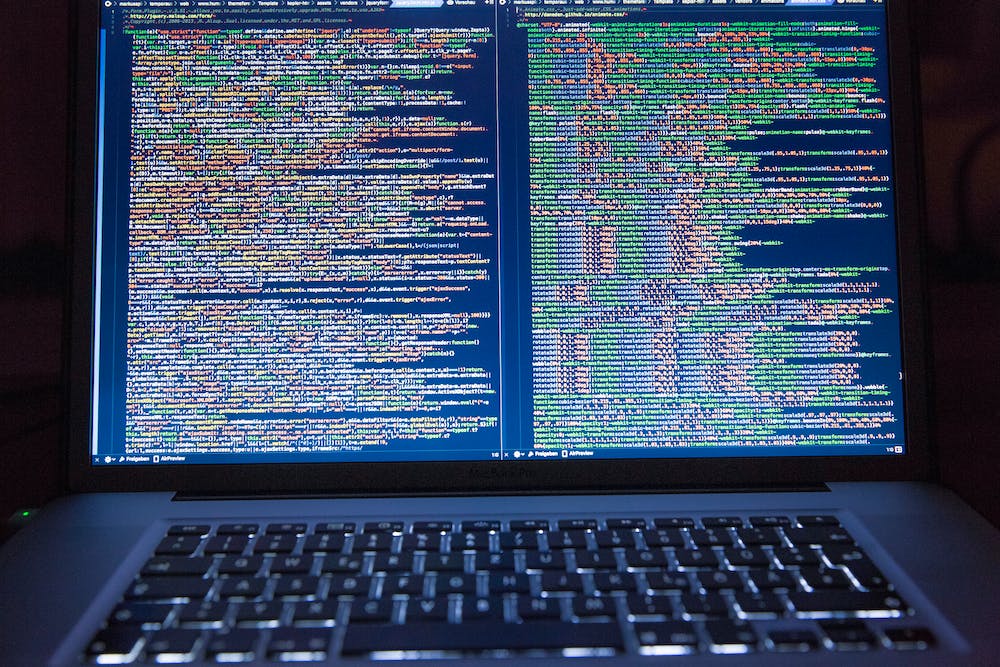
PHP is a powerful scripting language that is widely used for web development. One common task in PHP is to search and replace strings within a given text. The str_replace
function in PHP provides a simple and efficient way to achieve this. In this article, we will explore how to use str_replace
to search and replace strings in PHP.
Using str_replace
The str_replace
function in PHP is used to replace all occurrences of a search string with a replacement string in a given text. The basic syntax of the str_replace
function is as follows:
str_replace(search, replace, subject)
Where search
is the string to search for, replace
is the string to replace IT with, and subject
is the input string.
For example, consider the following PHP code:
$text = "Hello, World!";
$newText = str_replace("Hello", "Hi", $text);
echo $newText;
When this code is executed, IT will output:
Hi, World!
In this example, we used str_replace
to replace the word “Hello” with “Hi” in the input string $text
.
Using str_replace with Arrays
In addition to replacing a single string, the str_replace
function can also work with arrays of search and replace strings. The syntax for using str_replace
with arrays is as follows:
str_replace(search, replace, subject)
Where search
and replace
are arrays of search and replace strings, and subject
is the input string. For example:
$text = "The quick brown fox jumps over the lazy dog";
$search = ["quick", "brown", "fox", "lazy"];
$replace = ["slow", "black", "dog", "cat"];
$newText = str_replace($search, $replace, $text);
echo $newText;
When this code is executed, IT will output:
The slow black cat jumps over the cat dog
In this example, we used str_replace
with arrays of search and replace strings to replace multiple words in the input string $text
.
Case-Insensitive Replacement
The str_replace
function in PHP is case-sensitive by default. However, IT is possible to perform a case-insensitive replacement using the str_ireplace
function. The syntax of the str_ireplace
function is identical to that of str_replace
, but IT performs a case-insensitive search for the search string.
For example:
$text = "The quick brown fox jumps over the lazy dog";
$newText = str_ireplace("FOX", "cat", $text);
echo $newText;
When this code is executed, IT will output:
The quick brown cat jumps over the lazy dog
In this example, we used str_ireplace
to perform a case-insensitive replacement of the word “FOX” with “cat” in the input string $text
.
Conclusion
Using the str_replace
function in PHP provides a simple and efficient way to search and replace strings within a given text. Whether replacing a single word or multiple words, the str_replace
function can handle these tasks with ease. Additionally, the str_ireplace
function allows for case-insensitive replacements. By understanding and utilizing these functions, PHP developers can effectively manipulate strings in their applications.
FAQs
Q: Can str_replace
be used with variables?
A: Yes, the str_replace
function can be used with variables to perform dynamic replacements within a given text.
Q: What is the difference between str_replace
and str_ireplace
?
A: The str_replace
function is case-sensitive, while the str_ireplace
function is case-insensitive.
Q: Can str_replace
replace multiple occurrences of a search string?
A: Yes, the str_replace
function will replace all occurrences of the search string with the replacement string in the given text.