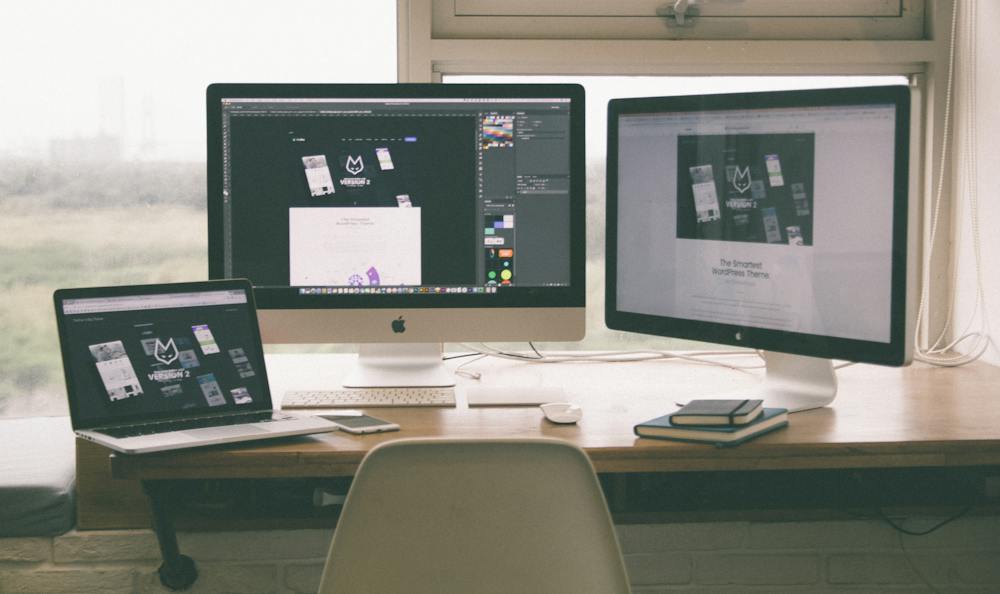
When working with PHP, IT‘s important to be able to determine whether a variable exists before attempting to use IT. One way to do this is by using the isset() function. In this article, we’ll take a look at how to use isset() to check for variable existence in PHP.
Using isset() to Check for Variable Existence
The isset() function in PHP is used to determine whether a variable is set and is not NULL. IT returns true if the variable exists and has a value other than NULL, and false otherwise. The syntax for using isset() is as follows:
if(isset($variable)){
// Do something if the variable exists
} else {
// Do something if the variable does not exist
}
Here’s a simple example of how to use isset() to check for the existence of a variable:
$name = "John Doe";
if(isset($name)){
echo "The variable 'name' exists";
} else {
echo "The variable 'name' does not exist";
}
In this example, the isset() function will return true since the variable $name has been set to a value.
Using isset() to Check for Array Element Existence
isset() can also be used to check for the existence of a specific element within an array. Here’s an example:
$fruits = array("apple", "banana", "orange");
if(isset($fruits[0])){
echo "The first element of the array exists";
} else {
echo "The first element of the array does not exist";
}
In this example, isset() will return true since the first element of the $fruits array exists.
Using isset() with Form Input
Another common use case for isset() is to check for the existence of form input. For example, if you have a form with a field named “username”, you can use isset() to check whether the user has submitted a value for that field:
if(isset($_POST['username'])){
// Process the form input
} else {
// Display an error message
}
In this example, the isset() function is used to check whether the “username” field has been submitted via the POST method.
Conclusion
As we’ve seen, the isset() function in PHP is a useful tool for checking the existence of variables, array elements, and form input. By using isset(), you can ensure that your code only accesses variables that have been properly initialized, which can help prevent errors and improve the overall reliability of your PHP applications.
FAQs
1. What is the difference between isset() and empty() in PHP?
The isset() function checks whether a variable is set and is not NULL, while the empty() function checks whether a variable is empty (i.e., IT does not exist, or IT has a value of 0, an empty string, or NULL). In general, isset() should be used to check for variable existence, while empty() is used to check whether a variable has a “falsy” value.
2. Can isset() be used with objects in PHP?
Yes, isset() can be used to check for the existence of object properties in PHP. For example, you can use isset() to check whether an object has a specific property before attempting to access IT.
3. Is IT necessary to use isset() for all variables in PHP?
While IT‘s not strictly necessary to use isset() for every variable in your PHP code, IT‘s generally a good practice to do so in order to ensure that your code is robust and error-free. Using isset() can help prevent undefined variable errors and make your code easier to understand and maintain.