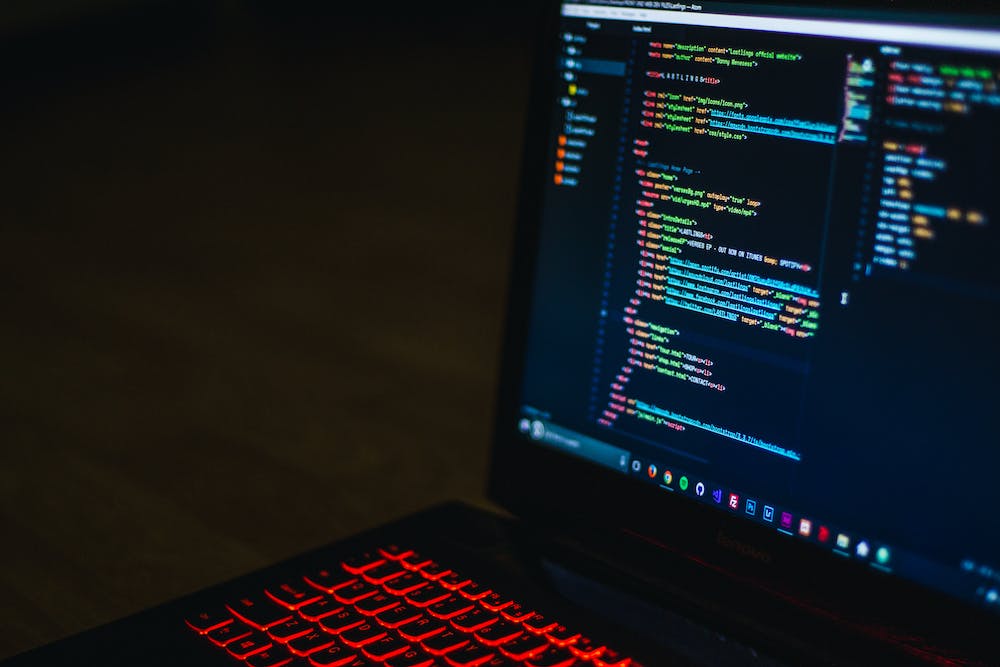
Python is a versatile programming language that is not only popular among seasoned developers but is also increasingly being embraced by beginners. Its simplicity and readability make IT an ideal choice for those looking to get started in the world of coding. To further enhance the user experience, Python offers a wide range of Graphical User Interface (GUI) frameworks that allow developers to create interactive and visually appealing applications. In this article, we will introduce you to the basics of Python GUI programming and provide you with a beginner’s guide to get started.
Choosing a GUI Framework
Python offers several GUI frameworks to choose from, each with its own set of features and advantages. Some of the most popular ones are:
- Tkinter: Tkinter is the standard GUI toolkit for Python and comes pre-installed with the language. IT provides a simple way to create windows, dialogs, buttons, and other elements.
- PyQt: PyQt is a set of Python bindings for the Qt toolkit, which is widely used for creating cross-platform applications. IT offers a comprehensive set of tools and is known for its robustness and flexibility.
- PySide: PySide is another set of Python bindings for the Qt toolkit. IT is similar to PyQt but is released under the LGPL license, making IT a preferred choice for commercial applications.
- wxPython: wxPython is a Python wrapper for the wxWidgets toolkit. IT provides native look and feel on various platforms and offers a wide range of customizable widgets.
These frameworks differ in terms of complexity, ease of use, and flexibility. Depending on your requirements and familiarity with a particular toolkit, you can choose the one that suits your needs.
Basic Concepts of GUI Programming
Before diving into GUI programming, IT is essential to understand some basic concepts. Let’s explore a few:
- Widgets: Widgets are the building blocks of a GUI application. They represent the various elements like buttons, labels, text fields, etc., that users interact with.
- Event-driven programming: GUI programming is primarily event-driven, meaning that the flow of the program is determined by user actions or events. For example, clicking a button or entering text in a text field triggers specific actions.
- Layout managers: Layout managers determine how widgets are arranged within a GUI window. They provide flexibility in designing the user interface and dynamically adjust the positions and sizes of widgets when the window is resized.
Understanding these concepts will help you create intuitive and user-friendly applications.
Getting Started with Tkinter
Tkinter is an excellent choice for beginners due to its simplicity and ease of use. Let’s take a look at a basic example to understand how to create a window using Tkinter:
import tkinter as tk
# Create a GUI window
window = tk.Tk()
window.title("My First GUI Application")
window.geometry("400x300")
# Add widgets
label = tk.Label(window, text="Hello, World!")
label.pack()
# Run the event loop
window.mainloop()
In this example, we import the Tkinter module under the name ‘tk’, create a window object, set its title and dimensions, and add a label widget displaying the text “Hello, World!”. Finally, we start the event loop using the ‘mainloop()’ method, which keeps the window open until the user closes IT.
Frequently Asked Questions (FAQs)
Q: Is Python suitable for GUI programming?
Yes, Python is suitable for GUI programming. IT offers several GUI frameworks like Tkinter, PyQt, PySide, and wxPython to create interactive and visually appealing applications.
Q: Which GUI framework should I choose as a beginner?
As a beginner, Tkinter is a recommended choice for GUI programming in Python due to its simplicity and ease of use. IT comes pre-installed with Python, making IT readily accessible.
Q: Can I create cross-platform GUI applications using Python?
Yes, several GUI frameworks available in Python, such as PyQt and PySide, allow you to create cross-platform applications. These frameworks provide native look and feel on different operating systems.
Q: Is GUI programming in Python only limited to desktop applications?
No, GUI programming in Python is not limited to desktop applications. With frameworks like Kivy and Pygame, which are tailored for graphical applications, you can develop GUI-based games and mobile applications.
Q: How can I handle user interactions in GUI programming?
In GUI programming, user interactions are handled through events. Each GUI framework provides methods or callbacks that are triggered when specific events occur, such as button clicks, mouse movements, or keystrokes.
Overall, Python GUI programming opens up a world of possibilities for developers looking to create attractive and interactive applications. Whether you are a beginner or an experienced coder, exploring GUI frameworks in Python can significantly enhance your programming skills and enable you to build intuitive user interfaces.