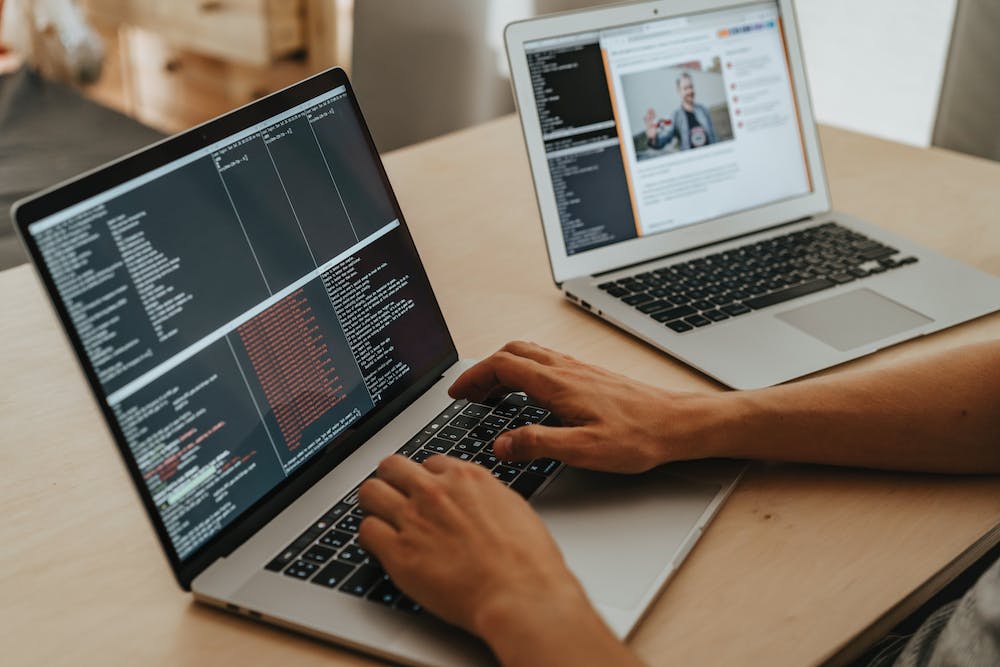
Python is a powerful and versatile programming language that is used in a wide range of applications, from web development to data analysis. One of the most fascinating aspects of Python is its ability to solve complex mathematical problems with just a few lines of code. In this article, we will take a deep dive into the world of Armstrong numbers and learn how to write a mind-blowing Python code to identify them. You won’t believe how IT works!
What are Armstrong Numbers?
An Armstrong number (also known as a narcissistic number, plenary number, or pluperfect number) is a number that is equal to the sum of its own digits raised to the power of the number of digits. For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
Armstrong numbers are named after Michael F. Armstrong, who first introduced them to the world in 1888. They have fascinated mathematicians and programmers for years due to their unique properties and the challenge they pose in terms of finding and identifying them.
Python Code for Armstrong Numbers
Now, let’s dive into the Python code for identifying Armstrong numbers. The beauty of Python lies in its simplicity and readability. Let’s start by writing a Python function that takes a number as input and returns true if it is an Armstrong number, and false otherwise:
“`python
def is_armstrong_number(num):
num_str = str(num)
num_digits = len(num_str)
armstrong_sum = sum(int(digit)**num_digits for digit in num_str)
return armstrong_sum == num
“`
Let’s break down the code:
- We first convert the input number into a string so that we can easily iterate through its digits.
- We then calculate the number of digits in the input number using the len() function.
- Next, we use a generator expression to iterate through each digit of the number, raise it to the power of the number of digits, and sum the results.
- Finally, we compare the sum to the original input number and return true if they are equal, and false otherwise.
Now that we have the code for identifying Armstrong numbers, let’s test it with a few examples:
“`python
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(370)) # Output: True
print(is_armstrong_number(9474)) # Output: True
print(is_armstrong_number(123)) # Output: False
“`
As you can see, the code correctly identifies 153, 370, and 9474 as Armstrong numbers, and 123 as a non-Armstrong number.
How It Works
Now, let’s take a closer look at how the Python code for Armstrong numbers works. The key insight here is the use of the generator expression to calculate the sum of the digits raised to the power of the number of digits.
By converting the input number to a string, we can easily iterate through its digits using a simple for loop. We then use the int() function to convert each digit back to an integer, raise it to the power of the number of digits, and sum the results using the sum() function.
In the end, we compare the result to the original input number to determine if it is an Armstrong number. This elegant and concise code showcases the power of Python in solving mathematical problems efficiently and effectively.
Conclusion
In conclusion, Python is an incredibly powerful tool for solving mathematical problems, and the code for identifying Armstrong numbers is a testament to its simplicity and elegance. With just a few lines of code, we can efficiently determine whether a number is an Armstrong number or not. The beauty of Python lies in its readability and ease of use, making it an ideal choice for tackling a wide range of mathematical challenges.
FAQs
What are some real-world applications of Armstrong numbers?
Armstrong numbers have applications in various fields, including cryptography, data validation, and algorithm design. For example, they can be used in data validation to check the integrity of numerical data, and in cryptography to generate secure keys and passwords.
Can the Python code for Armstrong numbers be optimized for performance?
Yes, the Python code for Armstrong numbers can be optimized for performance by utilizing techniques such as memoization and caching to store previously calculated results. Additionally, implementing the code in a more concise and efficient manner can further enhance its performance.
Are there any libraries or modules in Python specifically designed for working with Armstrong numbers?
While there are no specific libraries or modules dedicated to Armstrong numbers, Python offers a wide range of built-in functions and modules for working with mathematical calculations, such as the math module and the itertools module. These can be utilized to optimize and streamline the code for Armstrong numbers.
How can I further enhance my understanding of Python and mathematical programming?
To further enhance your understanding of Python and mathematical programming, consider exploring online resources, tutorials, and courses focused on Python programming, algorithms, and mathematical problem-solving. Additionally, practice and experimentation with different programming challenges can help strengthen your skills and knowledge in this area.