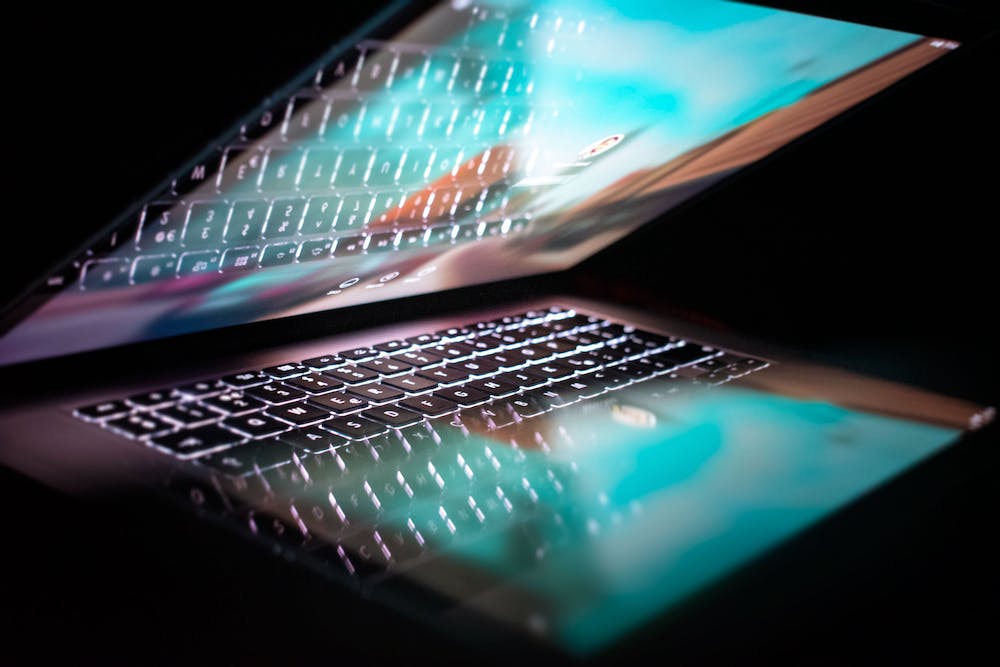
Python is a versatile and powerful programming language that is used for a wide range of applications, including web development, data analysis, artificial intelligence, and more. One of the most common uses of Python is for creating calculators, and IT‘s truly mind-blowing how easy it is to do with this language.
In this article, we will walk you through the process of creating an advanced calculator using Python. You’ll learn the essential code and concepts needed to build a calculator with Python, and by the end, you’ll be amazed at how simple and powerful this code is.
The Basics of Python
Before we dive into creating a calculator, let’s go over some basic Python concepts. Python is known for its simplicity and readability, which makes it a great language for beginners. It uses indentation to define code blocks, which makes it easy to read and write.
Python also has a large standard library that includes modules and functions for various tasks, such as math operations, string manipulation, file handling, and more. This means you don’t have to write everything from scratch, and you can take advantage of the built-in functionalities to make your code more efficient.
Creating a Simple Calculator
Now that we have a basic understanding of Python, let’s start by creating a simple calculator. We’ll begin with the four basic operations: addition, subtraction, multiplication, and division.
“`Python
# Simple Calculator
def add(x, y):
return x + y
def subtract(x, y):
return x – y
def multiply(x, y):
return x * y
def divide(x, y):
return x / y
“`
With this code, we have defined four functions for each of the basic operations. Now, let’s test our calculator with some simple calculations:
“`Python
print(add(5, 3)) # Output: 8
print(subtract(10, 4)) # Output: 6
print(multiply(7, 2)) # Output: 14
print(divide(15, 3)) # Output: 5.0
“`
As you can see, with just a few lines of code, we have created a simple calculator that can perform basic arithmetic operations. This is just the beginning, and we can expand on this to create a more advanced calculator with additional features.
Adding More Functionality to the Calculator
Now that we have a simple calculator, let’s enhance it with more functionality. We can add features such as exponentiation, square root, and handling invalid inputs.
“`Python
# Enhanced Calculator
import math
def power(x, y):
return x ** y
def square_root(x):
return math.sqrt(x)
def calculator():
while True:
try:
num1 = float(input(“Enter the first number: “))
op = input(“Enter the operation (+, -, *, /, ^, sqrt): “)
if op in (“+”, “-“, “*”, “/”):
num2 = float(input(“Enter the second number: “))
if op == “+”:
print(f”Result: {add(num1, num2)}”)
elif op == “-“:
print(f”Result: {subtract(num1, num2)}”)
elif op == “*”:
print(f”Result: {multiply(num1, num2)}”)
elif op == “/”:
print(f”Result: {divide(num1, num2)}”)
elif op == “^”:
num2 = float(input(“Enter the power: “))
print(f”Result: {power(num1, num2)}”)
elif op == “sqrt”:
print(f”Result: {square_root(num1)}”)
else:
print(“Invalid operation”)
choice = input(“Do you want to continue? (yes/no): “)
if choice.lower() != “yes”:
break
except ValueError:
print(“Invalid input. Please enter a valid number.”)
except ZeroDivisionError:
print(“Division by zero is not allowed.”)
“`
With this enhanced code, we have added two new functions for exponentiation and square root. We also created a loop to continuously perform calculations and handle invalid inputs gracefully.
Let’s test the enhanced calculator:
“`Python
calculator()
“`
Now, you have a fully functional calculator with advanced features, and it’s all built with Python. What’s even more amazing is that this code is just a fraction of what Python can do. There are endless possibilities for creating powerful and sophisticated applications using Python.
Conclusion
Creating an advanced calculator with Python is truly mind-blowing. With just a few lines of code, you can build a calculator that can perform basic arithmetic operations, as well as more advanced functions like exponentiation and square root. Python’s simplicity and versatility make it an excellent choice for building all kinds of applications, and this calculator is just one example of what you can achieve with this language.
Whether you’re a beginner or an experienced programmer, learning Python is a valuable skill that can open up a world of possibilities. If you’re interested in diving deeper into Python and creating even more impressive applications, consider enrolling in a Python programming course to enhance your skills and knowledge.
FAQs
Q: Can I use this code for a real-world application?
A: Yes, you can use this code as a starting point for building a fully functional calculator for a real-world application. You may need to add additional features and enhance the user interface depending on your specific requirements.
Q: Is Python the best language for creating a calculator?
A: Python is a great language for creating a calculator due to its simplicity, readability, and extensive library. However, the best language for a specific application depends on various factors, including the project requirements, your familiarity with the language, and the target platform.
Q: How can I further enhance this calculator?
A: There are numerous ways to enhance this calculator, such as adding memory functions, handling more complex mathematical operations, and improving the user interface. You can also explore integrating the calculator into a web application using Python’s web frameworks, such as Flask or Django.
Q: Are there any online resources for learning Python?
A: Yes, there are many online resources for learning Python, including interactive tutorials, video courses, and documentation. You can also join online communities and forums to seek help, share knowledge, and connect with other Python enthusiasts.