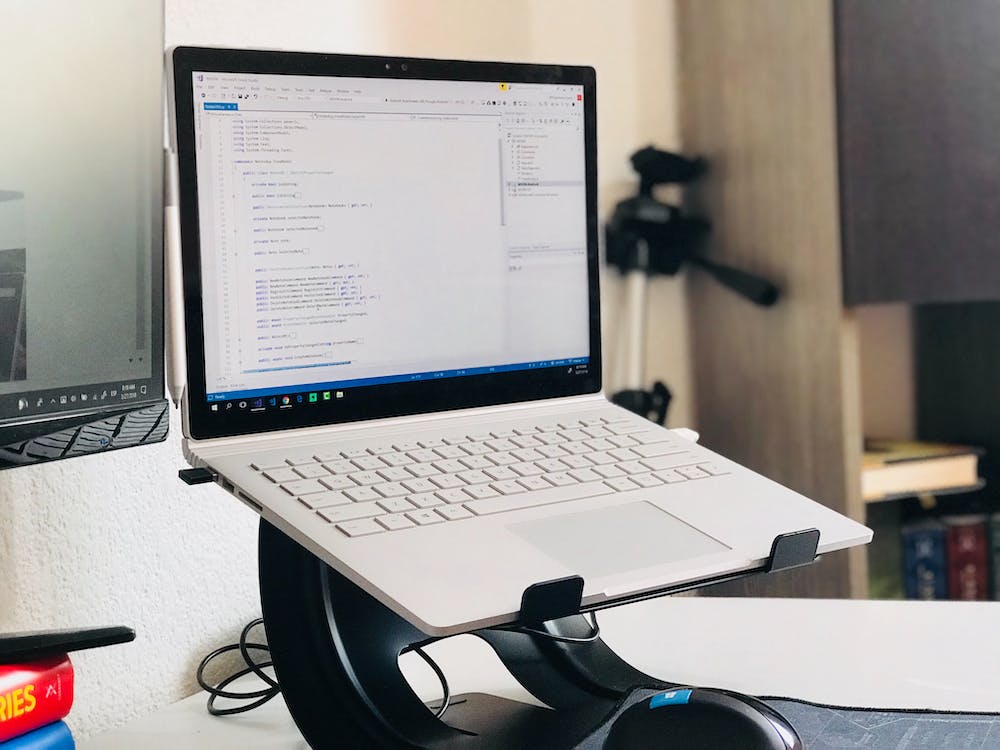
Python is a powerful and versatile programming language that is widely used for building games and applications. In this tutorial, we will explore how to create a mesmerizing snake game using Python with just a few simple steps. By the end of this tutorial, you will have a fully functional snake game that you can play and share with your friends.
Prerequisites
Before we dive into the tutorial, there are a few things you’ll need to have in place. Make sure you have the following:
- A computer with Python installed
- A code editor such as Visual Studio Code or Sublime Text
Step 1: Setting Up Your Development Environment
The first step is to set up your development environment. If you don’t have Python installed on your computer, you can download IT from the official Python Website. Once you have Python installed, you can use a code editor of your choice to write and run your Python code.
Step 2: Creating the Game Window
Now that your development environment is set up, it’s time to start creating the snake game. The first thing you’ll need to do is create the game window. You can use the pygame library to create the game window and handle user input. Here’s a simple example of how to create a game window using pygame:
“`python
import pygame
# Initialize the pygame library
pygame.init()
# Set up the game window
window_width = 800
window_height = 600
game_window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption(‘Snake Game’)
# Run the game loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
“`
In this example, we initialize the pygame library, set up the game window with a width of 800 pixels and a height of 600 pixels, and create a game loop that will keep the game running until the user closes the window.
Step 3: Creating the Snake
With the game window in place, the next step is to create the snake. You can represent the snake as a series of rectangles that move around the game window. Here’s a simple example of how to create the snake:
“`python
class Snake:
def __init__(self):
self.length = 1
self.positions = [((window_width / 2), (window_height / 2))]
self.direction = RIGHT
self.color = (0, 255, 0) # green
def get_head_position(self):
return self.positions[0]
def turn(self, point):
if self.length > 1 and (point[0] * -1, point[1] * -1) == self.direction:
return
else:
self.direction = point
def move(self):
cur = self.get_head_position()
x, y = self.direction
new = (((cur[0] + (x*GRIDSIZE)) % window_width), (cur[1] + (y*GRIDSIZE)) % window_height)
if len(self.positions) > 2 and new in self.positions[2:]:
self.reset()
else:
self.positions.insert(0, new)
if len(self.positions) > self.length:
self.positions.pop()
def reset(self):
self.length = 1
self.positions = [((window_width / 2), (window_height / 2))]
self.direction = RIGHT
def draw(self, surface):
for p in self.positions:
r = pygame.Rect((p[0], p[1]), (GRIDSIZE, GRIDSIZE))
pygame.draw.rect(surface, self.color, r)
pygame.draw.rect(surface, (93, 216, 228), r, 1)
“`
In this example, we define a Snake class that represents the snake in the game. The Snake class has methods for moving the snake, turning the snake in a new direction, and drawing the snake on the game window. We also define the initial position, length, and color of the snake.
Step 4: Handling User Input
Now that the snake is in place, you’ll need to handle user input to control the snake. You can use the pygame library to capture user input and update the snake’s direction accordingly. Here’s an example of how to handle user input:
“`python
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.turn(UP)
elif event.key == pygame.K_DOWN:
snake.turn(DOWN)
elif event.key == pygame.K_LEFT:
snake.turn(LEFT)
elif event.key == pygame.K_RIGHT:
snake.turn(RIGHT)
“`
In this example, we listen for KEYDOWN events and update the snake’s direction based on the user’s input. If the user presses the UP arrow key, the snake will turn upwards; if the user presses the DOWN arrow key, the snake will turn downwards; and so on.
Step 5: Adding Food and Collision Detection
At this point, you have a snake that can move around the game window based on user input. The next step is to add food for the snake to eat and implement collision detection. Here’s a simple example of how to add food and detect collisions in the snake game:
“`python
def draw_food(surface, color):
food_rect = pygame.Rect((food[0], food[1]), (GRIDSIZE, GRIDSIZE))
pygame.draw.rect(surface, color, food_rect)
pygame.draw.rect(surface, (93, 216, 228), food_rect, 1)
food = (random.randint(0, GRID_WIDTH-1) * GRIDSIZE, random.randint(0, GRID_HEIGHT-1) * GRIDSIZE)
score = 0
if snake.get_head_position() == food:
snake.length += 1
score += 1
food = (random.randint(0, GRID_WIDTH-1) * GRIDSIZE, random.randint(0, GRID_HEIGHT-1) * GRIDSIZE)
“`
In this example, we define a function to draw the food on the game window and use the random library to generate random positions for the food. We also implement collision detection to check if the snake has collided with the food. If the snake’s head position is the same as the position of the food, the snake eats the food, its length increases, and the score is updated.
Step 6: Adding Game Over Condition
Lastly, you’ll need to add a game over condition to end the game when the snake collides with itself or the game window boundaries. Here’s an example of how to implement the game over condition:
“`python
if snake.get_head_position() in snake.positions[1:]:
game_over()
if snake.get_head_position()[0] < 0 or snake.get_head_position()[0] >= window_width or snake.get_head_position()[1] < 0 or snake.get_head_position()[1] >= window_height:
game_over()
“`
In this example, we check if the snake’s head position is in the snake’s body, which indicates a collision with itself, or if the snake’s head position is outside the game window boundaries, which indicates a collision with the boundaries. If either condition is met, the game over function is called to end the game.
Conclusion
Congratulations! You have successfully created a mesmerizing snake game using Python with just a few simple steps. You have learned how to set up your development environment, create the game window, implement the snake, handle user input, add food and collision detection, and add a game over condition. You can now customize and enhance the game further with additional features and improvements. Have fun playing and sharing your snake game with others!
FAQs
Q: Can I customize the appearance of the snake and food?
A: Yes, you can customize the appearance of the snake and food by changing their colors, sizes, and shapes in the code.
Q: How can I add sound effects to the game?
A: You can use the pygame.mixer module to add sound effects to the game. Simply load the sound files and play them at the desired events in the game.
Q: Can I make the game multiplayer?
A: Yes, you can modify the game to support multiplayer by adding additional snake objects and handling user input for each player.
Q: What are some ways to optimize the game for better performance?
A: You can optimize the game by using efficient data structures, minimizing redundant code, and implementing game state management techniques.
Q: Where can I find more resources to learn about game development with Python?
A: You can explore online tutorials, forums, and communities dedicated to game development with Python. backlink works is a great resource for learning about Python game development and related topics.