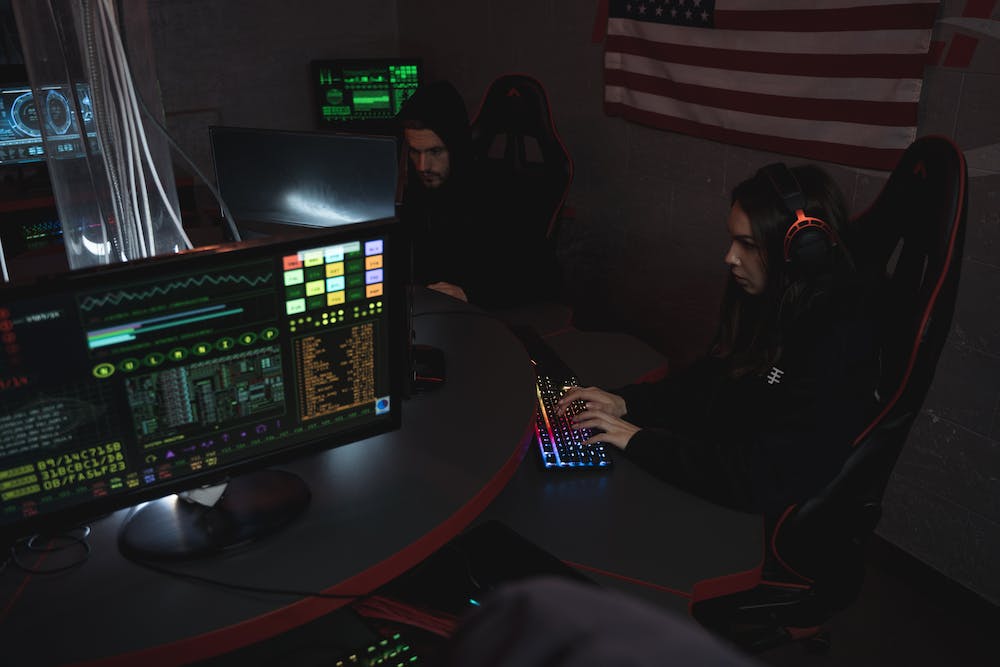
How to Use the PHP Array Filter Function
The PHP array_filter() function is a powerful tool that allows you to filter the elements of an array based on a given callback function. This function is particularly useful when you need to extract specific elements from an array based on certain criteria. In this article, we will explore how to use the array_filter() function effectively and provide some common use cases.
To use the array_filter() function, you need to have a basic understanding of PHP and how arrays work. If you are unfamiliar with these concepts, IT might be helpful to familiarize yourself with them before diving into this tutorial.
So, let’s get started with the basics of the array_filter() function.
Basic Syntax
The basic syntax of the array_filter() function is as follows:
array_filter(array, callback function);
The array
parameter represents the array you want to filter, while the callback function
is used to specify the criteria for filtering. The callback function takes each element of the array as its argument and returns either true
or false
based on the filtering criteria.
Let’s suppose we have an array of numbers, and we want to filter out all the even numbers. We can achieve this using the array_filter() function as shown below:
$numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
$filtered_numbers = array_filter($numbers, function($value){
return $value % 2 == 0;
});
In this example, the callback function checks whether each element in the array is divisible by 2. If the condition is satisfied, the element is included in the filtered array; otherwise, IT is excluded.
Now that you have a basic understanding of how the array_filter() function works, let’s explore some common use cases.
Filtering based on a specific key or value
You can use the array_filter() function to filter an array based on a specific key or value. Let’s say you have an associative array of students with their corresponding grades, and you want to filter out all the students who scored less than 80%.
$students = [
"John" => 90,
"Jane" => 75,
"Sarah" => 85,
"Michael" => 95,
"Emily" => 70
];
$filtered_students = array_filter($students, function($value){
return $value >= 80;
});
In this case, the callback function checks whether each grade is greater than or equal to 80. If IT is, the student name and corresponding grade are included in the filtered array.
Filtering based on multiple criteria
The array_filter() function also allows you to apply multiple criteria for filtering. Let’s consider an example where you have an array of products with their respective prices and availability status, and you want to filter out only the available products under a certain price range.
$products = [
["name" => "Phone", "price" => 500, "available" => true],
["name" => "Laptop", "price" => 1200, "available" => true],
["name" => "Tablet", "price" => 300, "available" => false],
["name" => "Headphones", "price" => 100, "available" => true],
["name" => "Camera", "price" => 800, "available" => false]
];
$filtered_products = array_filter($products, function($value){
return $value["price"] <= 500 && $value["available"] == true;
});
In this example, the callback function filters out the products with a price greater than 500 or with an availability status of false. Only the products that meet both criteria will be included in the filtered array.
FAQs:
Q: Can I modify the original array using array_filter()?
A: No, array_filter() function only filters the array and returns a new filtered array. The original array remains unchanged.
Q: Is IT possible to use array_filter() with multidimensional arrays?
A: Yes, you can use array_filter() with multidimensional arrays. The callback function should be adjusted to handle the specific structure of your array.
Q: Is IT mandatory to pass a callback function to array_filter()?
A: No, IT is not mandatory to pass a callback function to array_filter(). If you omit the callback function, array_filter() will remove all elements that are equal to false.
Q: Can I use a predefined function as the callback function?
A: Yes, you can use both predefined and user-defined functions as the callback function in array_filter(). However, keep in mind that the callback function should accept one argument and return a boolean value.
Q: What happens if the callback function returns null?
A: If the callback function returns null, the corresponding element will be excluded from the filtered array.