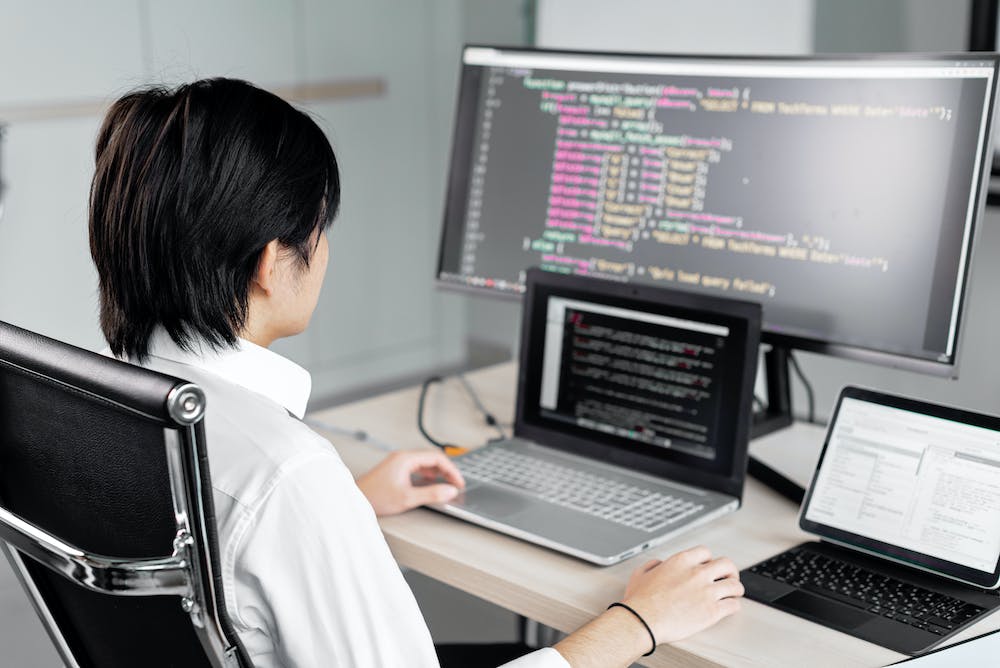
OpenCV, short for Open Source computer Vision Library, is an open-source computer vision and machine learning software library. IT is widely used in a variety of applications such as image processing, object detection, and feature recognition. Visual Studio Code is a popular integrated development environment (IDE) for building and debugging modern web and cloud applications. In this article, we will explore how to harness the power of OpenCV in Visual Studio Code with some mind-blowing tips and tricks!
Setting up OpenCV in Visual Studio Code
The first step in harnessing the power of OpenCV in Visual Studio Code is to set up the development environment. Here’s how to do it:
- Install Visual Studio Code: If you haven’t already, download and install Visual Studio Code from the official Website.
- Install the C/C++ extension: Open Visual Studio Code and install the C/C++ extension by clicking on the Extensions icon on the Activity Bar on the side of the window. Search for “C/C++” and click “Install”.
- Install OpenCV: Next, you’ll need to install OpenCV. You can download the latest version of OpenCV from the official website and follow the installation instructions for your operating system.
- Create a new C++ project: Once you have Visual Studio Code and OpenCV installed, you can create a new C++ project in Visual Studio Code and configure the necessary build settings to link to the OpenCV library.
Using OpenCV in Visual Studio Code
Now that you have set up OpenCV in Visual Studio Code, let’s explore some mind-blowing tips and tricks for harnessing its power:
1. Image loading and display
OpenCV provides a simple and powerful interface for loading and displaying images. You can use the imread()
function to load an image from a file, and the imshow()
function to display the image in a window.
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("image.jpg");
imshow("Image", image);
waitKey(0);
return 0;
}
2. Image processing
OpenCV offers a wide range of image processing functions, from basic operations like resizing and cropping to advanced techniques like edge detection and color manipulation. By harnessing the power of these functions, you can enhance and manipulate images in ways you never thought possible.
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("image.jpg");
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY);
Canny(gray, gray, 50, 150);
imshow("Edges", gray);
waitKey(0);
return 0;
}
3. Object detection
OpenCV also provides tools for object detection and recognition, such as the Haar feature-based cascade classifiers. By training these classifiers with positive and negative images, you can detect and localize objects in any given image with remarkable accuracy.
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("faces.jpg");
CascadeClassifier face_cascade;
face_cascade.load("haarcascade_frontalface_default.xml");
std::vector<Rect> faces;
face_cascade.detectMultiScale(image, faces, 1.1, 2, 0 | CASCADE_SCALE_IMAGE, Size(30, 30));
for (Rect face : faces) {
rectangle(image, face, Scalar(255, 0, 0), 2);
}
imshow("Faces", image);
waitKey(0);
return 0;
}
Conclusion
In conclusion, harnessing the power of OpenCV in Visual Studio Code opens up a world of possibilities for computer vision and machine learning applications. With the right tools and techniques, you can create stunning visual effects, automate image processing tasks, and build intelligent systems that can see and understand the world around them.
FAQs
Q: Can I use OpenCV with other programming languages in Visual Studio Code?
A: Yes, Visual Studio Code supports a wide range of programming languages, including C++, Python, and JavaScript, all of which are compatible with OpenCV.
Q: Is OpenCV difficult to learn?
A: While OpenCV has a steep learning curve due to its extensive features and functionalities, there are plenty of resources and tutorials available online to help you get started.
Q: Where can I find more information about OpenCV and Visual Studio Code integration?
A: You can find more information and resources on the official OpenCV and Visual Studio Code websites, as well as on developer forums and communities such as Stack Overflow and GitHub.