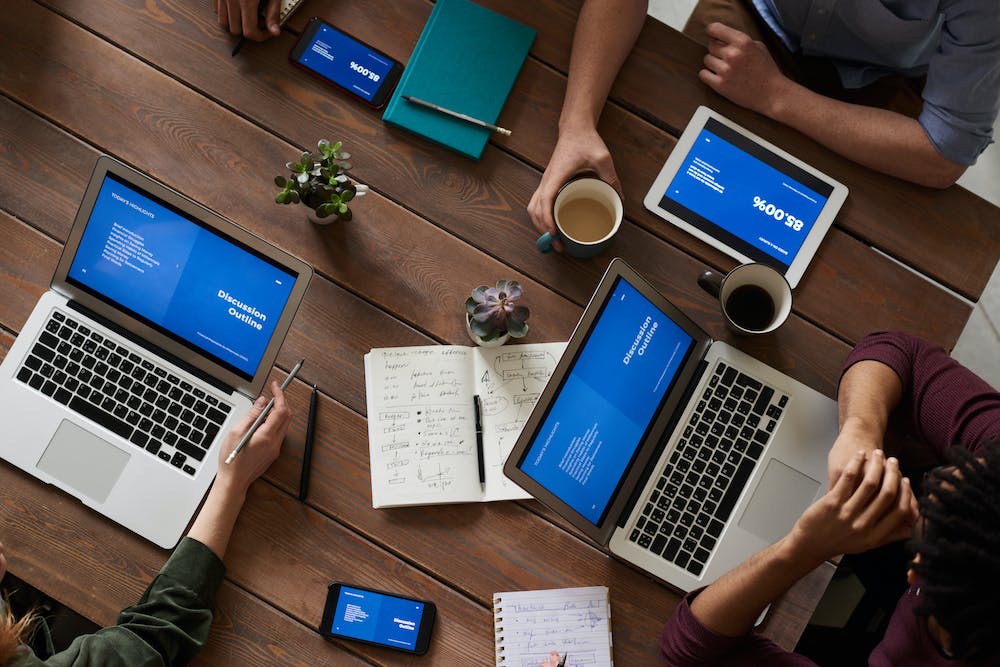
Python is one of the most popular programming languages in the world. IT is versatile, easy to learn, and has a wide range of applications. One of the many things you can do with Python is to code a powerful calculator. In this article, we will walk you through a few simple steps to create your own calculator in Python.
Step 1: Setting Up Your Environment
Before you start coding your calculator, you need to make sure you have Python installed on your computer. You can download Python from the official Website and follow the installation instructions for your operating system.
Step 2: writing the Code
Once you have Python installed, you can start coding your calculator. Open your favorite text editor or integrated development environment (IDE) and create a new Python file. You can name it something like calculator.py
.
First, let’s define the basic structure of our calculator program. We will create a class called Calculator
that will contain all the functionality of our calculator. Here’s what the initial code might look like:
class Calculator:
def add(self, x, y):
return x + y
def subtract(self, x, y):
return x - y
# Other functions for multiplication, division, etc.
In the above code, we have defined a class called Calculator
with two methods, add
and subtract
. These methods take two arguments, x
and y
, and perform addition and subtraction operations respectively. You can continue adding methods for multiplication, division, and any other operations you want to include in your calculator.
Step 3: Creating a User Interface
Now that we have the basic functionality of our calculator, it’s time to create a user interface for it. We can use Python’s built-in input
function to get input from the user and display the results using the print
function. Here’s an example of how you can create a simple command-line interface for your calculator:
calc = Calculator()
while True:
print("Options:")
print("Enter 'add' to add two numbers")
print("Enter 'subtract' to subtract two numbers")
print("Enter 'quit' to end the program")
user_input = input(": ")
if user_input == "quit":
break
elif user_input == "add":
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
print("Result: ", calc.add(num1, num2))
elif user_input == "subtract":
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
print("Result: ", calc.subtract(num1, num2))
else:
print("Unknown command")
In the above code, we create an instance of the Calculator
class and then ask the user for input. Depending on the input, we perform the corresponding operation and display the result. This provides a simple command-line interface for our calculator.
Step 4: Enhancing the Calculator
While the above code provides a basic calculator functionality, you can enhance it further by adding error handling, support for more operations, and a more user-friendly interface. You can also explore libraries like tkinter
to create a graphical user interface (GUI) for your calculator.
Conclusion
Creating a powerful calculator in Python is a great way to practice your programming skills and explore the versatility of the language. By following the simple steps outlined in this article, you can create your own calculator with just a few lines of code. Feel free to experiment with different features and functionalities to customize your calculator to suit your needs.
FAQs
Q: Can I use this calculator for complex mathematical operations?
A: While the basic calculator provided in this article is meant for simple arithmetic operations, you can expand its functionality to support more complex mathematical operations if needed.
Q: Can I create a graphical user interface for the calculator?
A: Yes, you can use libraries like tkinter
to create a GUI for your calculator, providing a more user-friendly interface for your application.
Q: Is it possible to add scientific functions to the calculator?
A: Absolutely! You can extend the functionality of the calculator to include scientific functions like trigonometric functions, logarithms, and more.
Q: Can I use this calculator for my own projects or applications?
A: Yes, you can tailor the calculator to fit the needs of your specific projects or applications, making it a versatile and valuable tool in your programming arsenal.
Q: Where can I find additional resources to expand my knowledge of Python programming?
A: backlink works offers a wide range of resources and tutorials for Python programming, providing you with the knowledge and skills to excel in your programming journey.