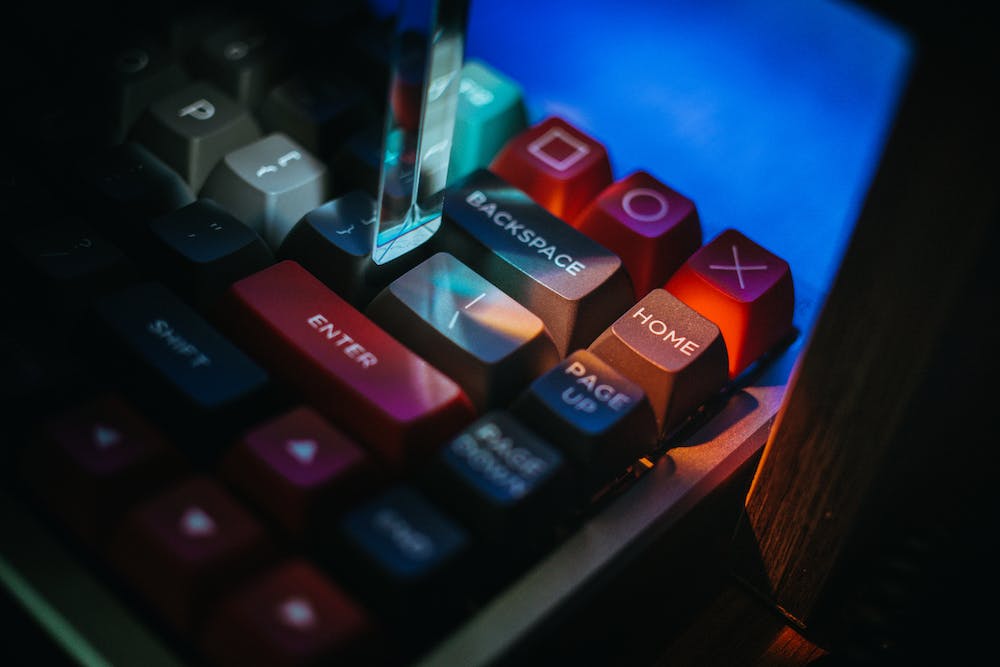
As web applications become more dynamic and interactive, the need for asynchronous data retrieval and display is increasing. AJAX (Asynchronous JavaScript and XML) is a powerful technology that allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. When combined with PHP, a server-side scripting language, IT becomes a powerful tool for creating dynamic web applications. In this article, we will explore the process of implementing AJAX with PHP to create dynamic and responsive web applications.
What is AJAX?
AJAX is a set of web development techniques using many web technologies on the client side to create asynchronous web applications. By asynchronously exchanging small amounts of data with the server, web pages can be updated without the need to reload the entire page. This results in a more responsive user experience and reduces the amount of data transferred between the client and server.
Why Use AJAX with PHP?
PHP is a widely used server-side scripting language that is well-suited for web development. When combined with AJAX, PHP can be used to create dynamic web applications that provide a smooth and responsive user experience. By using AJAX with PHP, you can update specific parts of a web page without reloading the entire page, leading to improved performance and usability.
Implementing AJAX with PHP
Implementing AJAX with PHP involves several steps, including creating a server-side PHP script to handle AJAX requests, writing client-side JavaScript to make AJAX calls, and updating the web page based on the server’s response. Let’s walk through the process of implementing AJAX with PHP.
Step 1: Create a PHP Script for Handling AJAX Requests
The first step in implementing AJAX with PHP is to create a server-side PHP script that can handle AJAX requests. This script will receive data from the client, process it, and return a response. Here’s an example of a simple PHP script that handles an AJAX request to fetch data from a database:
“`php
// Connect to the database
$servername = “localhost”;
$username = “username”;
$password = “password”;
$dbname = “myDB”;
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}
// Fetch data from the database
$sql = “SELECT * FROM myTable”;
$result = $conn->query($sql);
if ($result->num_rows > 0) {
// Output data of each row
while($row = $result->fetch_assoc()) {
echo “Name: ” . $row[“name”]. ” – Email: ” . $row[“email”]. “
“;
}
} else {
echo “0 results”;
}
$conn->close();
?>
“`
In this example, the PHP script connects to a database, fetches data from a table, and returns the data as a response.
Step 2: Write Client-Side JavaScript to Make AJAX Calls
Once you have a PHP script to handle AJAX requests, you need to write client-side JavaScript to make asynchronous calls to the server. This can be done using the XMLHttpRequest object, or by using the fetch API for modern browsers. Here’s an example of how to make an AJAX call using the XMLHttpRequest object:
“`javascript
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById(“data”).innerHTML = this.responseText;
}
};
xhttp.open(“GET”, “ajax.php”, true);
xhttp.send();
“`
In this example, a new XMLHttpRequest object is created, and an asynchronous GET request is made to the “ajax.php” script. When the response is received, the data is inserted into the HTML element with the id “data”.
Step 3: Update the Web Page Based on the Server’s Response
Once the server-side PHP script returns a response to the client, you can update the web page based on the data received. This can be done by manipulating the DOM using JavaScript. For example, you can update the contents of a
“`html
Fetch Data from Database