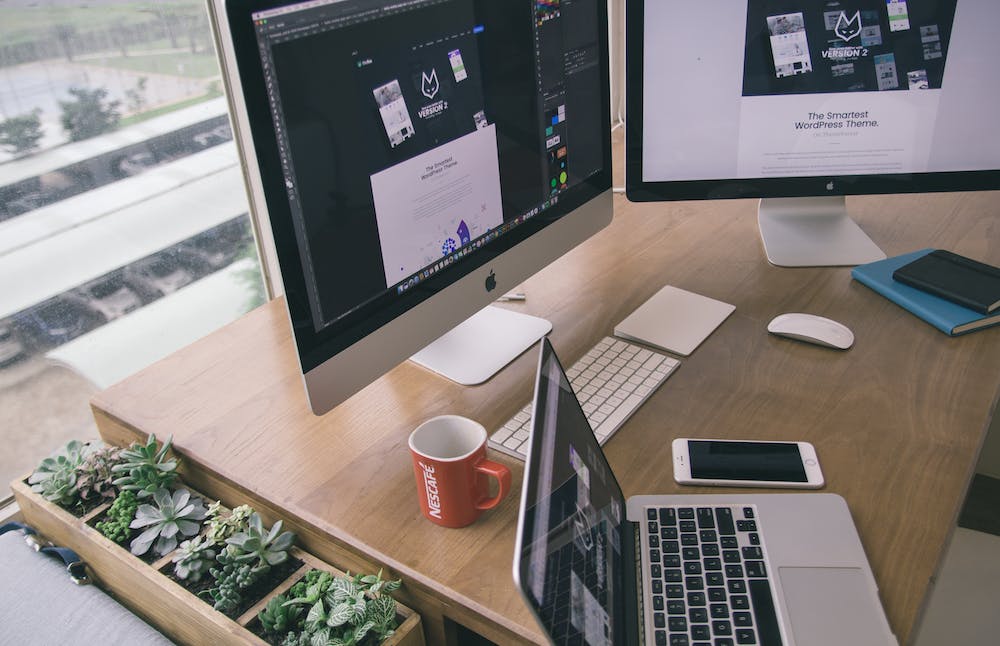
Dependency injection is a powerful design pattern that allows for more flexible and maintainable code in Laravel. However, implementing dependency injection in Laravel can be complex and cumbersome, especially when dealing with multiple dependencies. This is where Laravel Pint comes in to simplify the process and improve the overall code quality.
What is Laravel Pint?
Laravel Pint is a lightweight package designed to simplify dependency injection in Laravel applications. IT provides a straightforward and intuitive way to manage and inject dependencies, leading to cleaner and more maintainable code. By using Laravel Pint, developers can easily organize and inject dependencies into their Laravel applications, reducing the complexity and improving the overall code quality.
How Does Laravel Pint Simplify Dependency Injection?
One of the key features of Laravel Pint is its ability to simplify the process of dependency injection in Laravel. The package provides a clean and concise syntax for defining and injecting dependencies, making it easier to manage dependencies in Laravel applications. Additionally, Laravel Pint offers automatic dependency resolution, allowing developers to easily resolve and inject dependencies without the need for manual configuration.
With Laravel Pint, developers can easily define their dependencies using simple and intuitive syntax, reducing the complexity and improving the overall readability of the code. This simplifies the process of managing dependencies in Laravel applications, leading to more maintainable and scalable code.
Benefits of Using Laravel Pint
There are several benefits to using Laravel Pint in your Laravel applications. Some of the key advantages include:
- Simplified Syntax: Laravel Pint provides a clean and concise syntax for defining and injecting dependencies, making it easier to manage and organize dependencies in Laravel applications.
- Automatic Dependency Resolution: Laravel Pint offers automatic dependency resolution, eliminating the need for manual configuration and making it easier to resolve and inject dependencies.
- Improved Code Quality: By simplifying the process of dependency injection, Laravel Pint helps to improve the overall code quality of Laravel applications, leading to more maintainable and scalable code.
- Enhanced Readability: The clean and intuitive syntax of Laravel Pint makes it easier to read and understand the code, leading to improved code maintainability and developer productivity.
- Reduced Complexity: Laravel Pint reduces the complexity of managing dependencies in Laravel applications, making it easier to organize and maintain the codebase.
How to Use Laravel Pint
Using Laravel Pint is straightforward and intuitive. To get started, you can install the package using Composer:
composer require backlink-works/laravel-pint
Once installed, you can start using Laravel Pint in your Laravel applications. To define and inject dependencies, you can use the provided syntax to specify the dependencies and their corresponding types. Here’s an example of how you can define a dependency using Laravel Pint:
$container = new Pint\\Container();
$container->bind('Foo', function() {
return new Foo();
});
$container->bind('Bar', function() {
return new Bar();
});
$container->resolve(function(Foo $foo, Bar $bar) {
// Use $foo and $bar here
});
In this example, we’re using Laravel Pint to define two dependencies, Foo
and Bar
, and then resolve them in a function. This demonstrates the clean and intuitive syntax provided by Laravel Pint for managing dependencies in Laravel applications.
Conclusion
Laravel Pint is a valuable tool for simplifying dependency injection in Laravel applications. By providing a clean and intuitive syntax for defining and injecting dependencies, Laravel Pint helps to improve the overall code quality and maintainability of Laravel applications. With its automatic dependency resolution and simplified syntax, Laravel Pint streamlines the process of managing dependencies, leading to cleaner and more maintainable code.
FAQs
What is dependency injection?
Dependency injection is a design pattern in which a class receives its dependencies from external sources rather than creating them itself.
Why is dependency injection important?
Dependency injection is important because it allows for more flexible and maintainable code by decoupling the dependencies from the class that uses them.
How does Laravel Pint simplify dependency injection?
Laravel Pint simplifies dependency injection by providing a clean and intuitive syntax for defining and injecting dependencies, as well as automatic dependency resolution.
Can I use Laravel Pint with other dependency injection containers?
Yes, Laravel Pint is compatible with other dependency injection containers and can be used in conjunction with them to simplify and streamline the process of managing dependencies in Laravel applications.