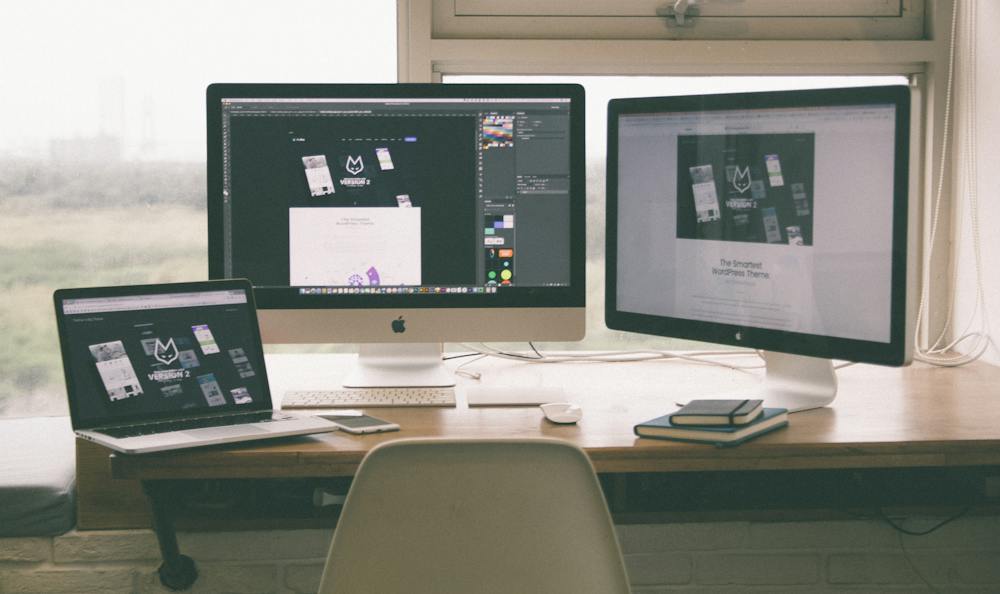
Lighweight Directory Access Protocol (LDAP) is a widely used protocol for accessing and maintaining distributed directory information services. IT provides a centralized way to manage user authentication and authorization in an organization. Many companies use LDAP as a means of centralizing user accounts and permissions across different systems and applications.
PHP is a popular server-side scripting language that is often used to build web applications. In this article, we will explore how to implement LDAP authentication in PHP applications, allowing users to authenticate against an LDAP server to access the application.
Prerequisites
Before diving into LDAP authentication, there are a few prerequisites that need to be in place:
- An LDAP server to authenticate against
- PHP installed on the server
- The LDAP PHP extension enabled
Connecting to the LDAP Server
The first step in implementing LDAP authentication in a PHP application is to establish a connection with the LDAP server. This can be done using the ldap_connect
function provided by the LDAP PHP extension:
$ldapServer = 'ldap.example.com';
$ldapPort = 389;
$ldapConnection = ldap_connect($ldapServer, $ldapPort);
if (!$ldapConnection) {
die('Unable to connect to LDAP server');
}
Once the connection is established, the next step is to bind to the LDAP server using a set of credentials. There are two ways to bind to the LDAP server: anonymously or with a specific user’s credentials.
Here is an example of binding to the LDAP server using a specific user’s credentials:
$ldapUsername = 'uid=user,ou=people,dc=example,dc=com';
$ldapPassword = 'password';
$bind = ldap_bind($ldapConnection, $ldapUsername, $ldapPassword);
if (!$bind) {
die('Unable to bind to LDAP server');
}
Authenticating Users
Once the connection to the LDAP server is established and the user is bound, the application can proceed with authenticating users. This typically involves searching the LDAP directory for a user’s entry and then verifying the user’s credentials.
Here is a basic example of authenticating a user against an LDAP server:
$searchFilter = '(uid=johndoe)';
$searchResult = ldap_search($ldapConnection, 'ou=people,dc=example,dc=com', $searchFilter);
$entry = ldap_first_entry($ldapConnection, $searchResult);
if ($entry) {
$userDn = ldap_get_dn($ldapConnection, $entry);
$userBind = ldap_bind($ldapConnection, $userDn, $password);
if ($userBind) {
echo 'User authenticated successfully';
} else {
echo 'Invalid credentials';
}
} else {
echo 'User not found';
}
This example demonstrates how to search for a user with the uid ‘johndoe’ in the LDAP directory and then authenticate the user using their distinguished name (DN) and password.
Handling Authentication Errors
It’s important to handle authentication errors gracefully in a PHP application. If the LDAP authentication fails, the application should provide meaningful feedback to the user.
For example, if the user provides invalid credentials, the application can display an appropriate error message:
if (!$userBind) {
echo 'Invalid credentials';
}
Similarly, if the user is not found in the LDAP directory, the application can display a message indicating that the user does not exist:
if (!$entry) {
echo 'User not found';
}
Conclusion
Implementing LDAP authentication in PHP applications provides a secure and centralized way to manage user authentication and authorization. By leveraging the LDAP PHP extension, developers can seamlessly integrate LDAP authentication into their applications, allowing users to authenticate against an LDAP server to access the application.
By following the steps outlined in this article, developers can ensure that their PHP applications are able to authenticate users against an LDAP directory, providing a seamless and secure user experience.
FAQs
1. What is LDAP?
LDAP stands for Lightweight Directory Access Protocol. It is a protocol for accessing and maintaining distributed directory information services over an IP network.
2. Is PHP a suitable language for implementing LDAP authentication?
Yes, PHP is a suitable language for implementing LDAP authentication. The LDAP PHP extension provides a comprehensive set of functions for working with LDAP directories.
3. Can LDAP authentication be used in conjunction with other authentication methods?
Yes, LDAP authentication can be used in conjunction with other authentication methods. Many applications support multiple authentication methods, allowing users to choose the one that best suits their needs.
4. Are there any security considerations when implementing LDAP authentication in PHP applications?
Yes, there are several security considerations to keep in mind when implementing LDAP authentication. It’s important to securely store and manage user credentials, and to properly handle authentication errors to prevent information disclosure.
5. Can LDAP authentication be used for single sign-on (SSO) purposes?
Yes, LDAP authentication can be used for single sign-on purposes. Many organizations use LDAP as a means of centralizing user accounts and permissions across different systems and applications, allowing users to authenticate once and access multiple resources.
6. Are there any best practices for implementing LDAP authentication in PHP applications?
Yes, there are several best practices for implementing LDAP authentication in PHP applications. These include securely storing and managing user credentials, properly handling authentication errors, and following industry standards and guidelines for LDAP integration.
7. How can I test LDAP authentication in a PHP application?
You can test LDAP authentication in a PHP application by setting up a local LDAP server or using a publicly available LDAP service for testing purposes. By following the steps outlined in this article, you can ensure that your PHP application is able to authenticate users against an LDAP directory.