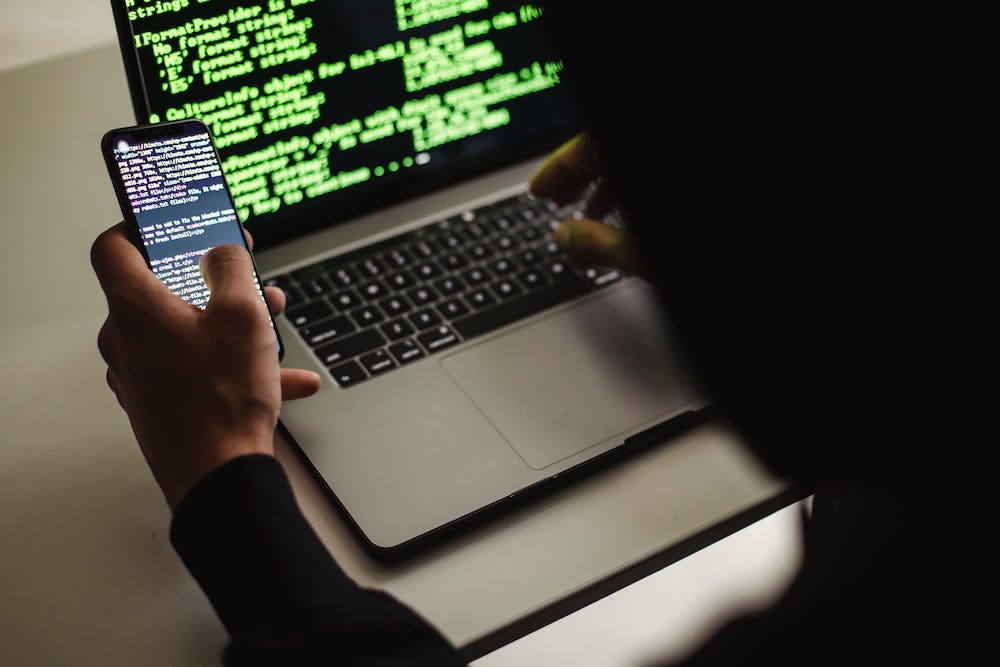
Palindromes are words, phrases, or sequences that remain the same when read backward. For instance, “racecar” and “level” are palindromes. In this article, we will explore how to write a palindrome program in Python. With the help of this program, you can easily determine if a given word or phrase is a palindrome or not. Additionally, we will cover some frequently asked questions related to palindromes and their applications.
writing a Palindrome Program in Python
To write a palindrome program in Python, we can follow a simple approach. Here, we will compare the original string with its reverse counterpart. If both are equal, then the input is a palindrome; otherwise, IT is not. Let’s look at an example implementation:
def is_palindrome(word):
return word == word[::-1]
user_input = input("Enter a word or phrase: ")
if is_palindrome(user_input):
print(user_input + " is a palindrome.")
else:
print(user_input + " is not a palindrome.")
In this program, we define a function called is_palindrome
that takes a word or phrase as an input parameter. The function applies string slicing using the [::-1]
notation to reverse the input. IT then compares the reversed string with the original one and returns True
if they are the same, indicating a palindrome. Otherwise, IT returns False
.
Finally, we prompt the user to enter a word or phrase using the input()
function. We pass the user’s input to the is_palindrome
function and display the appropriate message based on the returned value.
Frequently Asked Questions
1. What is a palindrome?
A palindrome is a word, phrase, number, or sequence that reads the same forward as IT does backward. Examples include “deed,” “madam,” and “1221.”
2. How does the palindrome program work?
The palindrome program works by comparing the input string with its reverse counterpart. If both are the same, the input is considered a palindrome. Otherwise, IT is not.
3. Can the palindrome program handle phrases with spaces?
Yes, the palindrome program can handle phrases with spaces. IT treats the entire input as a single string and checks palindromic properties accordingly.
4. Is the palindrome check case-sensitive?
Yes, the palindrome check is case-sensitive. For example, “Racecar” and “racecar” would be considered different words in terms of palindromes.
5. Can the palindrome program handle numbers?
Yes, the palindrome program can handle numbers as input. IT treats them as strings and checks for palindromic properties accordingly.