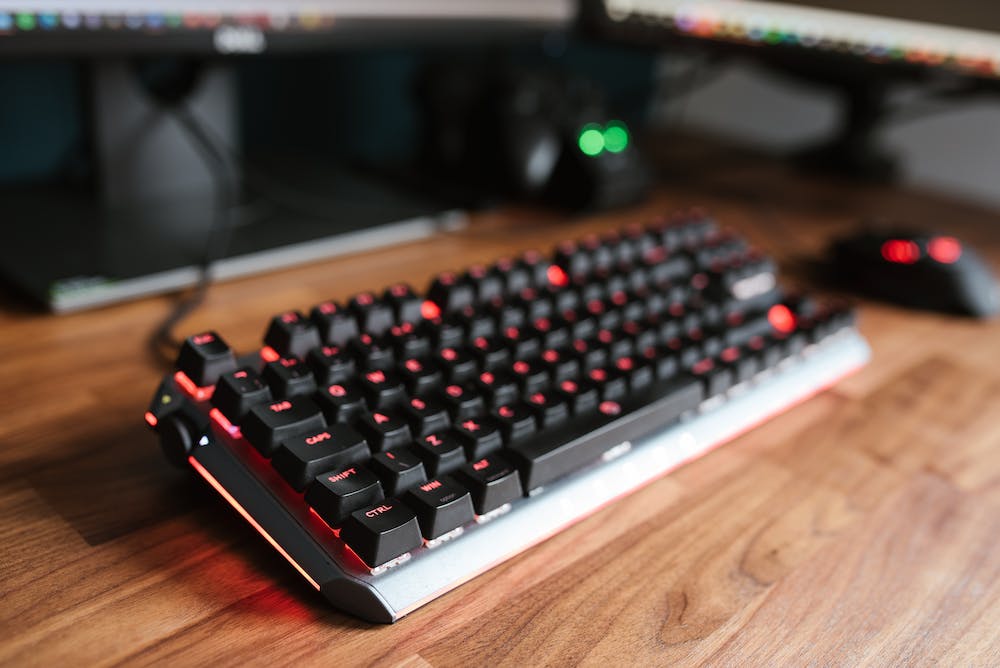
PHP is one of the most popular programming languages for web development. IT powers a large part of the internet, but it also has a reputation for being insecure if not used properly. In this article, we will discuss best practices for writing secure PHP scripts to ensure that your code is not vulnerable to common security threats.
1. Keep PHP Updated
One of the most important things you can do to ensure the security of your PHP scripts is to keep your PHP version updated. PHP releases regular updates with security patches to fix vulnerabilities. By keeping your PHP version up to date, you can ensure that you are protected against known security threats.
2. Sanitize User Input
Unsanitized user input is one of the most common causes of security vulnerabilities in PHP scripts. Always validate and sanitize user input before using it in your scripts to prevent SQL injection, cross-site scripting (XSS), and other security issues. Use functions like htmlspecialchars() and mysqli_real_escape_string() to sanitize input and prevent attacks.
3. Use Prepared Statements
When working with databases in PHP, use prepared statements instead of directly embedding user input in SQL queries. Prepared statements ensure that user input is treated as data, not as part of the SQL query, which prevents SQL injection attacks.
4. Avoid Using Eval
The eval() function in PHP allows you to execute arbitrary PHP code stored in a string, but it is a major security risk. Avoid using eval() whenever possible, as it can lead to code injection and other security issues.
5. Validate File Uploads
If your PHP script allows file uploads, make sure to validate the file type and size before accepting the upload. Use functions like getimagesize() to check the file type and move_uploaded_file() to store the file in a secure location. Never trust the file extension provided by the user; always validate the file contents.
6. Use HTTPS for Secure Communication
When your PHP script communicates with the server or transmits sensitive data, use HTTPS to encrypt the communication. This prevents eavesdropping and man-in-the-middle attacks and ensures the security of your data.
7. Limit Error Reporting
While debugging your PHP scripts, it’s common to display error messages, but this can expose sensitive information to potential attackers. Set the error_reporting directive in your PHP configuration to limit the amount of error information displayed to users and log errors instead.
8. Secure Session Management
When using sessions in PHP, make sure to use secure session management practices. Use session_regenerate_id() to regenerate the session ID to prevent session fixation attacks, and set the session cookie to be secure and HttpOnly to prevent session hijacking and cross-site scripting attacks.
9. Use Secure Authentication
When implementing user authentication in your PHP scripts, use secure authentication methods such as bcrypt for password hashing and two-factor authentication for added security. Avoid storing passwords in plain text and use strong password policies to protect user accounts.
10. Regular Security Audits
Regularly audit your PHP scripts for security vulnerabilities using tools like PHP Security Scanner and conducting code reviews. Look for common security issues and vulnerabilities in your code and take steps to address them to ensure the security of your applications.
Conclusion
Writing secure PHP scripts is essential to protect your applications and users from potential security threats. By following best practices such as keeping PHP updated, sanitizing user input, using prepared statements, and implementing secure authentication, you can ensure that your PHP scripts are not vulnerable to common security issues.
FAQs
Q: What is the importance of keeping PHP updated?
A: Keeping PHP updated is essential to ensure that your scripts are protected against known security vulnerabilities. PHP releases regular updates with security patches to fix vulnerabilities and it’s important to stay up to date with the latest version.
Q: What are some common security vulnerabilities in PHP scripts?
A: Some common security vulnerabilities in PHP scripts include SQL injection, cross-site scripting (XSS), file upload vulnerabilities, and insecure session management. By following best practices for secure PHP scripting, you can mitigate these vulnerabilities.
Q: How can I ensure the security of user authentication in my PHP scripts?
A: Use secure authentication methods such as bcrypt for password hashing, implement two-factor authentication for added security, and ensure that passwords are not stored in plain text. By following secure authentication practices, you can protect user accounts from potential security threats.