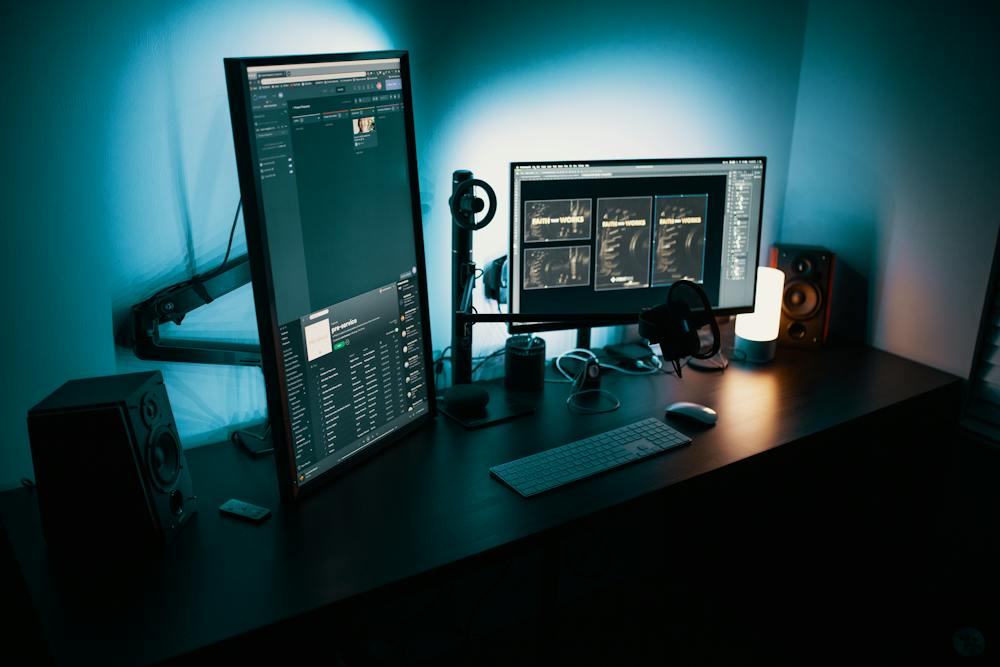
Python is one of the most popular programming languages in the world. IT is versatile, easy to learn, and has a wide range of applications. Whether you’re a beginner or an experienced programmer, mastering certain Python programs can significantly boost your skills and make you a more valuable asset in the tech industry.
1. Web Scraping with BeautifulSoup
Web scraping is the process of extracting data from websites. BeautifulSoup is a Python library that makes it easy to scrape web pages and extract the information you need. Mastering web scraping with BeautifulSoup can open up a world of possibilities for data collection and analysis.
Example:
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extracting a specific element
element = soup.find('h1')
print(element.text)
2. Data Analysis with Pandas
Pandas is a powerful data analysis library for Python. It provides data structures and functions for manipulating and analyzing large datasets. Mastering Pandas can help you clean, process, and analyze data more efficiently, making you a valuable asset for any data-driven organization.
Example:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 23, 25, 32]}
df = pd.DataFrame(data)
# Printing the DataFrame
print(df)
3. GUI Development with Tkinter
Tkinter is the standard GUI toolkit for Python. Mastering Tkinter can enable you to create user-friendly graphical interfaces for your Python programs. Whether you’re building simple applications or complex software, knowing how to use Tkinter can set you apart as a versatile programmer.
Example:
import tkinter as tk
root = tk.Tk()
root.title("Hello, Tkinter!")
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
root.mainloop()
4. Web Development with Flask
Flask is a lightweight and flexible web framework for Python. Mastering Flask can empower you to build web applications and APIs with ease. As web development continues to be in high demand, adding Flask to your skill set can be a game-changer for your career.
Example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
5. Machine Learning with scikit-learn
Scikit-learn is a popular machine learning library for Python. Mastering scikit-learn can enable you to build and deploy machine learning models for a wide range of applications, from predictive analytics to natural language processing.
Example:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load the iris dataset
iris = datasets.load_iris()
X, y = iris.data, iris.target
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Build and train the KNN model
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = knn.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
Conclusion
Mastering these Python programs can significantly boost your skills as a programmer. Whether you’re interested in data analysis, web development, or machine learning, these programs can open up new opportunities for you in the tech industry. Remember to practice and apply these programs in real-world projects to solidify your expertise.
FAQs
1. How can I learn these Python programs?
You can learn these Python programs through online tutorials, documentation, and practice. There are also many books and courses available that can help you master these programs.
2. Are these programs suitable for beginners?
Yes, these programs are suitable for beginners as well as experienced programmers. With dedication and practice, anyone can master these Python programs.
3. Are there any other Python programs I should learn?
While these programs are essential for any programmer, there are many other Python libraries and frameworks worth exploring, depending on your interests and career goals.
4. Can mastering these programs help me get a job?
Absolutely! Mastering these Python programs can make you a more valuable asset as a programmer, increasing your chances of landing a job in the tech industry.