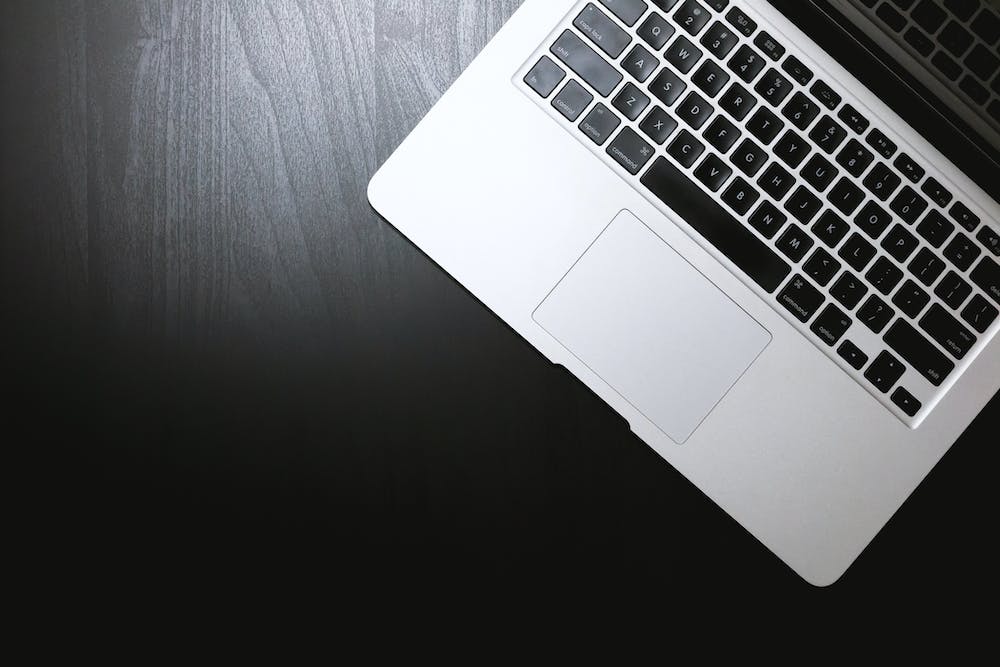
QR codes have become an integral part of our daily lives. From scanning product information to sharing contact details, QR codes provide a quick and convenient way to access and exchange information. In this article, we will explore how to create and read QR codes using OpenCV, a powerful computer vision library. You’ll be surprised at how simple IT is!
Creating QR codes with OpenCV
OpenCV is a popular open-source computer vision and machine learning software library. It provides various tools and functions for image processing, including the ability to generate QR codes. To create a QR code using OpenCV, you can use the following Python code:
import cv2
import numpy as np
import qrcode
# Create QR code instance
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
# Add data to the QR code
data = "https://www.example.com"
qr.add_data(data)
qr.make(fit=True)
# Create an image from the QR code
img = qr.make_image(fill_color="black", back_color="white")
# Save the image
img.save("qr_code.png")
This code snippet demonstrates how to generate a QR code using the qrcode
library and then save it as an image using OpenCV. The data
variable contains the information you want to encode in the QR code, such as a URL or a text message. After running the code, you will have a file named qr_code.png
containing the generated QR code.
Reading QR codes with OpenCV
Once you have a QR code, you may want to read the information encoded in it. OpenCV provides a simple way to achieve this using the cv2
module. Here’s an example of how to read a QR code from an image:
import cv2
from pyzbar.pyzbar import decode
# Read the image containing the QR code
img = cv2.imread("qr_code.png")
# Decode the QR code
decoded_objects = decode(img)
# Print the decoded data
for obj in decoded_objects:
print("Data:", obj.data)
In this code snippet, we use the pyzbar
library to decode the QR code from the image. The decode
function returns a list of decoded objects, each containing the data and the type of the barcode. You can then extract and use the decoded data as per your requirements.
Enhancing QR code detection
While the above examples provide a basic introduction to creating and reading QR codes with OpenCV, there are several ways to enhance the functionality and accuracy of QR code detection. Here are a few tips to consider:
- Image preprocessing: Before attempting to read a QR code from an image, it’s beneficial to perform image preprocessing techniques such as thresholding, edge detection, and noise reduction to improve the quality of the input image.
- Camera calibration: When working with real-time QR code detection from a camera feed, it’s essential to calibrate the camera to correct for lens distortion and other intrinsic parameters. OpenCV provides tools for camera calibration that can significantly improve the accuracy of QR code detection.
- Parameter tuning: The performance of QR code detection can be fine-tuned by adjusting parameters such as the QR code size, error correction level, and image resolution. Experimenting with these parameters can lead to better detection results.
- Integration with other libraries: OpenCV can be integrated with other computer vision libraries such as ZBar and zxing for additional QR code decoding capabilities. Combining the strengths of multiple libraries can improve the overall robustness of QR code detection.
Conclusion
Creating and reading QR codes with OpenCV is a straightforward process that offers a wide range of possibilities for applications in various domains. Whether you’re building a mobile app for QR code scanning or implementing QR code-based authentication systems, OpenCV provides the tools and flexibility to achieve your goals. By understanding the fundamentals of QR code generation and detection, you can leverage OpenCV to streamline your projects and enhance user experiences.
FAQs
Q: Can OpenCV handle colored QR codes?
A: Yes, OpenCV has the capability to handle colored QR codes. The image preprocessing techniques and QR code detection algorithms provided by OpenCV can effectively handle colored QR codes with high accuracy.
Q: What are some common applications of QR code detection using OpenCV?
A: Common applications of QR code detection using OpenCV include mobile payment systems, inventory management, access control systems, and augmented reality interactions. OpenCV’s flexibility and performance make it suitable for a wide range of QR code-based applications.
Q: Are there any limitations to QR code detection with OpenCV?
A: While OpenCV provides robust QR code detection capabilities, there may be limitations in handling highly distorted or damaged QR codes. In such cases, additional image processing techniques or the use of specialized libraries may be required to improve detection accuracy.
Q: Can I use OpenCV for real-time QR code detection from a live video stream?
A: Yes, OpenCV offers tools and functions for real-time QR code detection from a live video stream. By utilizing OpenCV’s video processing capabilities, you can implement real-time QR code detection in applications such as barcode scanning apps and interactive displays.
Q: Is it possible to generate dynamic QR codes with OpenCV?
A: While OpenCV itself does not provide functionality for dynamic QR code generation, it can be combined with other libraries and server-side technologies to generate dynamic QR codes. Dynamic QR codes allow for the inclusion of variable data such as timestamps and user-specific information.
Q: Can I use OpenCV for QR code recognition in non-standard orientations?
A: Yes, OpenCV offers rotation-invariant QR code detection capabilities, allowing for QR codes in non-standard orientations to be accurately recognized. By leveraging OpenCV’s feature detection and geometric transformation functions, QR codes can be detected and decoded irrespective of their orientation.