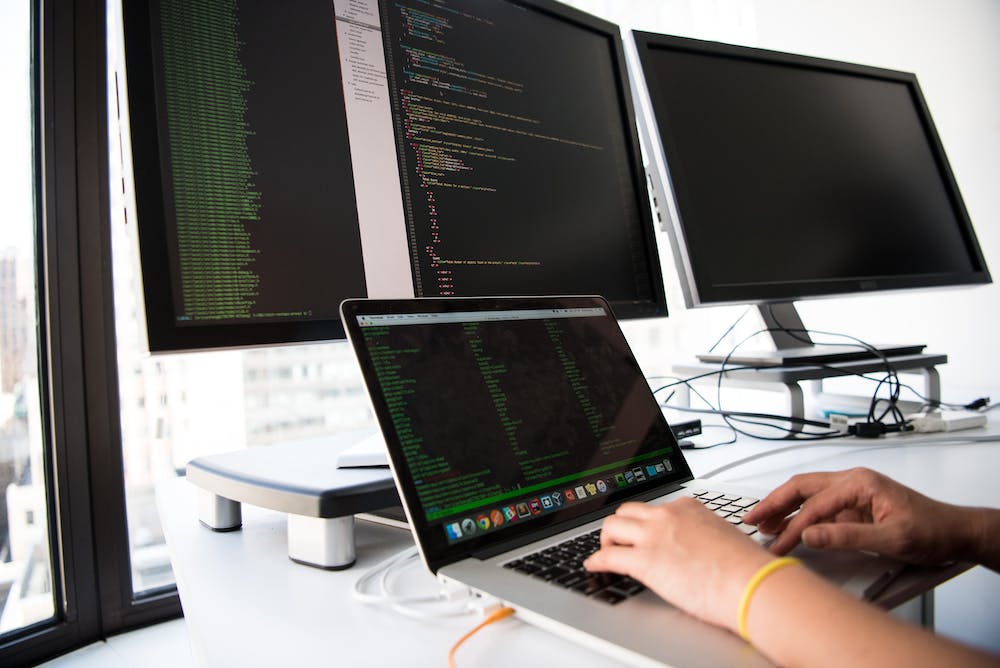
Microsoft Excel is a popular tool for creating and managing spreadsheets. IT is commonly used for data analysis, reporting, and visualization. In this article, we will explore how to read and write Excel files using PHP, a server-side scripting language widely used for web development.
Reading Excel Files Using PHP
PHP provides several libraries and extensions for reading and manipulating Excel files. One of the most commonly used is the PHPExcel library, which provides a rich set of features for working with Excel files. To read an Excel file using PHPExcel, you can use the following code:
require 'PHPExcel/IOFactory.php';
$objPHPExcel = PHPExcel_IOFactory::load('example.xlsx');
foreach ($objPHPExcel->getWorksheetIterator() as $worksheet) {
$worksheetTitle = $worksheet->getTitle();
$highestRow = $worksheet->getHighestRow();
$highestColumn = $worksheet->getHighestColumn();
for ($row = 1; $row <= $highestRow; $row++) {
$rowData = $worksheet->rangeToArray('A' . $row . ':' . $highestColumn . $row, null, true, false);
// Process $rowData
}
}
This code uses the PHPExcel library to load an Excel file named ‘example.xlsx’ and iterate through each worksheet to read the data. You can then process the data as needed, such as storing it in a database or displaying it on a web page.
writing Excel Files Using PHP
Similarly, PHP provides libraries and extensions for creating and writing Excel files. PHPExcel can also be used for this purpose. To create and write to an Excel file using PHPExcel, you can use the following code:
require 'PHPExcel.php';
$objPHPExcel = new PHPExcel();
$objPHPExcel->setActiveSheetIndex(0);
$objPHPExcel->getActiveSheet()->setCellValue('A1', 'Hello');
$objPHPExcel->getActiveSheet()->setCellValue('B1', 'World');
$objWriter = PHPExcel_IOFactory::createWriter($objPHPExcel, 'Excel2007');
$objWriter->save('hello_world.xlsx');
This code creates a new Excel file named ‘hello_world.xlsx’ and writes the text ‘Hello’ and ‘World’ into cells A1 and B1, respectively. You can add more data and formatting as needed to create more complex Excel files.
Working with Excel Files Using Other PHP Libraries
Aside from PHPExcel, there are other libraries and extensions that can be used to read and write Excel files using PHP. Some of these include PHPSpreadsheet, Spout, and SimpleExcel. Each of these libraries has its own set of features and capabilities, so it’s worth exploring them to find the one that best suits your needs.
Conclusion
Reading and writing Excel files using PHP can be a valuable skill for web developers, as it allows for the integration of spreadsheet data into web applications. Whether it’s importing data from Excel files into a database or generating Excel reports from web application data, PHP provides the necessary tools and libraries to work with Excel files effectively.
FAQs
Q: Can PHP read and write Excel files in other formats such as CSV or XLS?
A: Yes, PHP libraries and extensions such as PHPExcel and PHPSpreadsheet support reading and writing Excel files in various formats including CSV and XLS.
Q: Can PHP handle large Excel files efficiently?
A: PHP libraries such as PHPExcel and PHPSpreadsheet have optimizations for handling large Excel files, allowing for efficient processing of data without excessive memory usage.
Q: Are there any limitations to reading and writing Excel files using PHP?
A: While PHP libraries provide comprehensive features for working with Excel files, there may be limitations in terms of complex formulas, macros, and advanced formatting options. It’s important to consider these limitations when deciding to use PHP for Excel file manipulation.
References
1. PHPExcel Library Documentation: https://github.com/PHPOffice/PHPExcel
2. PHPSpreadsheet Library Documentation: https://github.com/PHPOffice/PhpSpreadsheet
3. Spout Library Documentation: https://github.com/box/spout