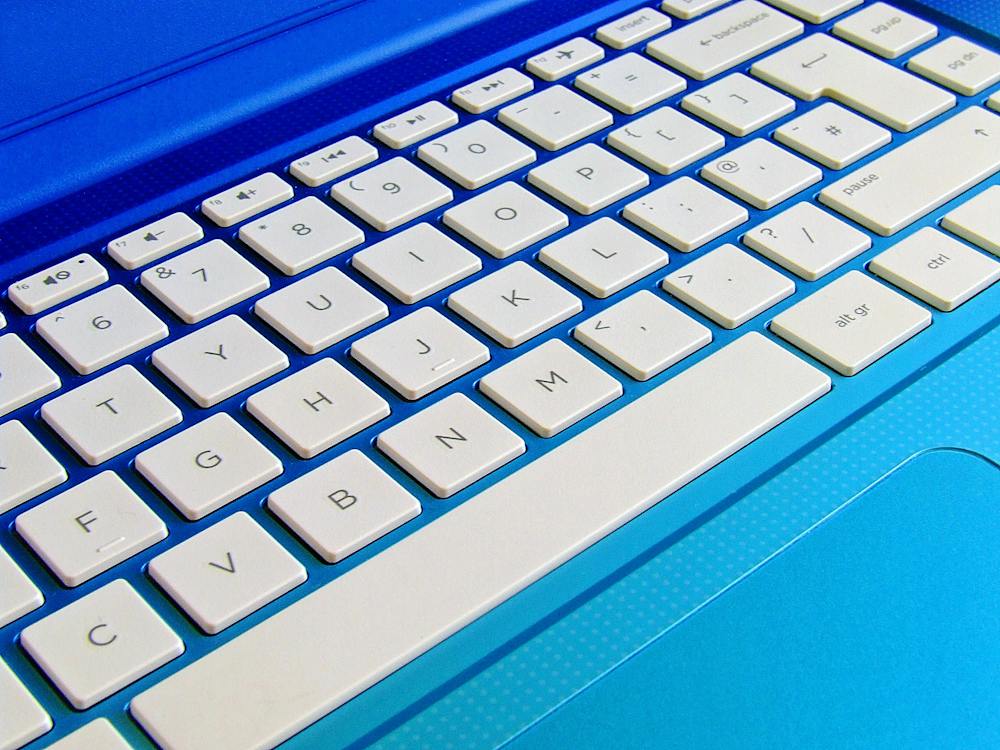
Have you ever been fascinated by the idea of creating secret codes and encrypting your messages? With the Caesar Cipher program in Python, you can learn how to do just that. The Caesar Cipher is one of the simplest and most widely known encryption techniques. IT is a type of substitution cipher in which each letter in the plaintext is shifted a certain number of places down or up the alphabet. By using this program, you can learn how to create your own unbreakable secret code to protect your sensitive information.
What is the Caesar Cipher?
The Caesar Cipher, also known as the shift cipher, is one of the oldest and simplest forms of encryption. It was used by Julius Caesar to communicate secretly with his generals during military campaigns. The technique involves shifting each letter in the plaintext by a certain number of positions down or up the alphabet. For example, with a shift of 3, A would be replaced by D, B would become E, and so on.
How does the Caesar Cipher program work?
The Caesar Cipher program in Python works by taking a plaintext message and a key, which indicates the number of positions each letter should be shifted. It then encrypts the message by applying the shift to each letter. For example, if the plaintext message is “HELLO” and the key is 3, the encrypted message would be “KHOOR”. To decrypt the message, the process is reversed by shifting the letters back by the same number of positions.
Creating the Caesar Cipher program in Python
Below is the Python code for implementing the Caesar Cipher program:
“`python
def caesar_cipher(text, shift):
encrypted_text = “”
for char in text:
if char.isalpha():
shift_amount = shift % 26
if char.islower():
shifted_char = chr((ord(char) – ord(‘a’) + shift_amount) % 26 + ord(‘a’))
else:
shifted_char = chr((ord(char) – ord(‘A’) + shift_amount) % 26 + ord(‘A’)
encrypted_text += shifted_char
else:
encrypted_text += char
return encrypted_text
plaintext = “SECRETMESSAGE”
key = 3
encrypted_message = caesar_cipher(plaintext, key)
print(encrypted_message) # Output: VHFUHWPHVVRPH
“`
Using the Caesar Cipher program in Python
To use the Caesar Cipher program, simply input the plaintext message and the key into the `caesar_cipher` function. The program will then return the encrypted message. You can also decrypt the message by applying the reverse shift to the encrypted text.
Advantages of the Caesar Cipher
The Caesar Cipher is a simple yet effective way to encrypt messages. It is easy to understand and implement, making it a great learning tool for beginners. Additionally, the Caesar Cipher can be used as a building block for more complex encryption techniques.
Conclusion
With the Caesar Cipher program in Python, you can learn how to create your own unbreakable secret code to protect your sensitive information. This simple yet powerful encryption technique has been used for centuries and continues to be relevant in the digital age. By understanding and implementing the Caesar Cipher, you can gain valuable knowledge in the field of cryptography and information security.
FAQs
Q: Is the Caesar Cipher secure?
A: The Caesar Cipher is a very basic form of encryption and can be easily cracked by modern cryptographic techniques. It is more of a learning tool than a secure encryption method for sensitive information.
Q: Can I use the Caesar Cipher for real-world encryption?
A: While the Caesar Cipher is not suitable for securing highly sensitive information, it can be used for educational purposes and as a stepping stone to more advanced encryption techniques.
Q: How can I improve the security of the Caesar Cipher?
A: One way to enhance the security of the Caesar Cipher is to use a more complex key, such as a random sequence of numbers or a passphrase. Additionally, combining the Caesar Cipher with other encryption methods can increase its security.