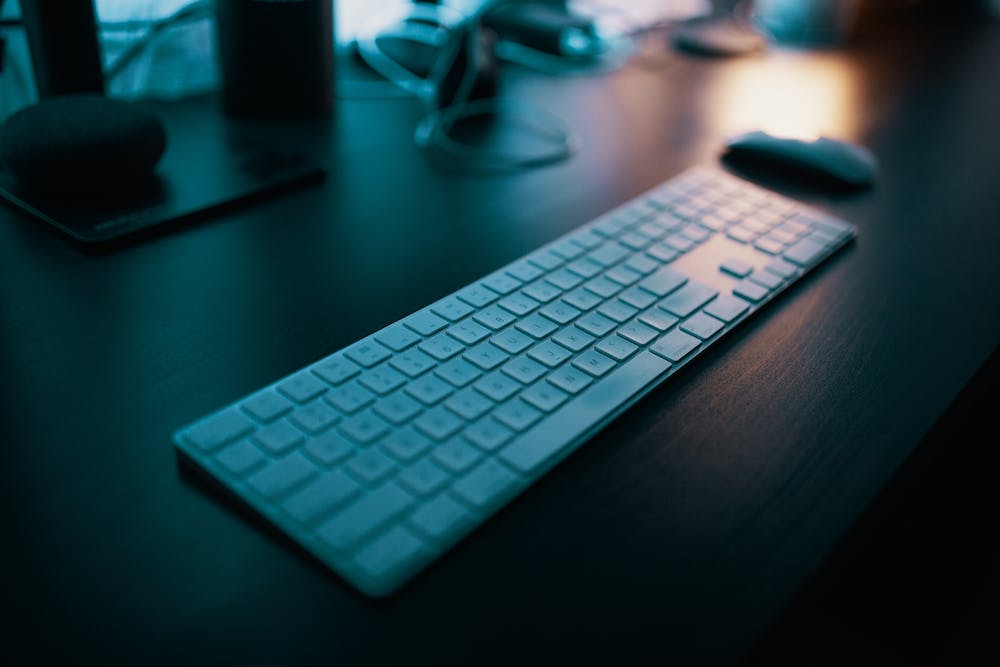
forEach is a built-in method in JavaScript that allows you to iterate over an array and perform a specific action for each element. IT provides a simple and concise way to loop through array elements without the need for a traditional for loop. In this comprehensive guide, we will explore the usage and syntax of forEach, discuss its benefits, and provide examples of its application in real-world scenarios.
Syntax:
array.forEach(function(currentValue, index, array) {
// code to be executed for each element
});
The forEach method takes a callback function as its parameter, which is executed for each element in the array. The callback function can have up to three parameters:
- currentValue: The current element being processed in the array.
- index: The index of the current element being processed.
- array: The array that the forEach method was called upon.
By utilizing these parameters, you can access and manipulate the elements of the array within the callback function.
Benefits of forEach:
There are several advantages to using forEach over traditional for loops:
- Readability: The forEach method provides a clear and concise way to iterate over an array, making the code easier to read and understand.
- Functional programming: forEach follows the principles of functional programming by applying a function to each element of the array, promoting code modularity and reusability.
- Avoiding common loop issues: Forgetting to initialize the loop variable, using incorrect termination conditions, or causing off-by-one errors are common pitfalls in traditional for loops. forEach eliminates these concerns.
Examples:
Let’s look at some examples to understand how forEach works:
// Example 1: Logging array elements
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
// Output: 1
// 2
// 3
// 4
// 5
In this example, forEach is used to log each element of the numbers array to the console.
// Example 2: Summing array elements
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
numbers.forEach(function(number) {
sum += number;
});
console.log(sum);
// Output: 15
In this example, forEach is used to calculate the sum of all elements in the numbers array.
FAQs:
Q: Can the forEach method be used with objects?
A: No, the forEach method can only be used with arrays. If you want to iterate over the properties of an object, you can use a for…in loop.
Q: Can the forEach method be used to stop or break the loop?
A: No, the forEach method will always iterate over all elements of the array. If you need to stop or break the loop based on a certain condition, you should use a traditional for loop or other loop construct that allows for early termination.
Q: Can the forEach method be used asynchronously?
A: Yes, the forEach method can be used with asynchronous operations. However, you need to handle the asynchronous behavior separately to ensure the desired outcome. You can use techniques such as Promises, async/await, or callbacks to manage asynchronous tasks within the forEach function.
Q: Can the forEach method modify the original array?
A: Yes, the forEach method can modify the original array. Since the callback function has access to the current element and the array itself, you can modify array elements or add/remove elements within the forEach loop.
Conclusion:
The forEach method in JavaScript provides a simple and effective way to iterate over array elements. With its clean syntax and array-specific functionality, IT enhances code readability and promotes functional programming principles. By understanding the usage, benefits, and examples of forEach, you can leverage its power to simplify your array manipulation tasks.