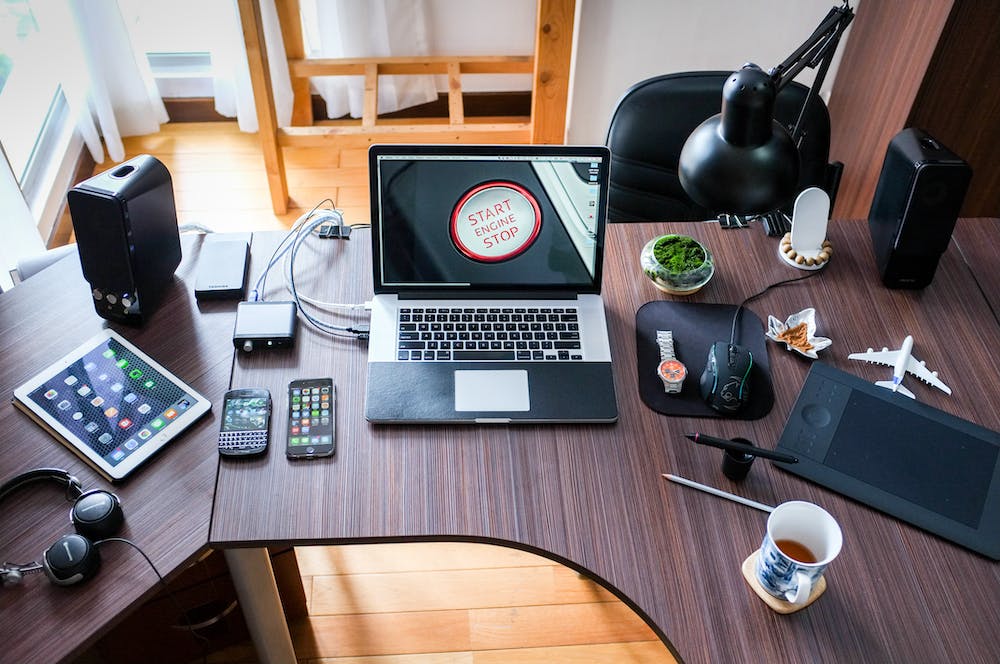
Python is a powerful programming language that is widely used for various applications, including web development, data analysis, machine learning, and more. One of the great things about Python is that IT is easy to learn and use, even for beginners. In this article, we will show you how to create amazing small Python programs in just 10 minutes! Whether you are a beginner or an experienced programmer, you will find these tips helpful in enhancing your coding skills.
Step 1: Set Up Your Development Environment
Before you can start writing Python programs, you need to set up your development environment. There are various ways to do this, but the most common method is to use an integrated development environment (IDE) such as PyCharm, Visual Studio Code, or Jupyter Notebook. These IDEs provide a user-friendly interface for writing and running Python code, as well as tools for debugging and testing your programs.
If you are new to Python, you can also start with an online code editor like Repl.it or Codecademy, which allows you to write and run Python code directly in your web browser without any installation required.
Step 2: Write Your First Python Program
Now that you have set up your development environment, it’s time to write your first Python program. For this example, let’s create a simple program that prints “Hello, World!” to the console:
“`python
print(“Hello, World!”)
“`
Save the above code in a file with a .py extension, for example, hello.py. Then, you can run the program by opening a terminal or command prompt, navigating to the directory where the file is located, and typing python hello.py. You should see “Hello, World!” printed to the console.
Step 3: Explore Python’s Built-in Functions and Libraries
Python comes with a rich set of built-in functions and libraries that make it easy to perform various tasks without writing complex code from scratch. For example, you can use the built-in input() function to get user input, the math library for mathematical operations, and the random library for generating random numbers.
Let’s take a look at a simple example that uses the random library to generate a random number between 1 and 10:
“`python
import random
random_number = random.randint(1, 10)
print(“Random number: “, random_number)
“`
Save the above code in a file with a .py extension, for example, random_number.py, and run it as we did before. You should see a random number printed to the console each time you run the program.
Step 4: Create Your Own Functions and Modules
As you become more comfortable with Python, you can start creating your own functions and modules to organize and reuse your code. Functions allow you to encapsulate a block of code that performs a specific task, while modules are files that contain a collection of related functions and variables.
Here’s an example of how you can create a simple function that calculates the area of a circle using the radius as input:
“`python
import math
def calculate_area(radius):
return math.pi * radius ** 2
radius = 5
area = calculate_area(radius)
print(“Area of the circle with radius”, radius, “is”, area)
“`
Save the above code in a file with a .py extension, for example, circle_area.py, and run it to see the calculated area of the circle printed to the console.
Step 5: Utilize Control Structures and Loops
Python provides various control structures and loops that allow you to manipulate the flow of your program and repeat certain tasks as needed. For example, you can use if-else statements to make decisions, while and for loops to iterate over lists, dictionaries, or other collections, and break and continue statements to control the flow of the loop.
Let’s create a simple program that uses a for loop to print the first 10 even numbers:
“`python
for i in range(2, 21, 2):
print(i)
“`
Save the above code in a file with a .py extension, for example, even_numbers.py, and run it to see the first 10 even numbers printed to the console.
Step 6: Practice, Practice, Practice!
The best way to improve your Python programming skills is to practice regularly. Try solving coding challenges, participate in programming contests, and work on small projects to apply what you have learned. By practicing consistently, you will become more comfortable with Python and gain confidence in writing complex programs.
Conclusion
Learning to create amazing small Python programs in just 10 minutes is an achievable goal for anyone, regardless of their experience level. By following the steps outlined in this article and practicing regularly, you can enhance your coding skills and build a solid foundation in Python programming. With its simplicity and versatility, Python is an excellent language for beginners to start their coding journey and for experienced programmers to tackle challenging projects.
FAQs
Q: Do I need to have programming experience to learn Python?
A: No, Python is a beginner-friendly language with a simple and easy-to-understand syntax, making it suitable for individuals with little to no programming experience.
Q: Can I create more complex programs using Python?
A: Absolutely! Python is a powerful language capable of handling complex projects, including web development, data analysis, artificial intelligence, and more. As you gain proficiency, you can explore advanced concepts and tools to build sophisticated applications.
Q: How can I further improve my Python programming skills?
A: Besides practicing regularly, consider joining coding communities, attending workshops and meetups, and seeking mentorship from experienced programmers. Online resources such as tutorials, documentation, and coding forums can also provide valuable insights and guidance.