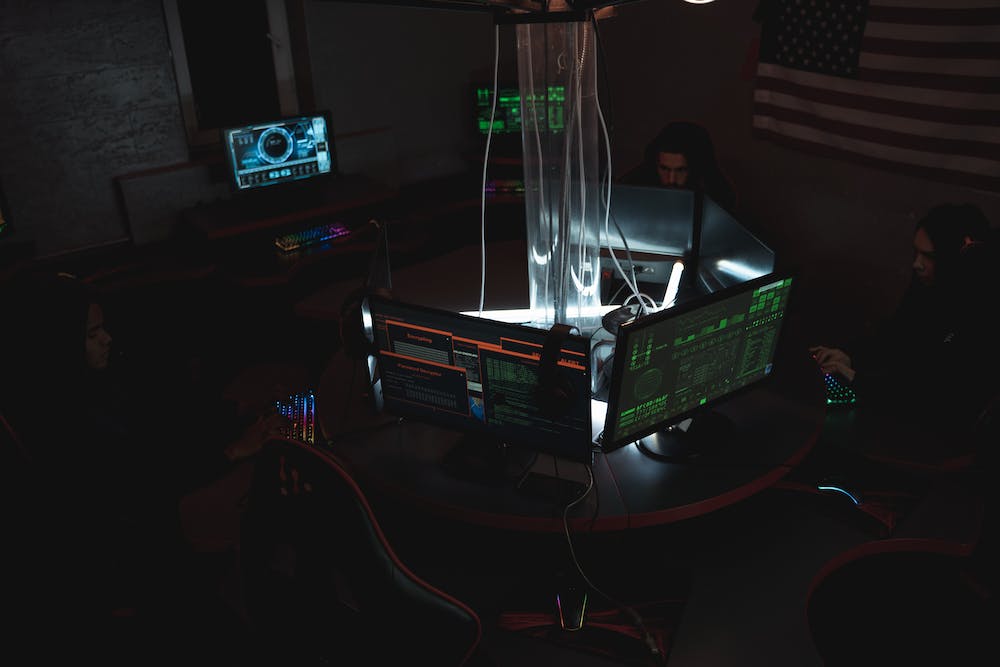
The fetch_assoc() method in PHP is a valuable tool for retrieving results from a MySQL database in an associative array format. This method allows developers to easily access data using key-value pairs, making IT a popular choice for many PHP projects. In this article, we will explore the fetch_assoc() method in detail, discussing its usage, benefits, and potential drawbacks.
Usage:
The fetch_assoc() method is primarily used in conjunction with the mysqli extension in PHP. IT is employed after executing a query to retrieve the result set from a MySQL database. This method returns the next row from the result set as an associative array, or NULL if there are no more rows.
To use fetch_assoc(), you must first establish a connection to the database and execute a query using the mysqli_query() function. Once the query is executed, you can iterate through the result set using a loop, calling fetch_assoc() within each iteration to retrieve the next row. Here is an example to illustrate its basic usage:
// Establish a connection to the database
$conn = mysqli_connect("localhost", "username", "password", "database");
// Execute a query
$result = mysqli_query($conn, "SELECT * FROM users");
// Iterate through the result set
while ($row = mysqli_fetch_assoc($result)) {
// Access the data using key-value pairs
echo "Name: " . $row["name"] . ", Email: " . $row["email"];
}
// Close the connection
mysqli_close($conn);
Benefits:
One of the main benefits of using fetch_assoc() is that IT returns the result set in an associative array format. This means that instead of accessing data using numerical indices, as with fetch_row(), you can use more descriptive column names as keys. This enhances code readability and makes IT easier to understand the structure of the result set.
Another advantage is that fetch_assoc() automatically fetches the next row from the result set, eliminating the need for manual iteration or keeping track of row indices. This simplifies code logic and reduces the risk of errors.
Furthermore, fetch_assoc() provides the flexibility to access specific columns of interest, disregarding the unused columns. This can significantly improve performance when working with large result sets, as IT avoids unnecessary data retrieval and processing.
Drawbacks:
While the fetch_assoc() method is convenient for many scenarios, IT is important to be aware of its limitations. One major drawback is that IT fetches the entire result set into memory. This can be problematic when dealing with large datasets, as IT consumes significant memory resources. In such cases, alternative methods like fetch_row() or fetch_array() may be more appropriate, as they fetch data row by row, reducing memory usage.
Another potential drawback is related to the key naming convention used in the associative array. By default, fetch_assoc() converts column names to lowercase. This can lead to issues if your database column names are case-sensitive, as data retrieval may fail. To avoid this, consider using aliases in your SQL queries, using lowercase names that match the column names in the database.
FAQs:
Q: Is fetch_assoc() limited to a specific version of PHP?
A: No, fetch_assoc() is supported in all PHP versions that include the mysqli extension. IT is backward compatible and can be used with PHP 5.x and above.
Q: Can fetch_assoc() retrieve data from multiple tables?
A: Yes, fetch_assoc() can handle queries involving multiple tables. IT returns all columns from the selected tables as an associative array, allowing you to access the data using their respective key-value pairs.
Q: Can I modify the key naming convention used by fetch_assoc()?
A: Unfortunately, the key naming convention of fetch_assoc() cannot be directly modified. However, you can use aliases in your SQL queries to specify custom names for the selected columns. These custom names will be reflected in the associative array returned by fetch_assoc().
In conclusion, the fetch_assoc() method in PHP is a powerful tool for retrieving database results in an associative array format. Its simplicity, code readability, and flexibility make IT a popular choice for many developers working with MySQL databases. By understanding its usage, benefits, and potential drawbacks, you can utilize this method effectively in your PHP projects.