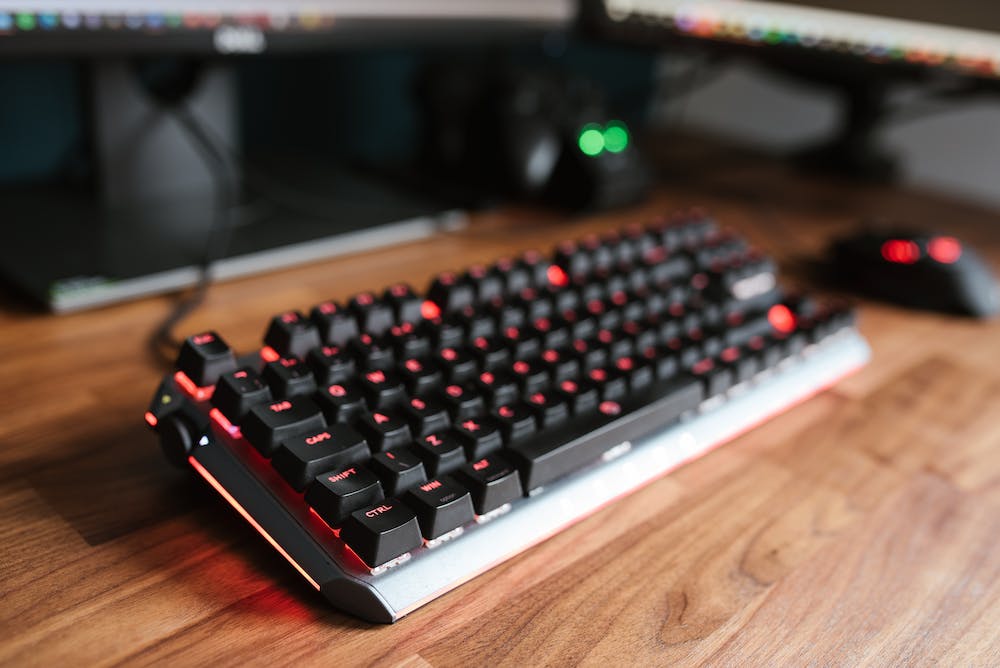
As a popular PHP framework, Laravel provides built-in features for implementing authentication and authorization in web applications. In this article, we will explore the process of implementing authentication and authorization in a Laravel application, along with best practices and examples.
Authentication in Laravel
Authentication is the process of verifying the identity of a user. In Laravel, authentication can be easily implemented using the built-in authentication scaffolding provided by the framework. The authentication scaffolding generates the necessary routes, controllers, and views for user authentication.
To implement authentication in Laravel, you can use the following command to generate the necessary files:
php artisan make:auth
This command will create the authentication scaffolding including routes, controllers, and views for user registration, login, and password reset. Once the authentication scaffolding is generated, users can register, log in, and log out of the application.
User Model and Database Migration
Laravel’s authentication scaffolding also includes a User model and database migration for the users table. The User model represents the user entity in the application and provides methods for user authentication and authorization. The database migration creates the users table with the necessary columns for storing user information such as name, email, and password.
After generating the authentication scaffolding, you can run the database migration using the following command:
php artisan migrate
This command will create the users table in the database, allowing users to be stored and managed in the application.
Authorization in Laravel
Authorization is the process of determining whether a user has the necessary permissions to perform a specific action. In Laravel, authorization can be implemented using middleware and gates. Middleware allows you to filter HTTP requests entering your application, while gates allow you to define granular authorization logic.
Middleware
Laravel provides a set of middleware for authentication and authorization. The auth
middleware can be used to restrict access to authenticated users, while the guest
middleware can be used to restrict access to unauthenticated users.
For example, to restrict access to a route to authenticated users, you can apply the auth
middleware as follows:
Route::get('/dashboard', 'DashboardController@index')->middleware('auth');
By applying the auth
middleware to the dashboard route, only authenticated users will be able to access the dashboard page. This helps to ensure that sensitive areas of the application are only accessible to authorized users.
Gates
In addition to middleware, Laravel provides gates for defining authorization logic within the application. Gates allow you to define fine-grained access control by specifying conditions that must be met for a user to perform a specific action.
For example, you can define a gate to check if a user has the role of administrator as follows:
Gate::define('admin', function ($user) {
return $user->role === 'administrator';
});
Once the gate is defined, you can use the gate to check if a user has the necessary permission to perform an action within the application. This helps to ensure that users are only able to perform actions that they are authorized to carry out.
Best Practices
When implementing authentication and authorization in a Laravel application, IT is important to follow best practices to ensure the security and integrity of the application. Some best practices for implementing authentication and authorization in Laravel include:
- Using strong and unique passwords for user accounts.
- Implementing two-factor authentication for an added layer of security.
- Regularly hashing and updating user passwords.
- Implementing role-based access control to assign specific permissions to users.
- Using HTTPS to encrypt data transmitted between the client and server.
By following these best practices, you can enhance the security of your Laravel application and mitigate the risk of unauthorized access and data breaches.
Conclusion
Implementing authentication and authorization in a Laravel application is essential for ensuring the security and integrity of the application. With the built-in features provided by Laravel, developers can easily implement authentication and authorization, and follow best practices to enhance the security of their applications.
FAQs
Q: What is the difference between authentication and authorization?
A: Authentication is the process of verifying the identity of a user, while authorization is the process of determining whether a user has the necessary permissions to perform a specific action.
Q: Can I customize the authentication and authorization logic in Laravel?
A: Yes, Laravel provides flexibility for customizing authentication and authorization logic using middleware and gates.
Q: What are some best practices for implementing authentication and authorization in Laravel?
A: Some best practices include using strong and unique passwords, implementing two-factor authentication, regularly hashing and updating passwords, implementing role-based access control, and using HTTPS to encrypt data.
Q: Are there any security risks associated with implementing authentication and authorization in Laravel?
A: While Laravel provides built-in features for authentication and authorization, developers should still follow best practices to mitigate security risks such as unauthorized access and data breaches.