure secure and efficient folder creation. Here are a few best practices for using the mkdir function:
- Verify the existence of the directory before creating it to avoid overwriting existing directories.
- Sanitize user input if the directory path is based on user input to prevent directory traversal attacks.
- Provide appropriate permissions to the newly created directories to control access and prevent unauthorized modifications.
- Use the recursive option carefully to avoid unintentionally creating a large number of nested directories.
By following these best practices, you can ensure that your folder creation process is secure, reliable, and optimized for performance.
Examples of Creating Folders with PHP mkdir
Let’s explore a few examples of creating folders using the mkdir function in PHP.
Example 1: Creating a Single Directory
$path = 'uploads';
if (!file_exists($path)) {
mkdir($path, 0777, true);
}
In this example, the mkdir function is used to create a directory with the path ‘uploads’. Before creating the directory, the file_exists function is used to verify its existence to prevent overwriting an existing directory with the same name.
Example 2: Creating Nested Directories
$path = 'documents/invoices/2022';
mkdir(dirname($path), 0777, true);
In this example, the secret trick of using the dirname function alongside the mkdir function is employed to create nested directories. The dirname function returns the parent directory of the specified path, and the mkdir function creates the nested directories if they do not already exist.
Conclusion
Creating folders with PHP mkdir is a simple yet powerful feature that can be incredibly useful for organizing files and data. By understanding the basics of the mkdir function and leveraging the secret trick of using the dirname function, you can streamline the process of creating nested directories and improve the efficiency of your code. Be sure to follow best practices for secure and optimized folder creation, and you’ll be well-equipped to handle directory management in your PHP projects.
FAQs
How can I check if a directory exists before creating it?
You can use the file_exists function in PHP to verify the existence of a directory before creating it. This will help prevent overwriting an existing directory with the same name.
Is it possible to create multiple nested directories in a single line of code?
Yes, you can use the secret trick of combining the dirname function with the mkdir function to create multiple nested directories in a single line of code. This can significantly simplify the process of creating directory structures in PHP.
What permissions should I provide to the newly created directories?
It’s important to provide appropriate permissions to the newly created directories to control access and prevent unauthorized modifications. The default permissions are set to 0777, but you can adjust them based on your specific requirements for directory access.