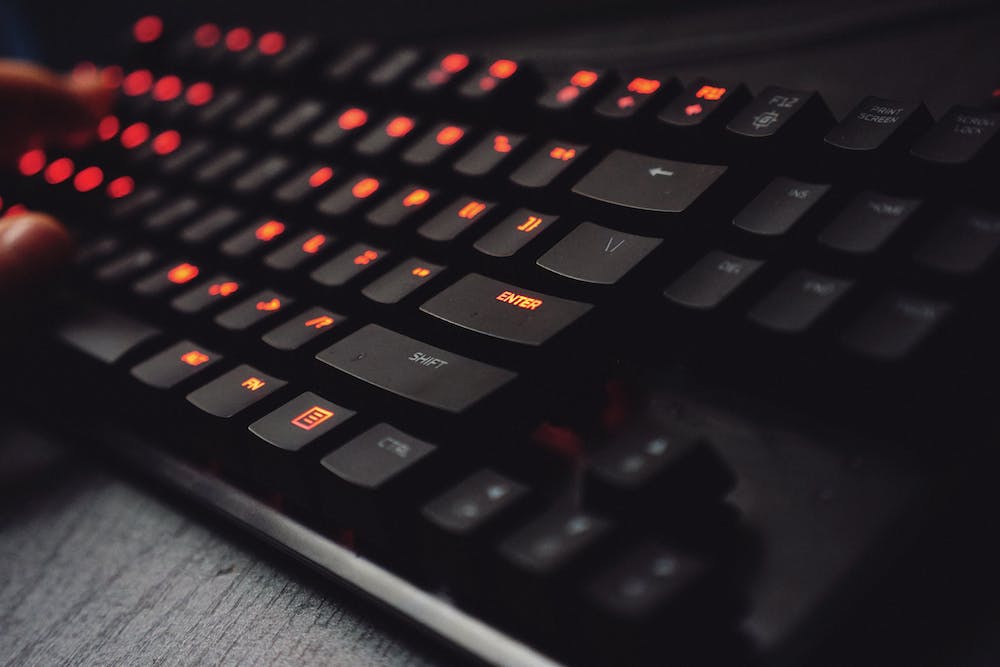
PHP, which stands for Hypertext Preprocessor, is a powerful server-side scripting language that is widely used for web development. IT is a versatile language that can be used to create dynamic and interactive websites. Whether you are a beginner or an experienced developer, there are always new and exciting tricks to learn in PHP. In this article, we will explore 5 mind-blowing PHP tricks that you need to know ASAP!
Trick #1: Utilize Object-Oriented Programming (OOP)
One of the most powerful features of PHP is its support for Object-Oriented Programming (OOP). With OOP, you can create reusable and modular code that is easier to maintain and extend. By utilizing classes and objects, you can organize your code more efficiently and improve its readability. Here’s an example of how to create a simple class in PHP:
class Car {
public $color;
public $model;
public function __construct($color, $model) {
$this->color = $color;
$this->model = $model;
}
public function drive() {
echo "Driving a " . $this->color . " " . $this->model;
}
}
$myCar = new Car("red", "Toyota");
$myCar->drive();
In this example, we have created a Car class with properties for color and model, as well as a method for driving the car. We then create a new instance of the Car class and call the drive method. By using OOP in PHP, you can write more organized and maintainable code, and unleash the full power of the language.
Trick #2: Take Advantage of Namespaces
Namespaces are a great way to organize your PHP code and avoid naming conflicts. They allow you to group related classes, functions, and constants together, and provide a way to encapsulate your code. Here’s an example of how to use namespaces in PHP:
namespace MyNamespace;
class MyClass {
// class implementation
}
function myFunction() {
// function implementation
}
const MY_CONSTANT = "value";
In this example, we have created a namespace called MyNamespace, and placed a class, a function, and a constant inside it. This allows us to avoid name collisions with other code, and provides a clear and logical structure to our codebase. By using namespaces in PHP, you can improve the organization and reusability of your code, and make it easier to collaborate with other developers.
Trick #3: Master Error Handling
Error handling is a crucial aspect of PHP development, and mastering it can save you a lot of headaches down the road. PHP provides several mechanisms for handling errors, such as try/catch blocks for exceptions, and the error_reporting and error_log functions for handling non-fatal errors. Here’s an example of how to use try/catch blocks in PHP:
try {
// code that may throw an exception
} catch (Exception $e) {
// handle the exception
}
By mastering error handling in PHP, you can ensure that your code is robust and reliable, and provide a better experience for users. Proper error handling can also make it easier to troubleshoot and debug your code, and improve the overall quality of your applications.
Trick #4: Optimize Your Database Queries
Database interaction is a common task in PHP development, and optimizing your database queries can have a significant impact on the performance of your applications. One way to optimize database queries in PHP is to use prepared statements, which can help prevent SQL injection attacks and improve the efficiency of your queries. Here’s an example of how to use prepared statements in PHP using PDO:
$pdo = new PDO("mysql:host=localhost;dbname=mydb", "username", "password");
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username");
$stmt->bindParam(':username', $username);
$stmt->execute();
$result = $stmt->fetch(PDO::FETCH_ASSOC);
By optimizing your database queries in PHP, you can improve the performance and security of your applications, and ensure that they can scale to meet the needs of your users. Efficient database interactions are essential for creating fast and responsive web applications, and mastering this trick can take your PHP development skills to the next level.
Trick #5: Harness the Power of Regular Expressions
Regular expressions are a powerful tool for manipulating and validating strings in PHP. They provide a flexible and efficient way to search for and manipulate text, and can be used for tasks such as validating input, parsing data, and performing search and replace operations. Here’s an example of how to use regular expressions in PHP:
$text = "The quick brown fox jumps over the lazy dog";
if (preg_match("/fox/", $text)) {
echo "The text contains the word 'fox'";
}
In this example, we use the preg_match function to search for the word ‘fox’ in a given text. Regular expressions provide a powerful and flexible way to work with strings in PHP, and mastering them can significantly expand your programming capabilities.
Conclusion
PHP is a versatile and powerful language that offers a wide range of features and capabilities for web development. By mastering these 5 mind-blowing PHP tricks, you can take your PHP skills to the next level and create more efficient, robust, and maintainable code. Whether you are a beginner or an experienced developer, there is always something new to learn in PHP, and these tricks can help you unlock the full potential of the language. By harnessing the power of OOP, namespaces, error handling, database optimization, and regular expressions, you can become a more proficient and effective PHP developer.
FAQs
1. How can I learn more about PHP?
There are many resources available for learning PHP, including online tutorials, books, and courses. You can also join online communities and forums to connect with other PHP developers and learn from their experiences.
2. Are these PHP tricks suitable for beginners?
Yes, these PHP tricks are suitable for developers of all skill levels. Whether you are a beginner or an experienced developer, mastering these tricks can help you improve your PHP skills and create more efficient and maintainable code.
3. How can I practice these PHP tricks?
You can practice these PHP tricks by creating small projects and experimenting with different features of the language. By applying these tricks in real-world scenarios, you can gain a deeper understanding of their capabilities and limitations.
4. Where can I find more examples of these PHP tricks in action?
You can find more examples of these PHP tricks in action by searching online for code snippets, tutorials, and open-source projects. You can also experiment with these tricks in your own projects and seek feedback from other developers to help you improve.