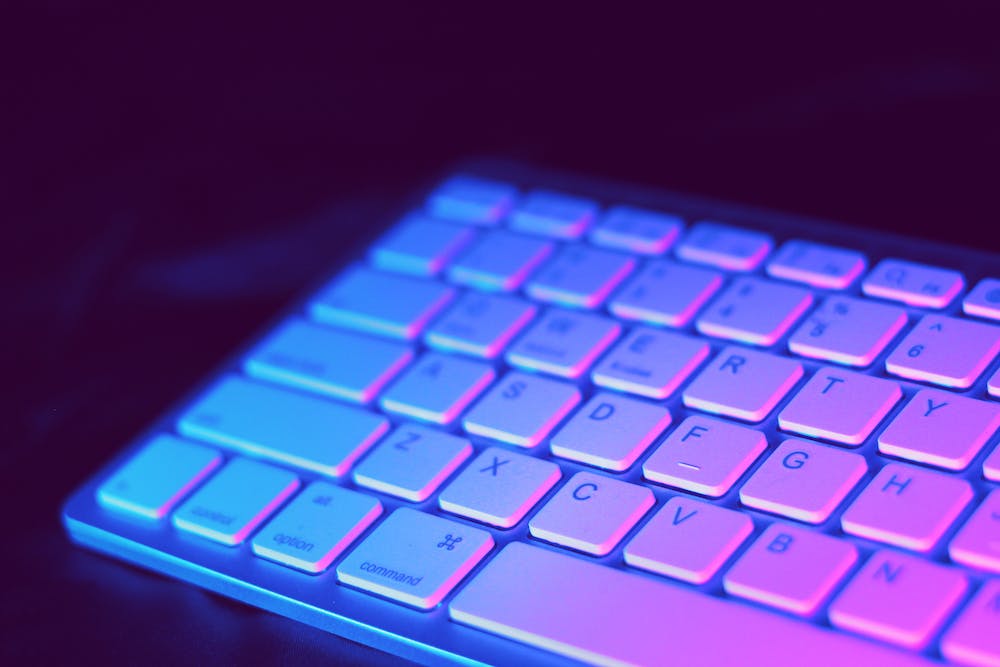
When IT comes to web development, data validation is a crucial aspect of ensuring that the input received from users is accurate and safe to use. In PHP, the ’empty()’ function is a powerful tool for validating data and ensuring that IT meets the required criteria. In this article, we will explore how to use the ’empty()’ function effectively for data validation in PHP.
Understanding the ’empty()’ Function
The ’empty()’ function in PHP is used to determine whether a variable is empty or not. IT returns true if the variable is empty, and false if IT is not. A variable is considered empty if IT does not exist or if its value equals false, 0, an empty string, an empty array, or null.
Here’s a simple example of how the ’empty()’ function works:
“`php
$name = “”;
if(empty($name)) {
echo “The variable is empty”;
} else {
echo “The variable is not empty”;
}
“`
In this example, the variable $name is assigned an empty string, so the ’empty()’ function returns true, and the message “The variable is empty” is displayed.
Using ’empty()’ for Data Validation
One of the most common use cases for the ’empty()’ function is data validation. When accepting user input, IT‘s essential to ensure that the data is not only present but also meets the required format or criteria. Here’s how you can use the ’empty()’ function for data validation:
1. Checking for Empty Input Fields
When processing form submissions, IT‘s crucial to check if the required fields are filled in. You can use the ’empty()’ function to determine if the input fields are empty and prompt the user to fill them in if necessary. Here’s an example:
“`php
if(empty($_POST[‘username’])) {
$errors[] = “Username is required”;
}
if(empty($_POST[‘password’])) {
$errors[] = “Password is required”;
}
“`
In this example, we use the ’empty()’ function to check if the username and password fields are empty. If they are, we add an error message to an array, indicating that the fields are required.
2. Validating Input Length
Another common use case for data validation is ensuring that the input meets the required length. You can combine the ’empty()’ function with the ‘strlen()’ function to check the length of a string. Here’s an example:
“`php
if(empty($_POST[‘username’]) || strlen($_POST[‘username’]) < 6) {
$errors[] = “Username must be at least 6 characters long”;
}
“`
In this example, we use the ’empty()’ function to check if the username is empty, and the ‘strlen()’ function to check if IT‘s at least 6 characters long. If the criteria are not met, we add an error message to the array.
3. Validating Email Addresses
Email validation is an essential aspect of data validation, especially when dealing with user registrations or contact forms. You can use the ’empty()’ function in combination with the ‘filter_var()’ function to validate email addresses. Here’s an example:
“`php
if(empty($_POST[’email’]) || !filter_var($_POST[’email’], FILTER_VALIDATE_EMAIL)) {
$errors[] = “Invalid email address”;
}
“`
In this example, we use the ’empty()’ function to check if the email field is empty, and the ‘filter_var()’ function with the FILTER_VALIDATE_EMAIL flag to validate the email address format. If the input is empty or not in the correct format, we add an error message to the array.
Conclusion
The ’empty()’ function in PHP is a valuable tool for effective data validation. By using IT in combination with other functions and conditions, you can ensure that the input received from users meets the required criteria and is safe to use in your applications. Whether you’re checking for empty input fields, validating input length, or verifying email addresses, the ’empty()’ function can help you enforce data validation rules and provide a seamless user experience.
FAQs
Q: Can the ’empty()’ function be used for validating numeric input?
A: Yes, the ’empty()’ function can be used to check if a numeric input is empty or equal to 0. However, for more advanced numeric validation, you may want to use other functions such as ‘is_numeric()’ or ‘filter_var()’ with the FILTER_VALIDATE_INT flag.
Q: Is the ’empty()’ function case-sensitive?
A: No, the ’empty()’ function is not case-sensitive. IT treats variables with the value of 0, “0”, or false as empty, regardless of their case.
Q: Are there any performance considerations when using the ’empty()’ function?
A: While the ’empty()’ function is a lightweight and fast way to check if a variable is empty, IT‘s essential to consider the overall performance of your application, especially when performing data validation on a large scale. Always test and benchmark your code to ensure optimal performance.
In conclusion, the ’empty()’ function in PHP is a versatile tool for data validation, allowing you to ensure that the input received from users meets the required criteria. By using IT in combination with other functions and conditions, you can enforce data validation rules and provide a seamless user experience in your web applications.