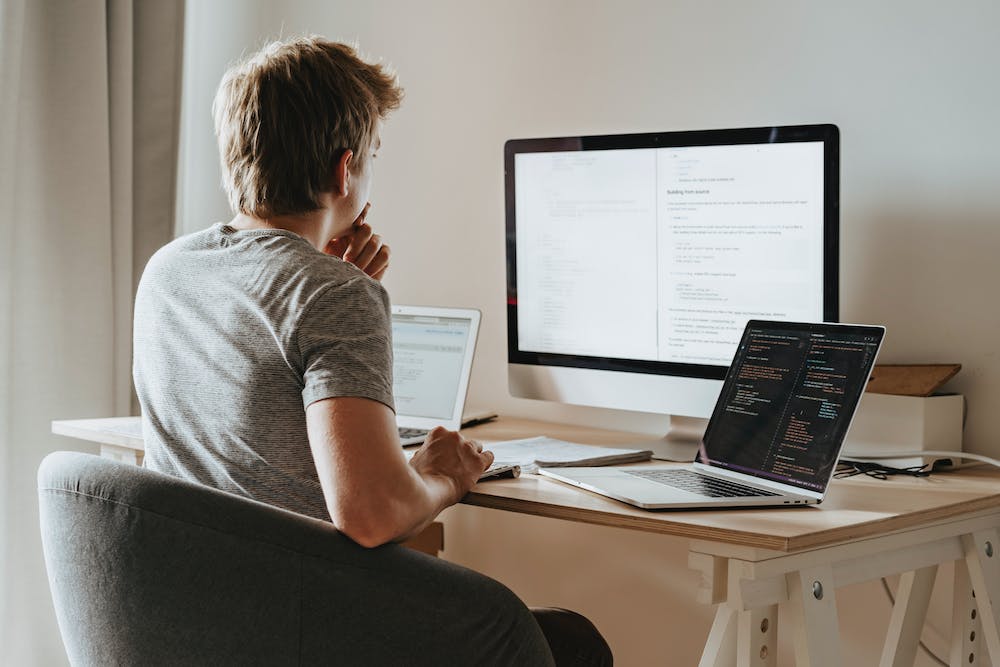
Node.js is a popular runtime environment that allows you to build scalable and high-performance applications using JavaScript. However, as your Node.js codebase grows in complexity, IT can become difficult to maintain and scale. That’s where design patterns come in.
Design patterns are proven solutions to recurring problems in software design. They provide a structured way to approach common challenges and help you create scalable and maintainable code. In this article, we will introduce you to Node.js design patterns and show you how to get started with writing code that is both scalable and maintainable.
Before diving into design patterns, let’s first understand the importance of writing scalable and maintainable code in Node.js. Scalable code can handle a growing number of requests and users without sacrificing performance. IT allows your application to handle increased traffic and workload without crashing or slowing down. Maintainable code, on the other hand, is easy to understand, modify, and debug. IT reduces the time and effort required to make changes or fix bugs in your code, ensuring that your application remains stable and reliable.
Now that we have established the importance of writing scalable and maintainable code, let’s explore some popular design patterns in Node.js:
1. Singleton Pattern: The Singleton pattern ensures that only one instance of a class is created throughout the lifetime of an application. IT is useful when you want to share stateful data across different parts of your codebase. For example, if you have a database connection that needs to be shared by multiple modules, you can use the Singleton pattern to ensure that there is only one instance of the database connection.
2. Factory Pattern: The Factory pattern is used to create objects without exposing the logic of their creation. IT allows you to separate the object creation process from the rest of your code, making IT easier to add or modify different types of objects in the future. For example, if you have a data access layer that needs to create different types of database connections based on the configuration, you can use the Factory pattern to encapsulate the creation logic.
3. Observer Pattern: The Observer pattern is used when you want to establish a one-to-many relationship between objects. IT allows one object to notify a list of observers whenever its state changes. This pattern is commonly used for event-driven programming in Node.js, where events are emitted by one module and listened to by multiple modules. For example, if you have a logging module that needs to be notified whenever an error occurs in your application, you can use the Observer pattern to establish this relationship.
4. Middleware Pattern: The Middleware pattern is a popular pattern in Node.js for handling request-response cycles. IT allows you to define a series of functions that are executed sequentially for each request. Each function has access to the request and response objects, and can modify them or perform actions before passing them to the next function. This pattern is commonly used for tasks such as authentication, logging, and error handling.
These are just a few examples of design patterns that can help you write scalable and maintainable code in Node.js. By understanding and applying these patterns, you can improve the structure, efficiency, and maintainability of your codebase.
FAQs
Q: Are design patterns specific to Node.js?
A: No, design patterns are not specific to Node.js. They are general solutions to common problems in software design that can be applied to any programming language or platform. However, some design patterns may be more commonly used in specific contexts or frameworks.
Q: How do I decide which design pattern to use in my Node.js application?
A: The choice of design pattern depends on the specific problem you are trying to solve and the requirements of your application. IT is important to understand the problem and the trade-offs of each design pattern before choosing one. You can also analyze existing codebases or seek advice from experienced developers to make an informed decision.
Q: Can I use multiple design patterns in the same Node.js application?
A: Yes, IT is common to use multiple design patterns in the same application. Different parts of your codebase may require different patterns to achieve scalability and maintainability. However, using too many patterns can make your codebase more complex, so IT is important to strike a balance and choose patterns that best suit your needs.
Q: Can design patterns be applied retrospectively to an existing Node.js codebase?
A: Yes, design patterns can be applied retrospectively to an existing codebase. However, IT may involve refactoring or redesigning certain parts of the code. IT is important to carefully analyze the impact of applying a design pattern and consider the trade-offs before making changes to an existing codebase.
Q: Where can I learn more about Node.js design patterns?
A: There are many resources available online to learn more about Node.js design patterns. Some recommended books on the topic include “Node.js Design Patterns” by Mario Casciaro and “Mastering Node.js Design Patterns” by Mario Casciaro and Luciano Mammino. You can also find tutorials, articles, and code examples on websites and online communities dedicated to Node.js development.