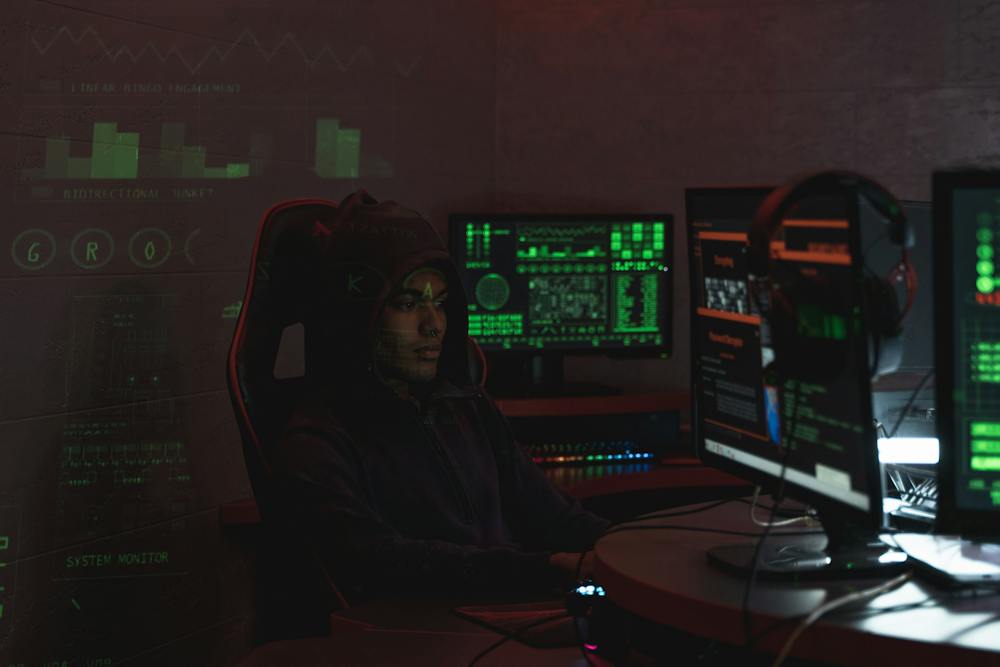
When working with data in Python, one of the key challenges is encoding categorical variables. One popular method for doing so is hot encoding, which is used to convert categorical variables into a numerical format that can be used in machine learning algorithms. In this article, we will explore the concept of hot encoding, its implementation in Python, and some best practices for efficient data encoding.
Understanding Hot Encoding
Hot encoding, also known as one-hot encoding, is a process of converting categorical variables into a binary format. This means that each category is represented by a binary vector, where only one element is “hot” (i.e., has a value of 1) while the rest are “cold” (i.e., have a value of 0).
For example, suppose we have a categorical variable “color” with three categories: red, green, and blue. After hot encoding, the variable would be represented as follows:
- Red: [1, 0, 0]
- Green: [0, 1, 0]
- Blue: [0, 0, 1]
This allows us to represent categorical data in a way that is easily understandable by machine learning algorithms.
Implementing Hot Encoding in Python
Python provides several libraries for working with hot encoding, including pandas and scikit-learn. Let’s take a look at how hot encoding can be implemented using pandas:
import pandas as pd
# Create a DataFrame with categorical variables
data = {'color': ['red', 'green', 'blue', 'red']}
df = pd.DataFrame(data)
# Use get_dummies function to hot encode the variable
encoded_df = pd.get_dummies(df, columns=['color'])
print(encoded_df)
In this example, the get_dummies function from the pandas library is used to hot encode the “color” variable. The resulting DataFrame will have separate columns for each category, with binary values representing the presence of each category.
Best Practices for Efficient Data Encoding
While hot encoding is a powerful technique for representing categorical data, there are some best practices that can help ensure efficient data encoding:
- Consider the cardinality of the categorical variable: If a variable has a large number of unique categories, hot encoding can result in a high-dimensional and sparse representation, which can be computationally expensive. In such cases, IT may be better to use other encoding techniques or to reduce the cardinality of the variable.
- Handle new categories appropriately: When new categories are encountered during prediction, it’s important to have a strategy for handling them. This can include ignoring new categories, mapping them to a default category, or using techniques such as target encoding.
- Use feature scaling: Hot encoding can result in variables with vastly different scales, which can affect the performance of machine learning algorithms. It’s important to use feature scaling techniques, such as standardization or normalization, to ensure that all variables have a similar scale.
Conclusion
Hot encoding is a valuable tool for converting categorical variables into a numerical format that can be used in machine learning algorithms. By understanding the concept of hot encoding and following best practices for efficient data encoding, you can ensure that your data is properly represented and ready for analysis.
FAQs
What are the drawbacks of hot encoding?
One drawback of hot encoding is the potential for high-dimensional and sparse representations, especially when dealing with categorical variables with a large number of unique categories. This can result in increased computational complexity and memory usage.
Can hot encoding handle missing values?
Hot encoding can handle missing values by creating a separate dummy variable for missing values. However, it’s important to consider the impact of missing values on the overall dataset and to handle them appropriately, such as through imputation or the use of specialized techniques like target encoding.
How does hot encoding compare to other encoding techniques?
Hot encoding is just one of several techniques for encoding categorical variables, and its suitability depends on the specific characteristics of the data. Other techniques, such as label encoding and target encoding, may be more appropriate in certain situations, and it’s important to consider the trade-offs of each technique when encoding categorical data.
Is feature scaling necessary after hot encoding?
Feature scaling is important after hot encoding, as it can help ensure that all variables have a similar scale, which can improve the performance of machine learning algorithms. Techniques such as standardization and normalization can be applied to the hot encoded variables to achieve this.
Where can I learn more about hot encoding and other data encoding techniques?
There are many online resources, tutorials, and courses available for learning more about hot encoding and other data encoding techniques. Additionally, you can consider seeking guidance from experts or enrolling in specialized data science programs that cover these topics in detail.