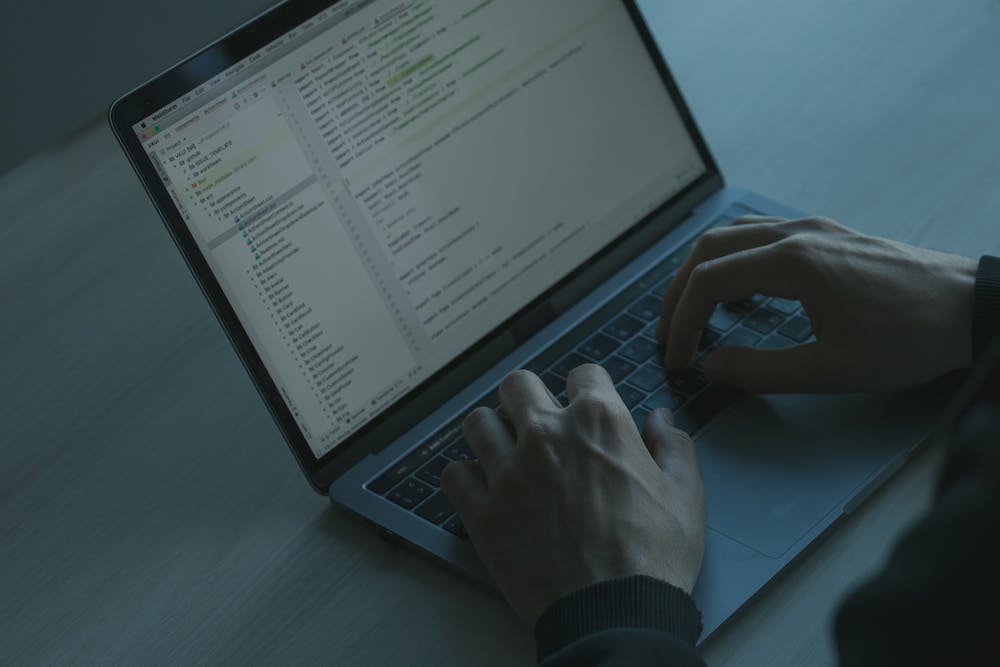
JavaScript is a powerful scripting language that allows developers to create dynamic and interactive web pages. One of the key features of JavaScript is its ability to manipulate the Document Object Model (DOM), which is the programming interface for HTML and XML documents. In this beginner’s guide, we’ll explore the basics of DOM manipulation using JavaScript.
What is the DOM?
The DOM is a tree-like structure that represents the elements on a web page. Each element, such as a paragraph, heading, or image, is represented as a node in the DOM tree. These nodes can be accessed and manipulated using JavaScript, allowing developers to dynamically change the content and appearance of a web page without having to manually edit the HTML.
Selecting DOM Elements
The first step in DOM manipulation is selecting the elements that you want to work with. JavaScript provides several methods for selecting DOM elements, such as document.getElementById
, document.getElementsByClassName
, and document.querySelector
. These methods allow you to select elements based on their ID, class, or CSS selector, respectively.
Example:
Let’s say we have the following HTML:
<div id="myDiv">
<p class="intro">Hello World!</p>
</div>
We can select the paragraph element using JavaScript:
var paragraph = document.querySelector('.intro');
console.log(paragraph.innerHTML); // Output: Hello World!
paragraph.innerHTML = 'Goodbye World!';
console.log(paragraph.innerHTML); // Output: Goodbye World!
Manipulating DOM Elements
Once you’ve selected the elements you want to work with, you can manipulate them in a variety of ways. For example, you can change the content of an element, modify its style, or add and remove classes or attributes.
Example:
Let’s say we have the following HTML:
<p id="myPara" class="intro">Hello World!</p>
We can change the content and style of the paragraph element using JavaScript:
var paragraph = document.getElementById('myPara');
paragraph.innerHTML = 'Goodbye World!';
paragraph.style.color = 'red';
Creating and Appending DOM Elements
In addition to manipulating existing elements, you can create new DOM elements and append them to the document. This allows you to dynamically generate content based on user interactions or data from an external source.
Example:
Let’s say we want to create a new paragraph element and append IT to the body of the document:
var newParagraph = document.createElement('p');
newParagraph.innerHTML = 'This is a new paragraph!';
document.body.appendChild(newParagraph);
Conclusion
JavaScript DOM manipulation is a fundamental skill for web developers. By understanding how to select, manipulate, and create DOM elements, you can create dynamic and interactive web pages that respond to user input and provide a rich user experience.
FAQs
Q: What is the difference between the DOM and HTML?
A: HTML is the markup language used to create the structure of a web page, while the DOM is the programming interface for accessing and manipulating that structure using JavaScript.
Q: Can I use external libraries for DOM manipulation?
A: Yes, there are several popular libraries and frameworks, such as jQuery and React, that provide additional features and convenience methods for DOM manipulation.
Q: How can I optimize my DOM manipulation for performance?
A: To optimize performance, minimize the number of DOM manipulations, use efficient selectors, and consider batching multiple changes together to minimize reflows and repaints.