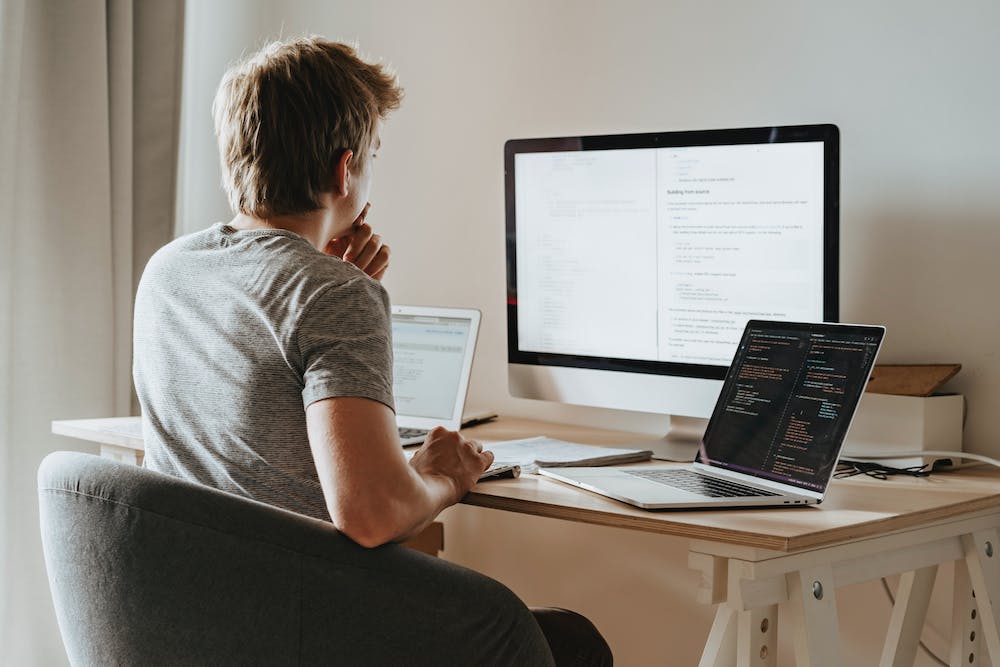
JavaScript is a popular programming language that is widely used for building dynamic websites and web applications. One of the key features of JavaScript is its ability to handle asynchronous tasks. In this article, we will explore how to implement a one-second delay using the setTimeout() function.
The setTimeout() function is a built-in JavaScript function that allows you to execute a specified piece of code after a certain amount of time has passed. This can be particularly useful when you want to introduce a delay between actions, such as displaying a message after a button click or animating the appearance of an element.
To use setTimeout(), you need to provide two parameters: the function you want to execute and the time delay in milliseconds. For example, if you want to display a message after one second, you can write the following code:
setTimeout(function(){
alert('Hello, world!');
}, 1000);
In the code above, we pass an anonymous function as the first parameter of setTimeout(), which will be executed after the delay of 1000 milliseconds (or one second). The alert() function is used to display a pop-up message with the text ‘Hello, world!’ when the timeout expires.
By using setTimeout() in this way, you can control the timing of different actions in your JavaScript code and create interactive and engaging user experiences.
FAQs
Q: Can I use setTimeout() to create delays longer than one second?
A: Yes, absolutely! The second parameter of setTimeout() represents the delay in milliseconds. If you want to introduce a delay of, let’s say, five seconds, you would pass 5000 as the second argument.
Q: Is setTimeout() limited to executing a single function?
A: No, you can pass any valid JavaScript expression or multiple function calls as the first parameter of setTimeout(). For example:
setTimeout(function(){
console.log('Delay complete!');
doSomethingElse();
}, 2000);
Q: Can I cancel a setTimeout() delay?
A: Yes, you can cancel a setTimeout() delay by using the clearTimeout() function. The clearTimeout() function takes the ID returned by setTimeout() as its parameter. For example:
var delayID = setTimeout(function(){
alert("This alert won't be displayed.");
}, 5000);
// Cancel the delay
clearTimeout(delayID);
Q: Are there any alternatives to using setTimeout() for delays?
A: Yes, there are other ways to introduce delays in JavaScript, such as using the setInterval() function or libraries/frameworks like jQuery or React that provide built-in delay functionality.
Q: Is setTimeout() supported in all web browsers?
A: Yes, the setTimeout() function is supported in all major web browsers, including Chrome, Firefox, Safari, and internet Explorer.
Overall, setTimeout() is a powerful tool in JavaScript that allows you to introduce delays and control the timing of actions in your code. By understanding how to use setTimeout(), you can enhance the user experience of your web applications and create more interactive and responsive websites.