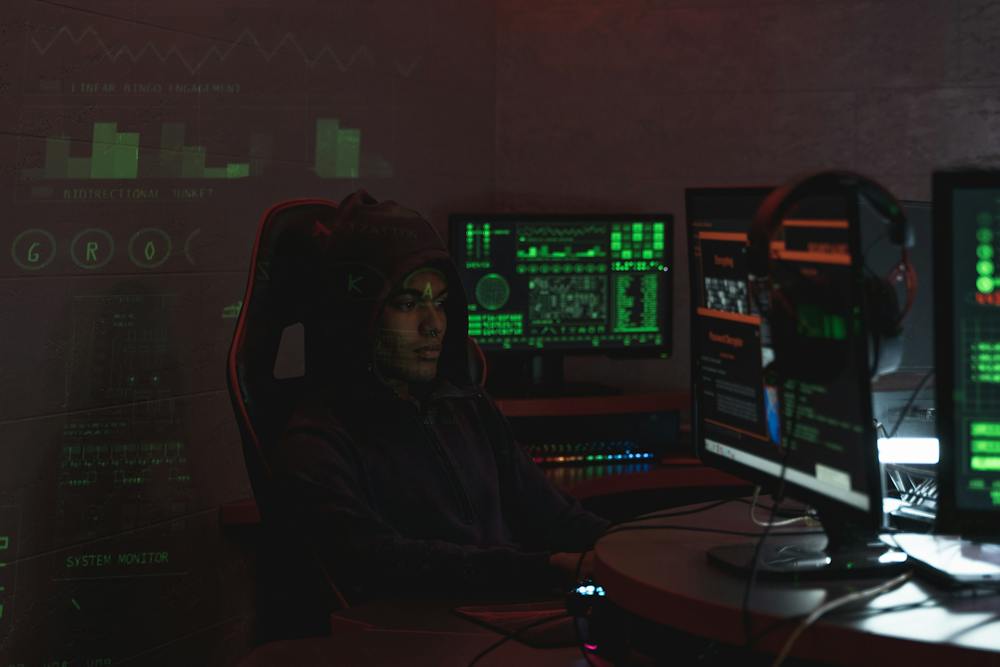
By: Your Name
Day 1: Getting Started with Python
Welcome to day 1 of our Python programming journey! Python is a high-level programming language known for its simplicity and easy readability. IT is widely used in various fields such as web development, data analysis, artificial intelligence, and more. Today, we will focus on setting up your development environment and getting familiar with basic Python syntax.
To get started, you will need to install Python on your computer. You can download the latest version of Python from the official Website (www.python.org). Once installed, you can open a command prompt or terminal and type ‘python’ to enter the Python interactive mode.
Let’s start by writing your first Python program, the traditional ‘Hello, World!’ program. Open a text editor and type the following code:
print('Hello, World!')
Save the file with a .py extension, for example, hello_world.py. Then, open a command prompt or terminal, navigate to the directory where the file is saved, and run the program by typing ‘python hello_world.py’. You should see the output ‘Hello, World!’ printed on the screen.
Congratulations! You have written and executed your first Python program. This is just the beginning of your Python journey.
Day 2: Variables and Data Types
On day 2, we will delve deeper into Python’s variables and data types. In Python, you can create variables to store data values. Unlike other programming languages, Python does not require you to declare the data type of a variable. Python automatically identifies the data type based on the value assigned to the variable.
Here are some commonly used data types in Python:
- Integer (int): represents whole numbers
- Float (float): represents floating-point numbers
- String (str): represents text
- List: represents a collection of items
- Dictionary: represents a collection of key-value pairs
- Boolean (bool): represents True or False
Let’s see some examples of using these data types in Python:
age = 25 # integer
temperature = 98.6 # float
name = 'John Smith' # string
numbers = [1, 2, 3, 4, 5] # list
person = {'name': 'Alice', 'age': 30} # dictionary
is_student = True # boolean
By understanding and mastering variables and data types, you will be well-equipped to handle various types of data in your Python programs.
Day 3: Control Flow and Loops
Day 3 is all about control flow and loops in Python. Control flow statements allow you to make decisions and perform different actions based on certain conditions. Loops, on the other hand, enable you to execute a block of code repeatedly.
Here are the main control flow statements and loop types in Python:
- If…else statement: allows you to execute different code based on the result of a condition
- While loop: executes a block of code as long as the specified condition is true
- For loop: iterates over a sequence of elements (e.g., list, dictionary) and executes a block of code for each element
Let’s see an example of using these control flow and loop types in Python:
age = 18
if age >= 18:
print('You are an adult')
else:
print('You are a minor')
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
Understanding control flow and loops is essential for building logic and iterating through data in your Python programs.
Day 4: Functions and Modules
Functions and modules are crucial for organizing and reusing code in Python. A function is a block of reusable code that performs a specific task, while a module is a file containing Python code that can be used in other Python programs.
To create a function in Python, you can use the ‘def’ keyword followed by the function name and parameters. Here’s an example of a simple function:
def greet(name):
print('Hello, ' + name + '!')
After defining a function, you can call it by passing the required arguments, as shown below:
greet('John')
Furthermore, Python comes with a rich library of standard modules that you can import and use in your programs. For instance, the ‘math’ module provides various mathematical functions and constants. To use a module in your program, you can use the ‘import’ keyword, followed by the module name.
Mastering functions and modules will significantly enhance the modularity and maintainability of your Python code.
Day 5: Working with Files and Exception Handling
On day 5, we will focus on file handling and exception handling in Python. Python provides robust mechanisms for reading from and writing to files, as well as handling potential errors and exceptions that may occur during program execution.
To open a file in Python, you can use the built-in ‘open()’ function, which takes the file name and mode as parameters. There are several modes for opening a file, such as ‘r’ for reading, ‘w’ for writing, and ‘a’ for appending.
Here’s an example of opening a file and writing data to it:
file = open('example.txt', 'w')
file.write('Hello, Python!')
file.close()
Additionally, Python allows you to handle exceptions using ‘try…except’ blocks. This enables you to gracefully handle errors and prevent program crashes.
By understanding file handling and exception handling, you can build more robust and error-tolerant Python applications.
Day 6: Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a programming paradigm that allows you to model real-world entities as objects with attributes and behaviors. Python is an object-oriented language, and it supports OOP principles such as encapsulation, inheritance, and polymorphism.
To create a class in Python, you can use the ‘class’ keyword followed by the class name. Within a class, you can define attributes (variables) and methods (functions) that operate on the attributes.
Here’s an example of defining a simple class in Python:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
print('This is a ' + self.make + ' ' + self.model)
After defining a class, you can create objects (instances) of that class and access their attributes and methods.
car1 = Car('Toyota', 'Camry')
car1.display_info()
By mastering object-oriented programming, you can design and implement complex, scalable, and maintainable Python applications.
Day 7: Final Projects and Practice
Congratulations on reaching day 7 of our Python programming journey! Today, we encourage you to embark on final projects and practice exercises to reinforce your learning and apply your newfound Python skills.
You can choose to work on a small project that interests you, such as a simple web application, a data analysis tool, or a game. Alternatively, you can explore online coding platforms and practice solving coding challenges and exercises.
Remember, continuous practice and building real-world projects are essential for solidifying your understanding and proficiency in Python programming. Stay curious, stay motivated, and keep coding!
Conclusion
Congratulations on completing the 7-day Python programming course! Throughout this journey, you have learned the fundamental concepts of Python, including variables, data types, control flow, functions, modules, file handling, exception handling, object-oriented programming, and more. As you continue your programming endeavors, we encourage you to explore advanced topics, contribute to open-source projects, and never stop learning. Python is a powerful and versatile language with endless possibilities, and we hope this guide has ignited your passion for coding with Python.
FAQs
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used for web development, data analysis, artificial intelligence, and more.
2. Is Python suitable for beginners?
Yes, Python is an excellent language for beginners due to its easy syntax and extensive community support. It is also used by professionals in various industries, making it a valuable skill to learn.
3. How can I practice Python programming?
You can practice Python programming by working on projects, solving coding challenges, participating in coding competitions, and contributing to open-source projects. Online coding platforms and communities are also great resources for practice and learning.