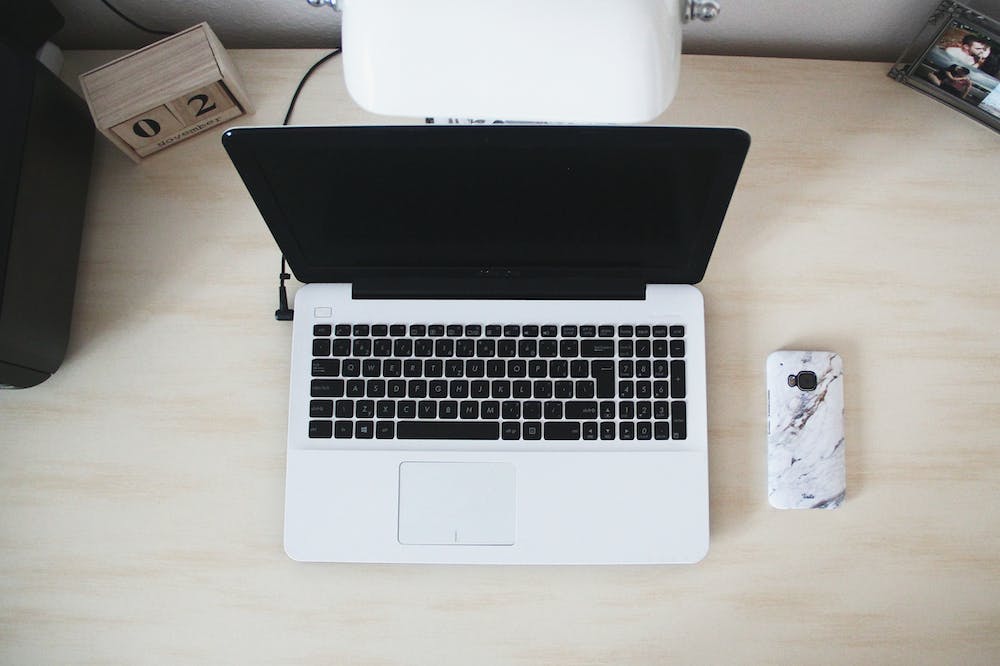
Laravel is a powerful PHP framework that offers a wide range of functionalities and features, making IT a popular choice among developers. One essential aspect of web development is ensuring the security of your application, especially when IT involves handling sensitive user data. Laravel provides excellent tools to secure your web application, and one of the most critical components is API authentication.
What is API Authentication?
API authentication is the process of verifying users’ identities when they interact with your application’s API endpoints. IT prevents unauthorized access and ensures that only authenticated users can access protected resources. API authentication is crucial when building applications that involve user registration, login, and managing user-specific data.
Laravel simplifies the implementation of API authentication by providing a robust authentication system out of the box. IT supports various authentication methods, including token-based, OAuth, and JWT authentication. This article will focus on token-based authentication with Laravel’s built-in features.
Token-based Authentication in Laravel
Laravel provides a simple and secure way to implement token-based authentication. IT works by generating a unique token for each authenticated user. This token is then sent with each subsequent API request to verify the user’s identity.
Here’s an example of how token-based authentication can be implemented in Laravel:
Route::post('/login', function () {
// validate user input
$credentials = request(['email', 'password']);
// attempt to authenticate the user
if (!auth()->attempt($credentials)) {
return response()->json(['error' => 'Unauthorized'], 401);
}
// generate a unique token for the user
$token = $user->createToken('api-token')->plainTextToken;
return response()->json(['token' => $token], 200);
});
In the above code snippet, we start by validating the user credentials. We then attempt to authenticate the user using Laravel’s built-in “attempt” method, which checks if the provided credentials are valid. If the authentication fails, we return an unauthorized response.
If the authentication succeeds, we generate a unique token for the user using the “createToken” method. This token is associated with the “api-token” name and is stored securely in the database. Finally, we return the generated token as a JSON response.
To protect API routes, Laravel provides a middleware called “auth:sanctum.” You can apply this middleware to any route that requires authentication. Here’s an example:
Route::middleware('auth:sanctum')->get('/profile', function () {
return response()->json(['user' => auth()->user()], 200);
});
In the above code snippet, we apply the “auth:sanctum” middleware to the “/profile” route. This middleware ensures that the user accessing the route is authenticated. If the authentication fails, Laravel automatically returns an unauthorized response.
Advantages of Laravel API Authentication
Laravel API authentication offers several advantages when IT comes to web security:
- Secure User Authentication: Laravel’s authentication system provides secure user authentication, preventing unauthorized access to sensitive user data.
- Token-based Authentication: Token-based authentication eliminates the need for username and password exchange with each request, improving performance and reducing the risk of credentials being intercepted.
- Easy Implementation: Laravel offers a straightforward API authentication implementation, allowing developers to focus on other aspects of their application.
- Scalability: Laravel API authentication is scalable, making IT suitable for applications of any size.
Conclusion
Laravel API authentication is an essential aspect of securing your web application. By implementing token-based authentication, you can ensure that only authenticated users have access to protected resources. Laravel provides a robust authentication system that simplifies the implementation process and offers various authentication methods.
Adopting Laravel API authentication not only fortifies your web security but also improves the performance and scalability of your application.
FAQs
Q: Can Laravel support OAuth authentication?
A: Yes, Laravel supports OAuth authentication out of the box. You can easily integrate OAuth providers like Google, Facebook, or Twitter into your application.
Q: Are there any rate-limiting features in Laravel for API authentication?
A: Yes, Laravel provides built-in rate-limiting features for API authentication. You can configure IT to prevent abuse or excessive requests from clients.
Q: Can I use Laravel API authentication for mobile applications?
A: Absolutely! Laravel API authentication works seamlessly with both web and mobile applications.
Q: Should I store tokens in the database?
A: Yes, Laravel securely stores the generated tokens in the database. You can also manage token revocation or expiration based on your application’s requirements.