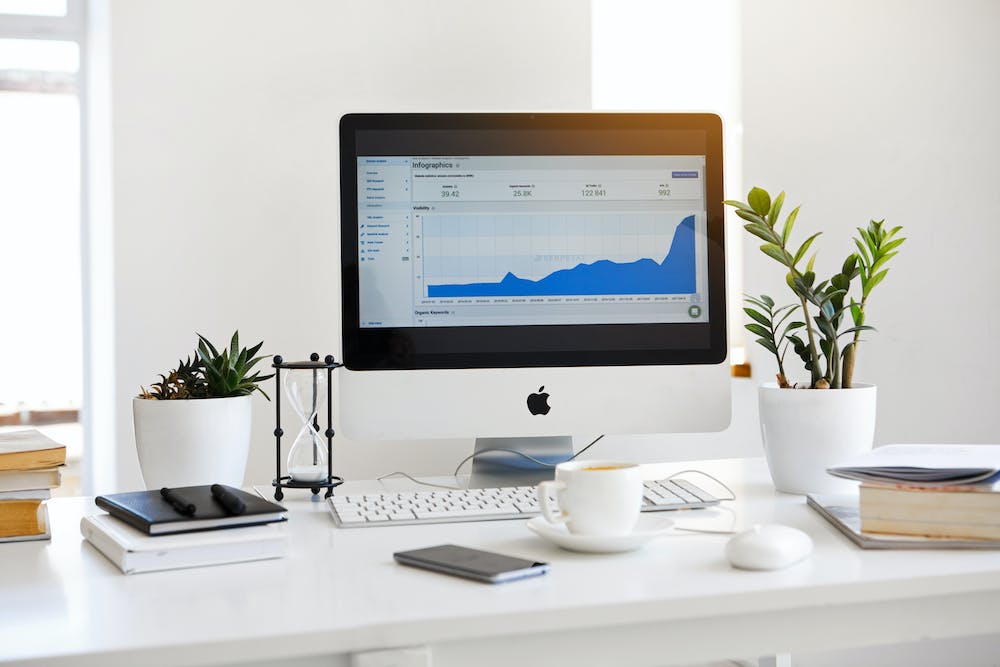
Sorting is a fundamental operation in computer science and is required in various applications. While there are many sorting algorithms available, one of the most efficient and widely used algorithms is the Quick Sort algorithm. In this article, we will explore the Quick Sort algorithm and learn how to implement IT in Python to achieve lightning-fast sorting.
Understanding the Quick Sort Algorithm
The Quick Sort algorithm is a comparison-based sorting algorithm that works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted. The key to the efficiency of the Quick Sort algorithm lies in its partitioning process, which allows it to sort the array with very little additional space and is also relatively easy to implement.
Implementing Quick Sort in Python
Now that we have an understanding of how the Quick Sort algorithm works, let’s take a look at how we can implement it in Python.
def quick_sort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[0]
less_than_pivot = [x for x in arr[1:] if x <= pivot]
greater_than_pivot = [x for x in arr[1:] if x > pivot]
return quick_sort(less_than_pivot) + [pivot] + quick_sort(greater_than_pivot)
With just a few lines of code, we have implemented the Quick Sort algorithm in Python. The ‘quick_sort’ function takes an array as input and recursively sorts it using the Quick Sort algorithm.
Testing Quick Sort in Python
Now that we have the Quick Sort algorithm implemented in Python, let’s test it with a sample array to see how efficient it is in action.
arr = [3, 6, 8, 10, 1, 2, 1]
sorted_arr = quick_sort(arr)
print(sorted_arr)
When we run the above code, we should see the output ‘[1, 1, 2, 3, 6, 8, 10]’, which is the sorted version of the input array. This demonstrates the effectiveness of the Quick Sort algorithm in sorting an array of integers.
Benefits of Quick Sort Algorithm
There are several benefits of using the Quick Sort algorithm:
- Efficiency: Quick Sort is one of the fastest sorting algorithms, with an average time complexity of O(n log n).
- In-place sorting: Quick Sort sorts the array in place, requiring only a small amount of additional memory.
- Easy to implement: The Quick Sort algorithm is relatively easy to implement, making it a popular choice for sorting large datasets.
Conclusion
The Quick Sort algorithm is a powerful and efficient sorting algorithm that is widely used in practice. By understanding how the Quick Sort algorithm works and how to implement it in Python, you can leverage its speed and efficiency to sort large datasets with ease. Whether you are a beginner or an experienced programmer, mastering the Quick Sort algorithm is an essential skill that will benefit you in various programming tasks and projects.
FAQs
Q: Is Quick Sort the fastest sorting algorithm?
A: While Quick Sort is one of the fastest sorting algorithms, its performance can vary depending on the input data. In general, Quick Sort has an average time complexity of O(n log n), which makes it highly efficient for sorting large datasets.
Q: Can Quick Sort be used to sort different types of data?
A: Yes, Quick Sort can be used to sort arrays of different data types, including integers, floating-point numbers, strings, and custom objects, as long as a comparison operation is defined for the data type.
Q: Are there any drawbacks to using Quick Sort?
A: One potential drawback of Quick Sort is its worst-case time complexity of O(n^2) when the input array is already sorted or nearly sorted. However, this can be mitigated by choosing a good pivot element and using randomized pivot selection.