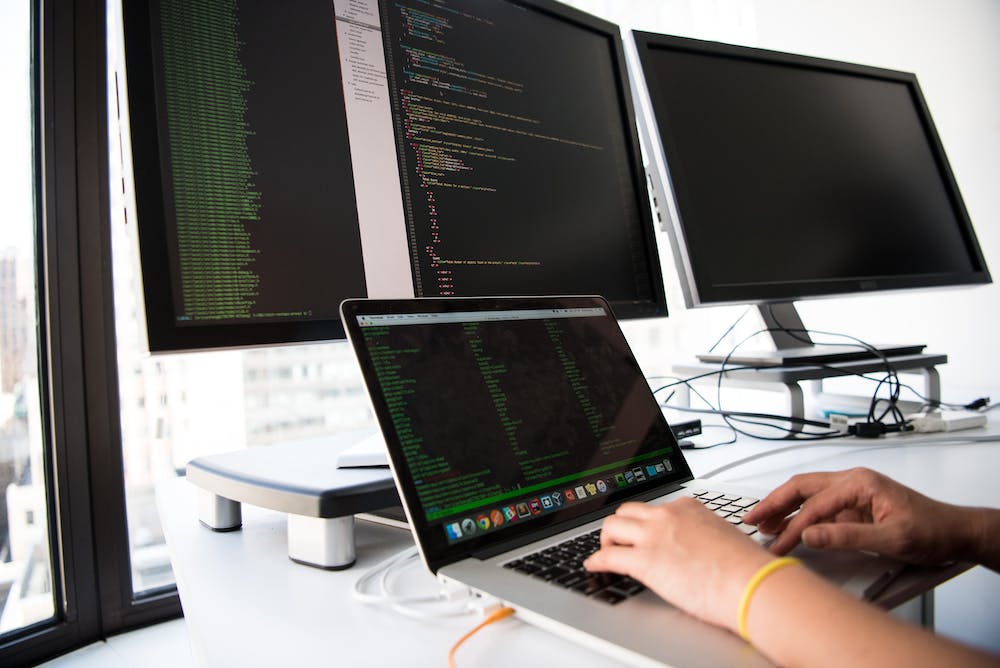
Are you a PHP developer, struggling with converting strings to dates? Look no further! In this article, we will uncover the magic of converting strings to dates easily with PHP. You won’t believe how simple IT is!
Understanding the Problem
As a PHP developer, you may have encountered the challenge of working with date and time data. Oftentimes, you receive date and time information in the form of strings, which need to be converted to a usable format for your application. This process can be complex and time-consuming, especially when dealing with various date formats and timezones. Fortunately, PHP provides powerful tools for handling date and time data, making the conversion process simple and efficient.
The Magic of strtotime()
One of the most powerful functions for converting strings to dates in PHP is strtotime(). This function allows you to parse about any English textual datetime description into a Unix timestamp. This means that you can easily convert a date string in various formats to a Unix timestamp, which can then be formatted into any desired date format using PHP’s date() function.
Let’s take a look at a simple example:
$dateString = "2022-03-15";
$dateTimestamp = strtotime($dateString);
$formattedDate = date('Y-m-d', $dateTimestamp);
echo $formattedDate;
In this example, we have a date string in the format “2022-03-15”. We use the strtotime() function to convert this string to a Unix timestamp, and then format the timestamp into the desired “Y-m-d” format using the date() function. The result is the formatted date “2022-03-15”. It’s as simple as that!
Dealing with Timezones
When working with date and time data, it’s important to consider the timezone in which the data is captured and processed. PHP provides the DateTime class, which makes it easy to work with dates and times in different timezones. This class allows you to create datetime objects from date strings, and then manipulate and format them according to different timezones.
Here’s an example of how to convert a date string to a datetime object and format it according to a specific timezone:
$dateString = "2022-03-15 12:00:00";
$dateTime = new DateTime($dateString, new DateTimeZone('UTC'));
$dateTime->setTimezone(new DateTimeZone('America/New_York'));
$formattedDate = $dateTime->format('Y-m-d H:i:s');
echo $formattedDate;
In this example, we have a date and time string in the format “2022-03-15 12:00:00”. We create a new DateTime object from the string, set the timezone to UTC, and then convert it to the “America/New_York” timezone. Finally, we format the datetime object into the desired format and echo the result. With the DateTime class, working with different timezones becomes a breeze!
Handling Different Date Formats
Another common challenge when working with date strings is dealing with different date formats. Fortunately, PHP provides the DateTime::createFromFormat() method, which allows you to create a datetime object from a string in a specific format. This method is particularly useful when working with date strings in non-standard formats.
Let’s take a look at an example of using DateTime::createFromFormat() to convert a date string in a custom format to a datetime object:
$dateString = "15/03/2022";
$dateTime = DateTime::createFromFormat('d/m/Y', $dateString);
$formattedDate = $dateTime->format('Y-m-d');
echo $formattedDate;
In this example, we have a date string in the format “15/03/2022”. We use DateTime::createFromFormat() to create a datetime object from the string, specifying the input format as ‘d/m/Y’. We then format the datetime object into the desired “Y-m-d” format and echo the result. With DateTime::createFromFormat(), you can easily handle date strings in custom formats.
Conclusion
Converting strings to dates in PHP doesn’t have to be a daunting task. With the powerful tools and functions provided by PHP, such as strtotime(), the DateTime class, and DateTime::createFromFormat(), you can easily and efficiently handle date and time data in your applications. Whether you’re working with different date formats, timezones, or non-standard datetime strings, PHP has you covered. By understanding and leveraging these tools, you can unlock the magic of converting strings to dates with ease!
FAQs
Q: Can I use strtotime() to parse any date and time string?
A: strtotime() is a powerful function for parsing English textual datetime descriptions into Unix timestamps. However, it may not work as expected with certain date and time formats or languages. It’s always a good practice to test the function with different formats to ensure accurate parsing.
Q: How can I handle date strings in non-standard formats?
A: You can use the DateTime::createFromFormat() method to create datetime objects from date strings in custom formats. By specifying the input format, you can easily convert non-standard date strings to datetime objects and then format them according to your needs.
Q: Is it necessary to consider timezones when working with date and time data?
A: Yes, it’s important to consider the timezone in which date and time data is captured and processed. PHP provides the DateTime class, which makes it easy to work with dates and times in different timezones. By creating datetime objects and setting timezones accordingly, you can ensure accurate and consistent handling of date and time data.