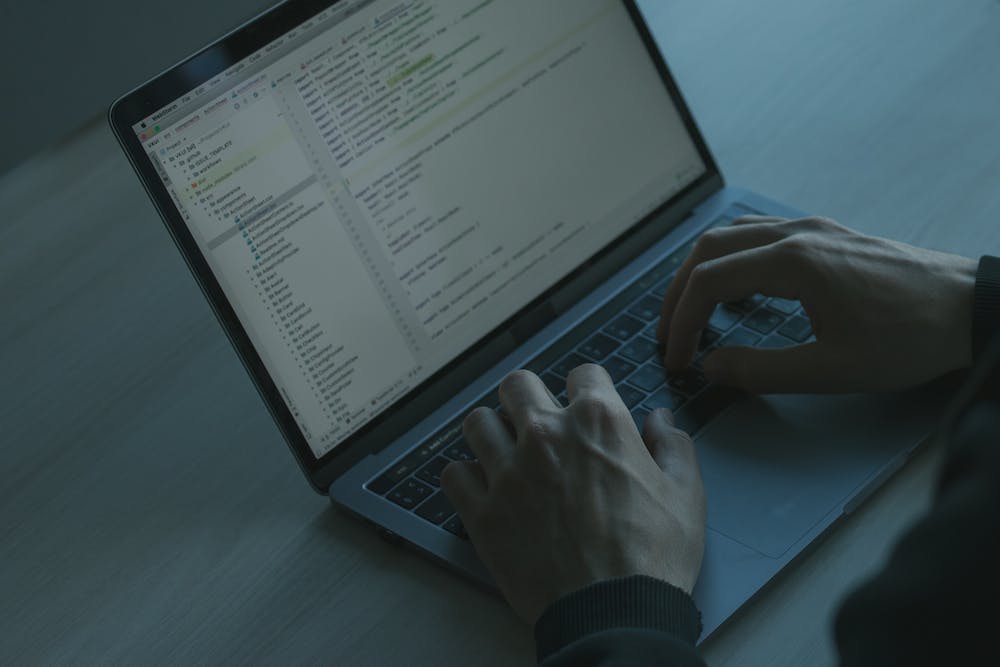
The PHP exec function allows developers to execute system commands directly from their PHP scripts. This can be useful in a variety of situations, such as running shell commands, executing command-line tools, and interacting with the operating system. In this article, we will dive deep into the PHP exec function, exploring its usage, best practices, and potential security concerns.
Before we begin, let’s cover the basic syntax and usage of the PHP exec function. The exec function takes a command as its parameter and executes IT, returning the last line of the command’s output. The command executes in a shell, allowing you to run complex commands with various options and arguments.
exec(command, output, return_var)
– command
: The command to be executed. IT can be a simple shell command or a complex one with full path and arguments.
– output
: An optional parameter that stores the output of the executed command. If specified, each line of the output will be added to the array.
– return_var
: Another optional parameter storing the exit status of the executed command. IT can be useful for error handling or checking if the command was executed successfully.
Now that we understand the basic syntax, let’s explore a few common use cases:
1. Running Basic Shell Commands:
The PHP exec function is often used to run simple shell commands. For example, we can execute the ‘ls’ command to list files and directories:
<\?php
exec('ls', $output);
print_r($output);
?>
The above code will print the output of the ‘ls’ command, which lists all files and directories in the current directory. IT can be modified to execute other shell commands as per your requirements.
2. Executing External Command-Line Tools:
The exec function allows us to execute external command-line tools, such as ImageMagick, FFmpeg, or Git. For example, let’s use the FFmpeg tool to convert a video file:
<\?php
$videoPath = '/path/to/video.mp4';
$outputPath = '/path/to/output.mp4';
exec("ffmpeg -i $videoPath -vcodec copy -acodec copy $outputPath");
?>
The above code takes an input video file, specified using the $videoPath
variable, and converts IT using FFmpeg. The converted output file is saved using the $outputPath
variable.
3. Security Considerations:
When using the PHP exec function, IT‘s important to consider security implications. Executing arbitrary commands can lead to command injection vulnerabilities if user input is not properly validated or sanitized. To mitigate this risk, IT‘s crucial to sanitize and validate any user-supplied input before using IT in a command.
Additionally, IT‘s advisable to use absolute paths for commands and their arguments, as relative paths can be manipulated by an attacker to execute arbitrary commands. Limit access permissions on the user executing the PHP scripts to prevent unauthorized execution of system commands.
Lastly, consider implementing appropriate input validation, output sanitization, and command whitelisting to further enhance the security of your PHP application.
FAQs:
Q1. Can I get the full output of the command?
A1. By default, the exec function only returns the last line of the command’s output. To capture the full output, you can pass an array as the second parameter to the exec function, like this:
<\?php
$output = [];
exec('command', $output);
print_r($output);
?>
Q2. How can I handle errors while executing commands?
A2. The last parameter of the exec function is used to capture the exit status of the executed command. A return value of 0 typically indicates success, while nonzero values indicate various error conditions. You can check this return value to handle errors appropriately:
<\?php
$returnValue = null;
exec('command', $output, $returnValue);
if ($returnValue !== 0) {
// Handle error
}
?>
Q3. Can I execute commands asynchronously?
A3. By default, the exec function executes commands synchronously, meaning IT waits for the command to finish before moving to the next line of code. If you need to execute commands asynchronously, you can use other PHP functions or libraries that provide such functionality, like proc_open
or pcntl_fork
.
In conclusion, the PHP exec function is a powerful tool for executing system commands from within PHP scripts. While IT offers great flexibility, IT‘s important to use IT with caution and consider security best practices to prevent potential vulnerabilities. By following the guidelines presented in this article, you can harness the power of the exec function while maintaining the security of your PHP applications.