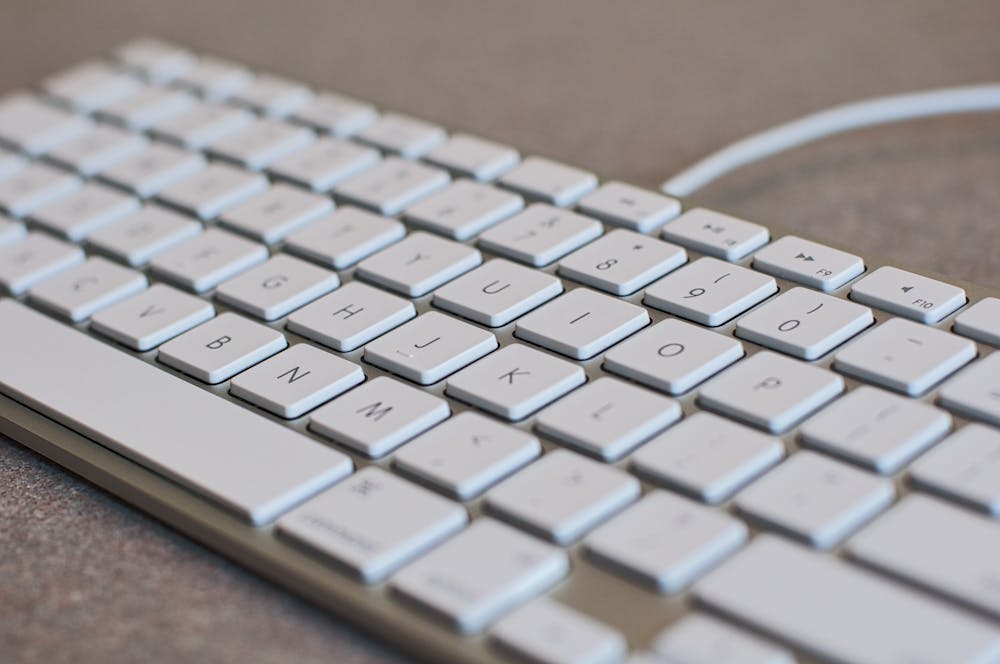
PHP is a popular server-side scripting language that is widely used for web development. IT provides a number of built-in functions that make it easy to work with variables, arrays, and objects. One such function is unset
, which allows you to remove a variable or an array element.
In this tutorial, we will explore the usage of the unset
function in PHP. We will start with a brief introduction to the function and then move on to its syntax and examples. By the end of this tutorial, you will have a clear understanding of how to use the unset
function in your PHP code.
Introduction to the Unset Function
The unset
function in PHP is used to destroy the specified variables. This means that it removes the variable from the current scope, making it undefined. You can also use unset
to remove a specific element from an array or to unset one or more variables.
When you unset a variable, the memory associated with that variable is released, and the variable is no longer accessible. However, it’s important to note that unset
does not destroy the variable itself, but only the variable’s association with a value. You can still use the variable after unsetting it, but it will be treated as undefined.
The Syntax of the Unset Function
The syntax of the unset
function is straightforward. You simply use the keyword unset
followed by the variable name or array element you want to unset. Here is the basic syntax:
unset($variable_name);
unset($array_name[key]);
Where $variable_name
is the name of the variable you want to unset, and $array_name[key]
is the specific array element you want to remove.
It’s important to note that you can unset multiple variables or array elements at the same time by separating them with a comma within the unset
function.
Examples of Using the Unset Function
Let’s go through a few examples to demonstrate how the unset
function works in PHP.
Example 1: Unsetting a Variable
In this example, we will unset a variable and then try to access it to see how PHP handles undefined variables.
$variable = "Hello, world!";
unset($variable);
echo $variable; // This will throw an error since $variable is undefined
As you can see, attempting to access the unset variable will result in a notice or warning, depending on your PHP error reporting settings. This is because the variable is no longer defined after being unset.
Example 2: Unsetting an Array Element
Arrays are a fundamental part of PHP, and you can use the unset
function to remove specific elements from an array. Here’s an example:
$colors = array("red", "green", "blue");
unset($colors[1]); // Removes the element at index 1
print_r($colors); // Outputs Array([0] => red [2] => blue)
After unsetting the element at index 1, the array will be re-indexed, and the remaining elements will be shifted to fill the empty space.
Example 3: Unsetting Multiple Variables
You can also unset multiple variables in a single unset
statement. Here’s an example:
$var1 = "value1";
$var2 = "value2";
$var3 = "value3";
unset($var1, $var2, $var3);
In this example, all three variables are unset simultaneously.
Best Practices for Using the Unset Function
When working with the unset
function in PHP, there are a few best practices to keep in mind:
- Always check if a variable or array element exists before unsetting it. Trying to unset an undefined variable or array element will result in a notice or warning.
- Be mindful of the potential side effects of unsetting variables, especially in larger applications. Unsetting variables can make your code harder to read and maintain, so use it judiciously.
- Consider using
unset
to free up memory when working with large arrays or objects that are no longer needed. This can help improve the performance of your PHP code.
Conclusion
The unset
function in PHP is a powerful tool for removing variables and array elements from the current scope. By using unset
judiciously, you can free up memory and improve the performance of your PHP code. However, it’s important to be mindful of the potential side effects of unsetting variables and to use this function responsibly.
Thank you for reading this step-by-step tutorial on how to use the unset
function in PHP. We hope you found it helpful and informative.
FAQs
What happens when you unset a variable in PHP?
When you unset a variable in PHP, you remove its association with a value, making it undefined. The memory associated with that variable is released, but the variable itself is not destroyed. You can still use the variable, but it will be treated as undefined.
Can you unset multiple variables at the same time in PHP?
Yes, you can unset multiple variables in a single unset
statement by separating them with a comma.
Are there any potential side effects of unsetting variables in PHP?
Yes, unsetting variables can make your code harder to read and maintain, especially in larger applications. It’s important to use unset
judiciously and consider its potential side effects.