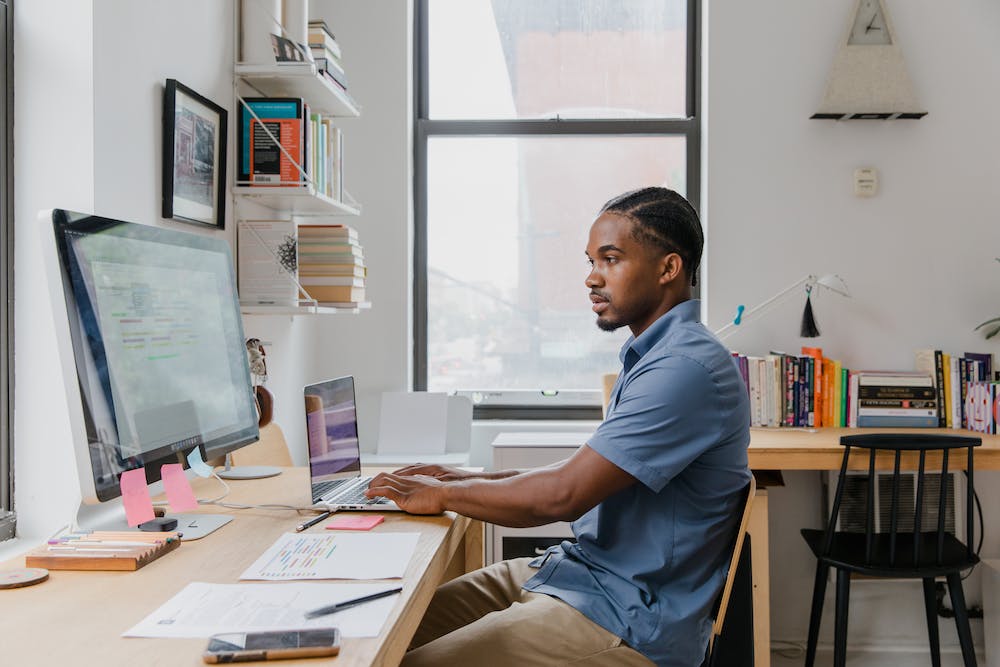
Decision trees are a powerful tool for both classification and regression tasks in machine learning. In this guide, we will walk you through mastering decision tree code in Python with easy steps. By the end of this article, you will have a solid understanding of decision trees and be able to implement them in your own projects.
Step 1: Installing Python and necessary libraries
The first step to mastering decision tree code in Python is to have Python installed on your computer. You can download and install Python from the official Website. Once Python is installed, you will need to install the necessary libraries for working with decision trees. The main libraries you will need are numpy
, pandas
, and scikit-learn
.
Step 2: Understanding decision trees
Before diving into the code, IT‘s important to have a good understanding of decision trees. Decision trees are a type of supervised learning algorithm that is used for both classification and regression tasks. They work by recursively splitting the data into subsets based on the most significant attribute at each node. This process continues until the data is completely classified or the maximum depth of the tree is reached.
Step 3: Importing the necessary libraries
Once Python and the necessary libraries are installed, you can start coding with decision trees. Begin by importing the required libraries into your Python script:
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
Step 4: Loading the dataset
Next, you will need a dataset to work with. For this example, we will use the famous Iris dataset, which can be loaded using the load_iris()
function from the sklearn.datasets
module:
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data
y = iris.target
Step 5: Preprocessing the data
Before training the decision tree, it’s important to preprocess the data by splitting it into training and testing sets:
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 6: Training the decision tree
Now you are ready to train the decision tree using the training data:
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
Step 7: Making predictions
With the decision tree trained, you can now make predictions on the testing data:
y_pred = clf.predict(X_test)
Step 8: Evaluating the model
Finally, you can evaluate the performance of the decision tree model by comparing the predicted labels to the actual labels in the testing set:
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy of the decision tree model:", accuracy)
Conclusion
Congratulations! You have successfully mastered decision tree code in Python. Decision trees are a versatile and powerful tool for solving both classification and regression problems. By following the easy steps outlined in this guide, you now have the knowledge and skills to implement decision trees in your own machine learning projects.
FAQs
What are decision trees?
Decision trees are a type of supervised learning algorithm that is used for both classification and regression tasks. They work by recursively splitting the data into subsets based on the most significant attribute at each node.
What is the main library used for implementing decision trees in Python?
The main library used for implementing decision trees in Python is scikit-learn
, which provides a wide range of machine learning algorithms and tools for data analysis.
Can decision trees be used for regression tasks?
Yes, decision trees can be used for both classification and regression tasks. In regression tasks, decision trees work by predicting the output value for a given input data point.
Is it necessary to preprocess the data before training the decision tree?
Yes, it is important to preprocess the data by splitting it into training and testing sets before training the decision tree. This helps to evaluate the model’s performance accurately.