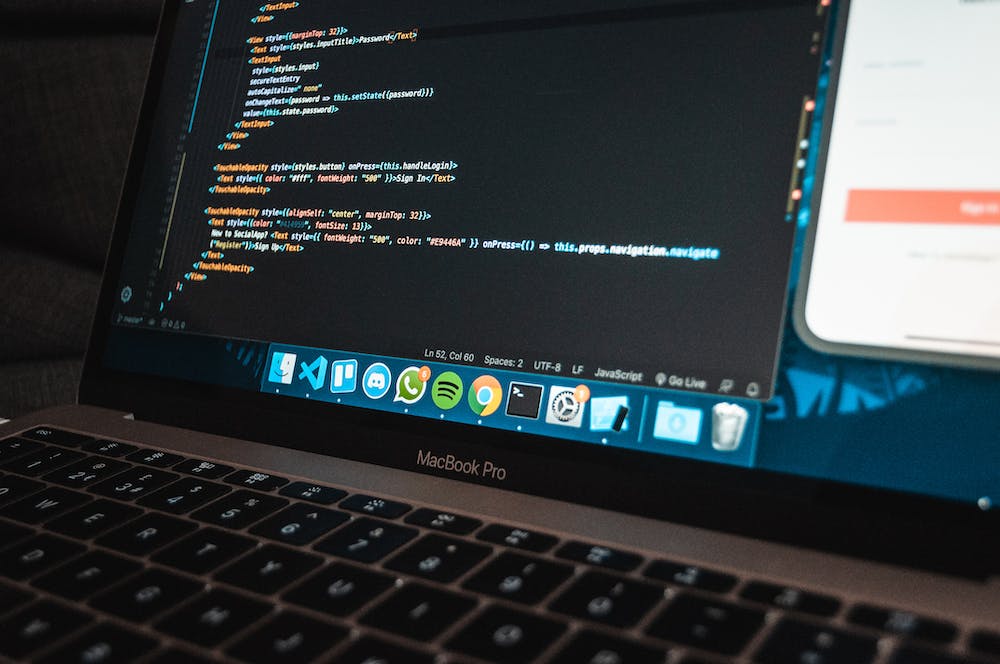
Implementing Advanced Filtering in Laravel: A Comprehensive Guide
Introduction:
Filtering large datasets is a common task in web development, and Laravel provides a robust framework for implementing advanced filtering. In this comprehensive guide, we will explore the various techniques and features that Laravel offers to create powerful filters for your application. Whether you are working on an e-commerce Website or a data visualisation platform, this guide will provide you with the knowledge to implement advanced filtering efficiently and effectively.
Getting Started:
Before diving into the advanced filtering techniques, let’s start by understanding the basic principles of filtering in Laravel. Laravel provides a Query Builder class that allows you to build SQL queries programmatically. This class provides various methods such as where, orWhere, and whereIn, which can be used to specify conditions for filtering the dataset.
Filtering by Single Field:
In most cases, you will need to filter your dataset based on one or more fields. Let’s start by considering a simple scenario where we have a “users” table with fields such as name, email, and role. To filter the dataset based on the “name” field, we can use the where method of the Query Builder as follows:
$users = DB::table('users')
->where('name', 'John')
->get();
This query will retrieve all the users whose name is “John” from the “users” table. Similarly, you can filter the dataset based on other fields by replacing ‘name’ with the desired field name.
Filtering by Multiple Fields:
Filtering by a single field is useful, but often we need to filter the dataset based on multiple fields. Laravel provides the where function, which accepts an array of conditions as its first argument. Each element in the array should be an array with three elements: the column name, the operator, and the value. Let’s consider an example:
$users = DB::table('users')
->where([
['name', '=', 'John'],
['role', '=', 'admin']
])
->get();
This query will retrieve all the users whose name is “John” and role is “admin” from the “users” table.
Filtering with Dynamic Conditions:
Often, the filter conditions need to be dynamic based on user input or other factors. Laravel provides the when method, which allows you to conditionally apply filters. The when method accepts two arguments: a boolean value and a closure. If the boolean value is true, the closure will be executed, and the filters inside the closure will be applied. Otherwise, the filters will be ignored. Here’s an example:
$name = 'John';
$users = DB::table('users')
->when($name, function ($query, $name) {
return $query->where('name', '=', $name);
})
->get();
In this example, if the $name variable is not null or empty, the filter on the “name” field will be applied. Otherwise, the filter will be ignored, and all users will be retrieved.
Advanced Filtering with Eloquent:
Laravel’s Eloquent ORM provides a convenient and expressive way to work with databases. IT also includes advanced filtering features that can simplify your code and make IT more readable. Let’s consider an example:
$users = User::query()
->where('name', 'John')
->orWhere('role', 'admin')
->orderBy('created_at', 'desc')
->get();
This example demonstrates how easy IT is to construct complex filters using the Eloquent ORM. We can chain multiple methods to build the desired query.
FAQs:
1. Can I use advanced filtering with pagination?
Yes, you can use advanced filtering techniques in combination with Laravel’s pagination feature. Instead of calling the get method at the end of your query, you can use the paginate method to retrieve paginated results.
2. Can I filter on relationships?
Yes, Laravel’s Eloquent ORM allows you to filter based on relationships between models. You can use the whereHas and orWhereHas methods to apply filters on related models.
3. How can I apply filters based on date ranges?
Laravel provides the whereBetween method, which can be used to apply filters based on date ranges. You can specify the start and end dates as arguments to this method.
Conclusion:
In this comprehensive guide, we have explored various techniques for implementing advanced filtering in Laravel. From basic filtering by single or multiple fields to dynamic conditions and advanced filtering with the Eloquent ORM, Laravel offers a powerful set of tools to handle complex filtering requirements. By leveraging these features, you can create robust and efficient filters for your Laravel applications.