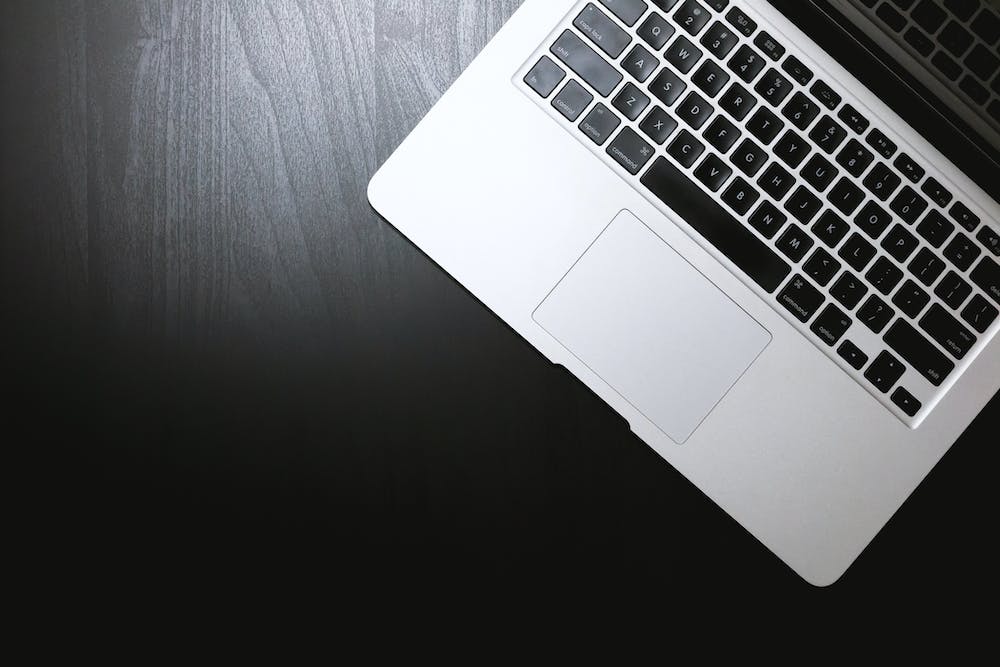
Python is a high-level, general-purpose programming language that is widely used for web development, data analysis, artificial intelligence, scientific computing, and many other applications. IT is known for its simplicity and readability, making IT a great language for beginners to learn. In this ultimate beginner’s guide, we will take you through the basics of Python programming from scratch, helping you to build a strong foundation in this versatile language.
Getting Started with Python
If you’re new to programming, Python is a great language to start with. IT has a simple and easy-to-understand syntax, which makes IT beginner-friendly. To get started with Python, you will need to install the Python interpreter on your computer. You can download the latest version of Python from the official Website and follow the installation instructions for your operating system.
Once you have Python installed, you can start writing your first program. The traditional “Hello, World!” program is often used as the first program to write in any programming language. In Python, IT looks like this:
print("Hello, World!")
Save this code in a file with a .py extension, such as hello.py, and run IT using the Python interpreter. You should see the output “Hello, World!” printed to the console. Congratulations, you’ve just written your first Python program!
Variables and Data Types
Like any programming language, Python provides a way to store and manipulate data. Variables are used to store values, and data types define the kind of data that can be stored in a variable. Some of the basic data types in Python include:
- Integer (int): for whole numbers
- Float (float): for decimal numbers
- String (str): for text
- Boolean (bool): for true or false values
Here’s an example of how you can declare and use variables in Python:
# Declare variables
num1 = 10
num2 = 3.14
name = "Python"
is_learning = True
# Print variables
print(num1)
print(num2)
print(name)
print(is_learning)
When you run this code, you should see the values of the variables printed to the console. This is just a simple example, and Python supports many other data types and operations for working with data.
Control Flow and Loops
Control flow statements allow you to control the flow of your program based on conditions. If statements are used to execute a block of code if a condition is true, while loops are used to execute a block of code repeatedly while a condition is true, and for loops are used to iterate over a sequence of elements.
Here’s an example of how you can use if and while statements in Python:
# if statement
age = 18
if age >= 18:
print("You are an adult")
# while loop
count = 0
while count < 5:
print(count)
count += 1
When you run this code, you should see the output “You are an adult” printed to the console, followed by the numbers 0 to 4 printed on separate lines. These are just a few examples of control flow statements in Python, and there are many more ways to control the flow of your program.
Functions and Modules
Functions are used to organize and reuse code, while modules are used to organize and reuse functions and other elements of your program. You can define your own functions and modules in Python, and use them to build larger, more complex programs.
Here’s an example of how you can define and use a function in Python:
# Define a function
def greet(name):
print(f"Hello, {name}!")
# Call the function
greet("Python")
When you run this code, you should see the output “Hello, Python!” printed to the console. This is a simple example of a function, and Python supports many more advanced features for defining and using functions and modules.
Conclusion
Congratulations on completing this ultimate beginner’s guide to learning Python programming from scratch! We’ve covered the basics of Python, including how to get started, working with variables and data types, control flow and loops, and functions and modules. With these foundational skills, you’re well on your way to becoming a proficient Python programmer. Keep practicing and building on what you’ve learned, and you’ll soon be able to tackle more advanced Python topics with confidence.
FAQs
1. What can I do with Python?
Python is a versatile language that can be used for web development, data analysis, artificial intelligence, scientific computing, and many other applications. IT is also commonly used in scripting and automation tasks.
2. Is Python easy to learn for beginners?
Yes, Python is considered to be a beginner-friendly language due to its simple and readable syntax. IT is a great language for beginners to learn as their first programming language.
3. How can I practice and improve my Python skills?
There are many resources available for practicing and improving your Python skills, including online tutorials, coding challenges, and projects. You can also join Python communities and forums to connect with other developers and learn from their experiences.
4. Are there any advanced topics I can learn after mastering the basics of Python?
Once you have a strong foundation in Python, you can explore more advanced topics such as object-oriented programming, database integration, web development frameworks, and data science libraries. There are endless opportunities to continue learning and growing as a Python programmer.