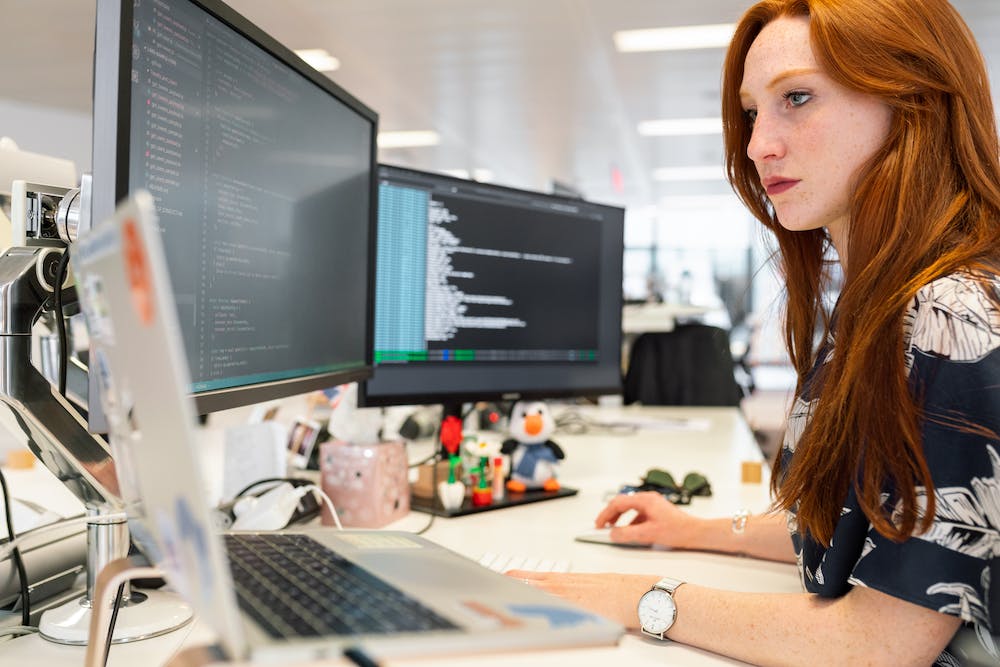
Are you tired of writing the same repetitive code over and over again in your PHP projects? Do you want to learn a powerful technique that will make your code more efficient and easier to maintain? Look no further! In this article, we will dive deep into the secret PHP do while loop technique that will revolutionize your coding and take your programming skills to the next level.
What is a Do While Loop?
Before we get into the secret technique, let’s first understand what a do while loop is and how IT differs from a regular while loop. In PHP, a do while loop is a control structure that allows you to execute a block of code repeatedly as long as a specified condition is true. The key difference between a do while loop and a regular while loop is that a do while loop will always execute the block of code at least once, regardless of whether the condition is true or false.
The Secret Technique
Now that we have a basic understanding of the do while loop, let’s dive into the secret technique that will revolutionize your coding. The secret technique involves using the do while loop in combination with a flag variable to create a more efficient and flexible looping structure.
Instead of having the condition directly in the do while loop, you can use a flag variable to control the loop. This allows you to have more control over when the loop should stop, and gives you the flexibility to change the condition within the loop based on certain criteria. Let’s take a look at an example to illustrate this technique:
$flag = true;
do {
// Your code here
if (/* Some condition */) {
$flag = false;
}
} while ($flag);
In this example, we initialize a flag variable to true before entering the do while loop. Within the loop, we have a conditional statement that can change the value of the flag variable based on certain criteria. This allows us to control when the loop should stop, and gives us the flexibility to change the condition within the loop without having to modify the initial condition of the do while loop.
Benefits of the Secret Technique
Now that you understand the secret technique, let’s take a look at some of the benefits it offers:
- Flexibility: Using a flag variable allows you to change the loop condition dynamically within the loop based on certain criteria, giving you greater flexibility in controlling the loop.
- Efficiency: The secret technique helps streamline your code by reducing the need for repetitive condition checks within the loop, making your code more efficient and easier to maintain.
- Readability: By using a flag variable to control the loop, you can improve the readability of your code by separating the loop condition from the do while loop, making it easier for other developers to understand your code.
Examples
Let’s take a look at some real-world examples to see how the secret technique can be applied in practice:
Example 1: Processing User Input
Suppose you are building a web application that processes user input in a form. You can use the secret technique to validate the user input and continue processing only if the input is valid. Here’s a simplified example:
$inputValid = false;
do {
$input = /* Get user input */;
if (/* Validate input */) {
$inputValid = true;
// Process input
} else {
// Display error message
}
} while (!$inputValid);
Example 2: Batch Processing Data
Imagine you need to batch process a large dataset, but you want to limit the processing to a certain number of items at a time. You can use the secret technique to control the batch processing and stop when a certain condition is met. Here’s a simplified example:
$dataProcessed = 0;
$batchSize = 100;
do {
$data = /* Get batch of data */;
if (/* Check data condition */) {
// Process data
$dataProcessed += count($data);
} else {
// Stop processing
}
} while ($dataProcessed < $batchSize);
Conclusion
The secret PHP do while loop technique offers a powerful and flexible approach to controlling loops in your code. By using a flag variable to control the loop, you can make your code more efficient, flexible, and easier to read. Whether you are processing user input, batch processing data, or tackling any other coding challenge, the secret technique can revolutionize your coding and take your programming skills to the next level.
FAQs
What is the difference between a while loop and a do while loop?
A while loop will check the condition before executing the block of code, while a do while loop will always execute the block of code at least once before checking the condition.
Can I use the secret technique with other programming languages?
While this article focuses on PHP, the secret technique can be applied to other programming languages that support do while loops, such as C, C++, Java, and more.
Where can I learn more about advanced PHP programming techniques?
For more advanced PHP programming techniques, consider exploring resources such as online tutorials, forums, and books dedicated to PHP programming.