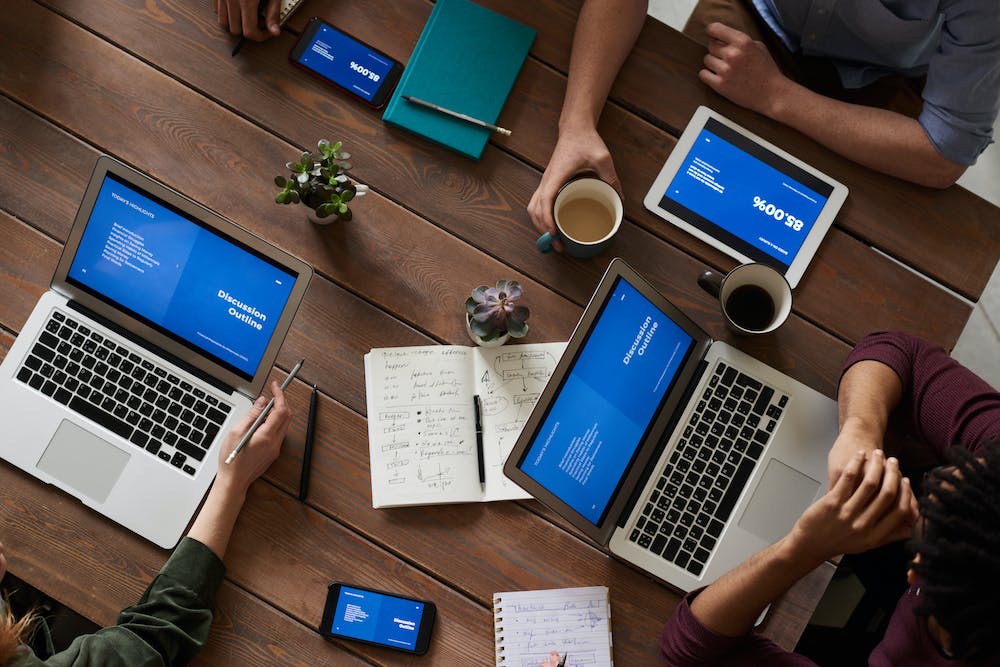
JavaScript is a powerful and versatile language that is widely used in web development. However, writing efficient and maintainable code in JavaScript can be challenging, especially for beginners. In this article, we will discuss some best practices, tips, and tricks for writing efficient JavaScript code to help you improve your coding skills and build better web applications.
Use Strict Mode
One of the best practices in JavaScript is to use strict mode. Strict mode is a special mode that you can enable in JavaScript to catch common coding errors and make the code more secure. IT helps you to write cleaner and more secure code by preventing certain actions and encouraging better coding practices. To enable strict mode in your JavaScript code, simply add the following line at the beginning of your script:
"use strict";
Using strict mode is a good practice that can help you catch errors early and make your code more robust.
Avoid Global Variables
Global variables can cause naming conflicts and make your code harder to maintain. It is a best practice to avoid using global variables in your JavaScript code. Instead, use local variables and encapsulate your code in functions to reduce the risk of naming conflicts and improve the modularity of your code.
Use Descriptive Variable Names
It is important to use descriptive variable names in your JavaScript code to improve code readability and maintainability. Instead of using generic names like “x” or “y”, use descriptive names that reflect the purpose of the variable. For example, instead of using “var a = 10;”, use “var numberOfItems = 10;”. This makes it easier for other developers (or even yourself in the future) to understand the code and make changes without introducing bugs.
Minimize Global Scope Pollution
Global scope pollution occurs when too many variables are declared in the global scope, increasing the risk of naming conflicts and making the code more difficult to manage. To minimize global scope pollution, encapsulate your code in functions and use closures to control the visibility of variables. This practice can help you keep your code organized and prevent unexpected behavior.
Avoid Using eval()
The eval() function in JavaScript can execute code in the form of a string, but it is considered a security risk and should be avoided. Using eval() can introduce security vulnerabilities and make the code harder to debug. Instead of using eval(), consider refactoring your code to use other programming constructs such as functions or objects to achieve the same result.
Optimize Loops and Iterations
Loops and iterations are common in JavaScript code, and optimizing them can greatly improve the performance of your web applications. Use efficient looping techniques, such as caching the length of arrays in for loops and avoiding nested loops whenever possible, to make your code more efficient and reduce the time complexity of your algorithms.
Use Built-in Functions and Methods
JavaScript provides many built-in functions and methods that you can use to perform common operations, such as array manipulation, string manipulation, and mathematical calculations. Using built-in functions and methods can make your code more concise and easier to read, while also benefiting from the performance optimizations implemented in these functions by the browser’s JavaScript engine.
Handle Errors Gracefully
Errors are an inevitable part of software development, and handling them gracefully is an important aspect of writing efficient and robust JavaScript code. Use try-catch blocks to handle exceptions and prevent them from crashing your application. Additionally, consider using custom error messages and logging to help you diagnose and fix errors more quickly.
Use Object-Oriented Programming (OOP) Principles
Object-oriented programming is a powerful paradigm that can help you write more organized and manageable code in JavaScript. Use OOP principles, such as encapsulation, inheritance, and polymorphism, to structure your code in a more modular and scalable way. This can make your code easier to understand and maintain, especially as your project grows in size and complexity.
Optimize DOM Manipulation
Manipulating the Document Object Model (DOM) is a common task in web development, but it can also be a performance bottleneck if not done efficiently. Minimize DOM manipulations by caching elements, using document fragments, and batch updating the DOM to reduce the overhead of reflow and repaint operations. This can greatly improve the performance of your web applications and create a smoother user experience.
Conclusion
JavaScript is a versatile language that enables you to build powerful and interactive web applications. By following these best practices, tips, and tricks for writing efficient JavaScript code, you can improve the performance, maintainability, and security of your applications. Remember to use strict mode, avoid global variables, use descriptive variable names, and handle errors gracefully to write cleaner and more maintainable code. By optimizing loops and iterations, using built-in functions, and leveraging OOP principles, you can make your code more efficient and scalable. Finally, be mindful of DOM manipulation and avoid using eval() to ensure the security and performance of your web applications.
FAQs
Q: What are some common performance bottlenecks in JavaScript?
A: Common performance bottlenecks in JavaScript include inefficient loops and iterations, excessive DOM manipulations, and unnecessary global variables. By optimizing these aspects of your code, you can greatly improve the performance of your web applications.
Q: How can I improve the security of my JavaScript code?
A: To improve the security of your JavaScript code, avoid using eval(), handle errors gracefully, and sanitize user input to prevent common vulnerabilities such as cross-site scripting (XSS) and injection attacks.
Q: What are the benefits of using strict mode in JavaScript?
A: Strict mode helps catch common coding errors, makes the code more secure, and prevents certain actions that might lead to unexpected behavior. It encourages better coding practices and can help you write cleaner and more robust code.
Q: How can I optimize DOM manipulations in JavaScript?
A: To optimize DOM manipulations in JavaScript, minimize the number of DOM operations, use document fragments for batch updates, and cache elements to reduce reflow and repaint operations. This can greatly improve the performance of your web applications.
Q: What is the importance of using descriptive variable names in JavaScript?
A: Using descriptive variable names improves code readability and maintainability, making it easier for other developers to understand the code and make changes without introducing bugs. It is an important best practice for writing clean and maintainable JavaScript code.