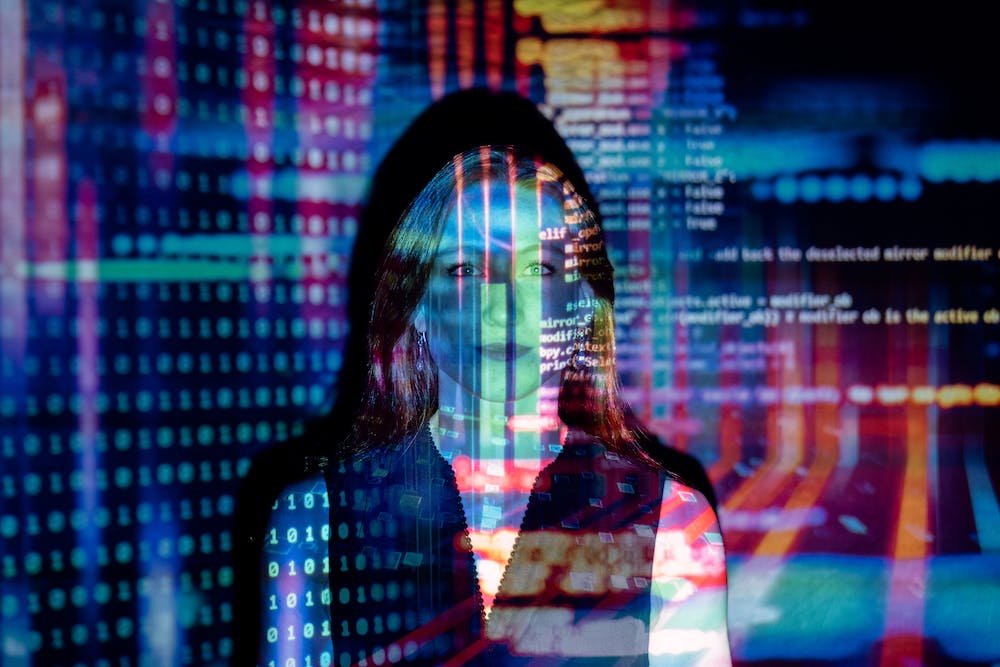
Python is a powerful and versatile programming language that can be used to create a wide range of applications, including games. In this article, we will walk you through the process of creating a Tic Tac Toe game in Python. By the end of this tutorial, you will have a fully functional game that you can play and share with your friends.
Getting Started
Before we dive into the code, make sure you have Python installed on your computer. You can download IT from the official Website and follow the installation instructions. Once you have Python installed, open your favorite code editor and create a new file for our Tic Tac Toe game.
The Game Board
The first step in creating our Tic Tac Toe game is to create the game board. We will represent the board as a 3×3 grid, and we will use a list of lists to store the game state. Here’s how we can initialize the game board:
game_board = [[' ', ' ', ' '],
[' ', ' ', ' '],
[' ', ' ', ' ']]
Each element in the list represents a row in the game board, and each row contains three elements representing the columns. We will use the ‘X’ and ‘O’ characters to mark the positions of the players on the board.
Displaying the Board
Next, we will create a function to display the game board to the players. We will iterate through the game board and print the current state of each cell. Here’s the code for the display_board function:
def display_board(board):
for row in board:
print('|'.join(row))
print('-----')
Now that we have a way to represent and display the game board, we can move on to allowing players to make moves and checking for a winner.
Implementing Player Moves
We will create a function that allows players to make moves on the game board. The function will take the player’s symbol (‘X’ or ‘O’) and the position they want to play as input, and update the game board accordingly. Here’s the code for the make_move function:
def make_move(board, player, row, col):
if board[row][col] == ' ':
board[row][col] = player
return True
else:
return False
With this function, players can now make moves on the game board. However, we need to add logic to check for a winner after each move.
Checking for a Winner
To determine if there is a winner, we will create a function that checks the rows, columns, and diagonals of the game board for a winning combination. If a player has three of their symbols in a row, column, or diagonal, they win the game. Here’s the code for the check_winner function:
def check_winner(board, player):
for i in range(3):
if board[i][0] == player and board[i][1] == player and board[i][2] == player:
return True
if board[0][i] == player and board[1][i] == player and board[2][i] == player:
return True
if board[0][0] == player and board[1][1] == player and board[2][2] == player:
return True
if board[0][2] == player and board[1][1] == player and board[2][0] == player:
return True
return False
Now that we have functions to allow players to make moves and check for a winner, we can implement the game loop to handle the flow of the game.
The Game Loop
We will create a main function that represents the game loop. The game loop will alternate between the two players, allowing each player to make a move and checking for a winner after each move. Here’s the code for the game_loop function:
def game_loop():
game_board = [[' ', ' ', ' '],
[' ', ' ', ' '],
[' ', ' ', ' ']]
player = 'X'
while True:
display_board(game_board)
row = int(input("Enter the row for your move (0, 1, 2): "))
col = int(input("Enter the column for your move (0, 1, 2): "))
if make_move(game_board, player, row, col):
if check_winner(game_board, player):
print(f"Player {player} wins!")
break
if all(all(cell != ' ' for cell in row) for row in game_board):
print("It's a tie!")
break
player = 'O' if player == 'X' else 'X'
else:
print("Invalid move. Try again.")
With the game loop in place, players can now play the Tic Tac Toe game. The game will continue until one of the players wins or there is a tie. Congratulations! You have successfully created an epic Tic Tac Toe game in Python!
Conclusion
In this article, we walked you through the process of creating a Tic Tac Toe game in Python. We started by creating the game board, then implemented functions for displaying the board, allowing players to make moves, and checking for a winner. Finally, we created a game loop to handle the flow of the game. By following this tutorial, you have learned how to create a fully functional game that you can play and share with others. We hope you enjoyed this tutorial and have fun playing your new Tic Tac Toe game!
FAQs
Q: Is Python a good language for game development?
A: Yes, Python is a versatile language that can be used to create a wide range of games, from simple text-based games to complex 3D simulations.
Q: Can I add more features to the Tic Tac Toe game?
A: Absolutely! Once you have the basic game working, you can add features such as a graphical user interface, an AI opponent, or a multiplayer mode.
Q: Where can I learn more about game development in Python?
A: There are many resources available online for learning game development in Python, including tutorials, forums, and community websites.
Thank you for reading!