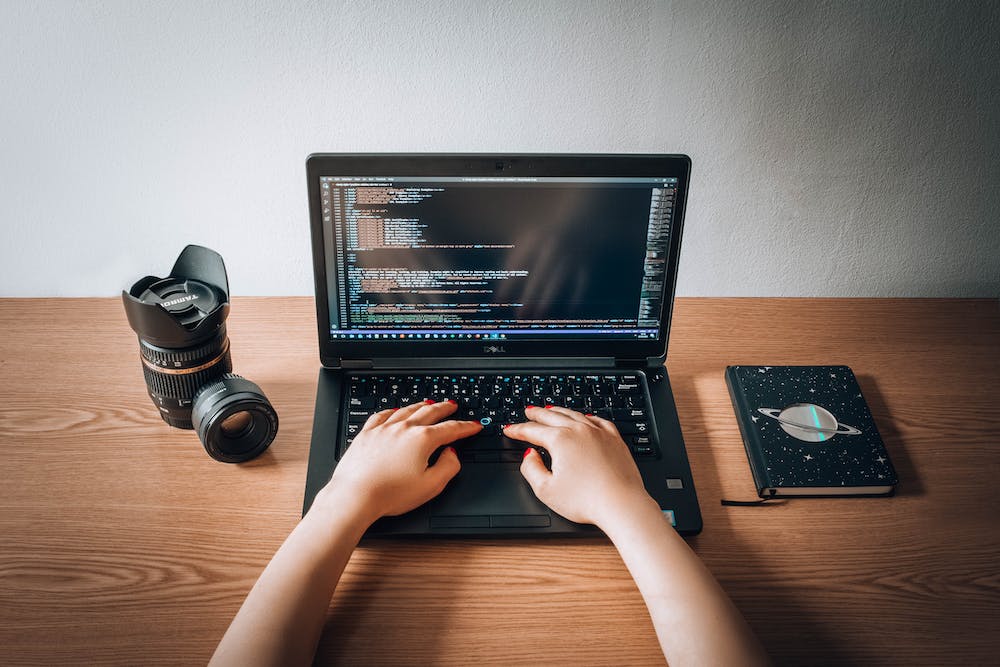
PHP is a popular programming language used for web development. One essential aspect of web development is URL handling. In this article, we will explore the secret to mastering PHP URL handling in just 5 minutes! By understanding the fundamentals of URL handling in PHP, you can take your web development skills to the next level. Let’s dive in!
The Basics of URL Handling in PHP
URL handling in PHP involves manipulating and interpreting URLs to extract information and control the behavior of web applications. URLs (Uniform Resource Locators) are the addresses used to access resources on the web, such as web pages, images, and other media.
When a user requests a URL, the web server uses the URL to determine which resource to serve. In the context of PHP, URL handling is crucial for routing requests to the appropriate PHP scripts and processing the data contained in the URL.
Understanding the Components of a URL
A standard URL consists of several components, including the protocol (e.g., http, https), the domain name (e.g., example.com), the path to the resource, query parameters, and fragments. Let’s break down each component:
- Protocol: The protocol specifies the method used to access the resource, such as HTTP or HTTPS.
- Domain Name: This is the unique name that identifies a Website, such as google.com or facebook.com.
- Path: The path indicates the location of the resource on the web server. For example, in the URL https://example.com/blog/article.php, the path is /blog/article.php.
- Query Parameters: Query parameters are used to pass data to the server. They appear after the “?” in the URL and are in the form key=value pairs, separated by “&”. For example, in the URL https://example.com/search?q=php, the query parameter is “q=php”.
- Fragments: Fragments are used to specify a specific section within a web page. They appear after the “#” in the URL. For example, in the URL https://example.com/about#team, the fragment is “team”.
PHP Functions for URL Handling
PHP provides several built-in functions for working with URLs. These functions enable developers to parse, manipulate, and generate URLs dynamically. Some of the key functions for URL handling in PHP include:
- parse_url(): This function parses a URL and returns an associative array containing its components, such as the scheme, host, path, query, and fragment.
- http_build_query(): This function generates a URL-encoded query string from an associative array of parameters.
- urlencode(): This function encodes a string to be used in a URL, ensuring that special characters are properly represented.
- urldecode(): This function decodes a URL-encoded string, converting special characters back to their original form.
Mastering URL Handling in 5 Minutes
Now that we understand the basics of URL components and PHP functions for handling URLs, let’s dive into the secret to mastering PHP URL handling in just 5 minutes! The key to mastering URL handling is to practice using these functions and understanding how to manipulate URLs to achieve specific goals.
Example 1: Parsing a URL
Let’s start with a basic example of parsing a URL using the parse_url() function:
“`php
$url = “https://example.com/blog/article.php?id=123”;
$parsed_url = parse_url($url);
echo “Scheme: ” . $parsed_url[‘scheme’] . “
“;
echo “Host: ” . $parsed_url[‘host’] . “
“;
echo “Path: ” . $parsed_url[‘path’] . “
“;
echo “Query: ” . $parsed_url[‘query’] . “
“;
“`
In this example, we parse the URL “https://example.com/blog/article.php?id=123” and extract its components using parse_url(). This allows us to access the scheme, host, path, and query parameters of the URL.
Example 2: Building a URL with Query Parameters
Now, let’s use the http_build_query() function to generate a URL with query parameters:
“`php
$params = array(
‘page’ => ‘blog’,
‘id’ => 123
);
$url = “https://example.com?” . http_build_query($params);
echo $url;
“`
In this example, we create a URL with the query parameters “page=blog” and “id=123” using http_build_query(). This demonstrates how to construct dynamic URLs with query parameters.
Example 3: Encoding and Decoding URL Components
Lastly, let’s look at how to encode and decode URL components using urlencode() and urldecode():
“`php
$search_query = “mastering PHP URL handling”;
$encoded_query = urlencode($search_query);
echo “Encoded query: ” . $encoded_query . “
“;
$decoded_query = urldecode($encoded_query);
echo “Decoded query: ” . $decoded_query;
“`
In this example, we encode the search query “mastering PHP URL handling” using urlencode(), and then decode IT back to its original form using urldecode(). This is useful for dealing with special characters in URLs.
Conclusion
In just 5 minutes, you’ve learned the secret to mastering PHP URL handling! By understanding the components of a URL and utilizing PHP’s built-in functions for URL manipulation, you can effectively handle and manipulate URLs in your web applications. Practice and experimentation are key to mastering URL handling, so be sure to explore the various ways you can leverage PHP’s URL handling capabilities in your projects.
FAQs
Q: Can I use PHP to redirect users to a different URL?
A: Yes, you can use the header() function in PHP to send a raw HTTP header and perform a redirect. For example, to redirect users to a different page, you can use the following code:
“`php
header(“Location: https://example.com/newpage.php”);
exit();
“`
Q: Is it necessary to sanitize and validate user input in URLs?
A: Yes, it is crucial to sanitize and validate user input in URLs to prevent security vulnerabilities, such as SQL injection and cross-site scripting (XSS). Always validate and sanitize user input before using it in URLs or processing it in your application.
Q: How can I handle friendly URLs (e.g., example.com/blog/post-title) in PHP?
A: To handle friendly URLs in PHP, you can use URL rewriting techniques, such as mod_rewrite in Apache or URL routing in frameworks like Laravel or Symfony. These techniques allow you to map friendly URLs to specific PHP scripts or controllers for processing.