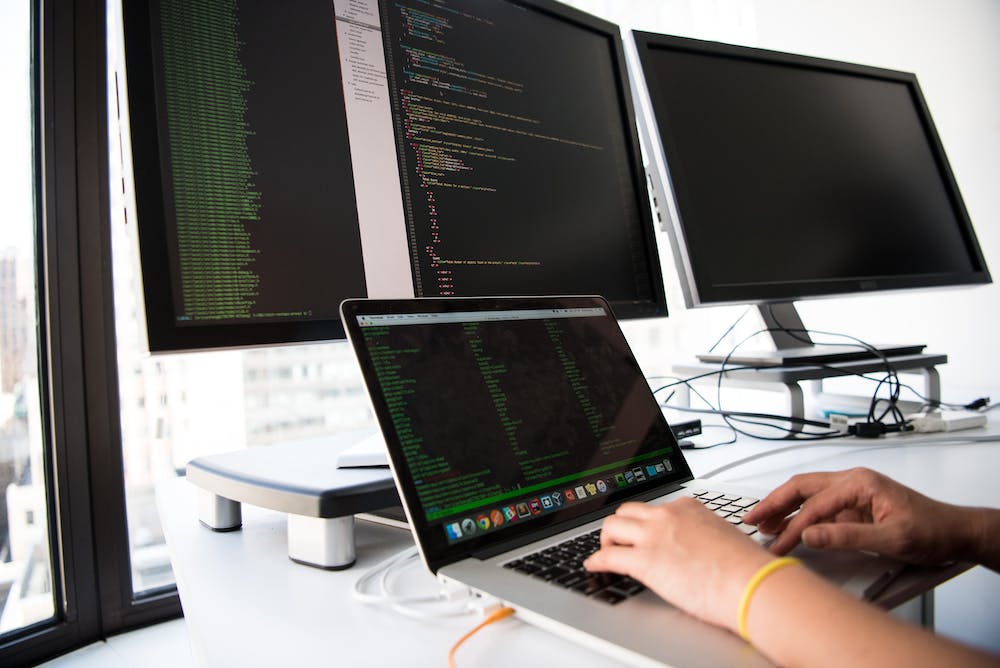
Search functionality is a crucial component of any web application, and implementing advanced search features can greatly enhance the user experience. In this article, we will discuss the best practices and techniques for implementing advanced search features in PHP.
Understanding Advanced Search Features
Before we delve into the best practices and techniques for implementing advanced search features, IT is important to understand what advanced search features are and why they are important.
Advanced search features go beyond the basic search functionality by allowing users to refine their search queries using various filters and parameters. This can include date ranges, categories, price ranges, and more. Advanced search features provide users with more control over their search results, leading to a better user experience and increased engagement.
Best Practices for Implementing Advanced Search Features
When implementing advanced search features in PHP, there are several best practices to keep in mind to ensure a smooth and efficient search experience for users.
1. Use a Structured Data Model
Before implementing advanced search features, it is important to have a well-structured data model that allows for easy querying and filtering. This often involves using a database management system such as MySQL to organize and store the data.
By using a structured data model, you can easily retrieve and filter the data based on the user’s search parameters, resulting in faster and more accurate search results.
2. Implement Proper Indexing
Proper indexing of the database is crucial for efficient search functionality. By properly indexing the fields that are commonly used for searching and filtering, you can significantly improve the performance of the search queries.
For example, if you have a table of products and users commonly search for products based on their names, it is important to index the “product_name” field to speed up the search queries.
3. Utilize Full-Text Search
Full-text search is a powerful feature that allows users to search for specific words or phrases within a large body of text. In PHP, you can utilize the full-text search capabilities of databases such as MySQL to enable advanced text-based searching.
By implementing full-text search, you can provide users with a more comprehensive search experience, allowing them to easily find relevant content within large datasets.
4. Incorporate Dynamic Filtering Options
Dynamic filtering options allow users to refine their search results based on various parameters such as categories, price ranges, date ranges, and more. When implementing advanced search features, it is important to provide users with a range of dynamic filtering options to cater to their specific search needs.
For example, an e-commerce Website may offer dynamic filtering options for product categories, price ranges, sizes, colors, and more, allowing users to quickly narrow down their search results based on their preferences.
5. Implement Pagination for Large Result Sets
When dealing with large result sets, it is important to implement pagination to ensure that the search results are displayed in manageable chunks. Pagination allows users to navigate through multiple pages of search results, improving the overall user experience.
By implementing pagination, you can prevent overwhelming users with a large number of search results on a single page, making it easier for them to browse through the results and find what they are looking for.
Techniques for Implementing Advanced Search Features in PHP
Now that we have covered the best practices for implementing advanced search features, let’s explore some techniques for actually implementing these features in PHP.
1. Building a Dynamic Search Form
The first step in implementing advanced search features is to build a dynamic search form that allows users to input their search parameters. This can include input fields for keywords, dropdown menus for categories, price ranges, date ranges, and more.
Using PHP, you can dynamically generate the search form based on the available filtering options, allowing for a flexible and user-friendly search experience.
Here’s an example of a dynamic search form in PHP:
“`php
“`
2. Processing the Search Query
Once the user submits the search form, the search parameters are sent to a PHP script for processing. In this script, you can use the user-inputted parameters to construct a query that retrieves the relevant search results from the database.
For example, if a user enters keywords and selects a category, the PHP script can construct a SQL query that retrieves products matching the keywords within the specified category.
Here’s an example of processing a search query in PHP:
“`php
// Retrieve search parameters from the form
$keywords = $_GET[‘keywords’];
$category = $_GET[‘category’];
// Construct the SQL query based on the search parameters
$sql = “SELECT * FROM products WHERE product_name LIKE ‘%$keywords%’ AND category = ‘$category'”;
“`
3. Displaying the Search Results
After processing the search query and retrieving the relevant results from the database, the next step is to display the search results to the user. In PHP, you can iterate through the retrieved data and present it in a user-friendly format, such as a list of products with relevant details and links to view more information.
Here’s an example of displaying search results in PHP:
“`php
// Execute the query and fetch the results
$results = $db->query($sql);
// Display the search results
while ($row = $results->fetch_assoc()) {
echo “
echo “
” . $row[‘product_name’] . “
“;
echo “
” . $row[‘description’] . “
“;
echo “View Details“;
echo “
“;
}
“`
Conclusion
Implementing advanced search features in PHP can greatly enhance the user experience and improve the overall usability of a web application. By following the best practices and techniques outlined in this article, you can create a robust and efficient search functionality that provides users with more control over their search queries and delivers relevant and accurate results.
Remember to continuously test and optimize your search functionality to ensure that it meets the evolving needs of your users and provides a seamless search experience.
FAQs
Q: What are some common challenges when implementing advanced search features in PHP?
A: Some common challenges include optimizing search performance for large datasets, handling complex search queries, and ensuring that the search results are accurate and relevant to the user’s query.
Q: Is it necessary to use a database management system such as MySQL for implementing advanced search features?
A: While it is not strictly necessary, using a database management system can greatly simplify and streamline the process of implementing advanced search features, especially when dealing with structured data and complex search queries.
Q: How can I enhance the search functionality of my web application beyond basic keyword-based searching?
A: You can enhance the search functionality by implementing features such as dynamic filtering options, full-text search, faceted search, and natural language processing to provide users with a more comprehensive and intuitive search experience.