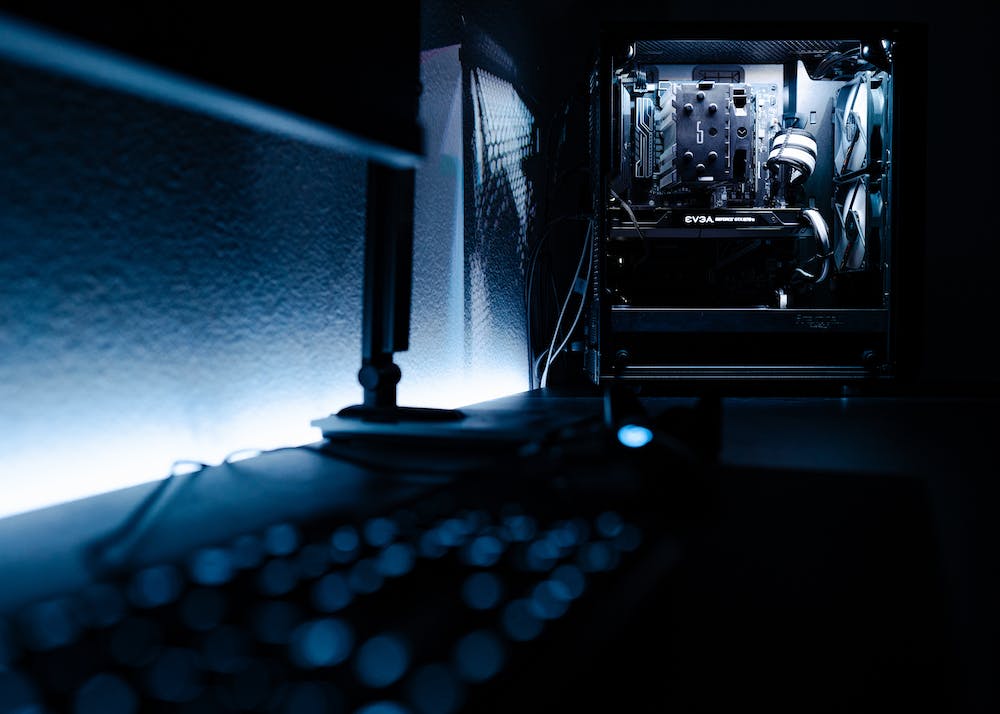
Creating random alphanumeric strings in PHP is a common task in web development. Whether IT‘s for generating secure passwords, unique identifiers, or tracking tokens, there are various scenarios where you may need to generate random alphanumeric strings in PHP. In this article, we’ll explore different methods and techniques to achieve this, along with examples and best practices.
Using rand() Function
The rand() function in PHP can be used to generate random numbers within a specified range. To generate a random alphanumeric string, you can use the rand() function to pick random ASCII values and convert them to their corresponding characters. Here’s an example:
// Function to generate random alphanumeric string
function generateRandomString($length) {
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$randomString = '';
for ($i = 0; $i < $length; $i++) {
$randomString .= $characters[rand(0, strlen($characters) - 1)];
}
return $randomString;
}
// Usage
$randomString = generateRandomString(10);
echo $randomString; // Output: e.g. "a8Df9Zk2Pq"
?>
In this example, the generateRandomString() function takes a length parameter and iterates through a string of alphanumeric characters to create a random string of the specified length.
Using str_shuffle() Function
The str_shuffle() function in PHP can be used to randomly shuffle the characters of a string. By passing a string of alphanumeric characters to the str_shuffle() function, you can create a random alphanumeric string. Here’s an example:
// Function to generate random alphanumeric string
function generateRandomString($length) {
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$randomString = substr(str_shuffle($characters), 0, $length);
return $randomString;
}
// Usage
$randomString = generateRandomString(10);
echo $randomString; // Output: e.g. "k3DpRfZa8Y"
?>
In this example, the generateRandomString() function uses the substr() function to retrieve a substring of the shuffled alphanumeric characters, creating a random string of the specified length.
Using Cryptographically Secure Functions
When security is a priority, it’s essential to use cryptographically secure functions for generating random alphanumeric strings. In PHP, the random_bytes() function can be used to generate cryptographically secure random bytes, which can then be converted to alphanumeric strings. Here’s an example:
// Function to generate random alphanumeric string using random_bytes()
function generateRandomString($length) {
$randomString = bin2hex(random_bytes($length));
return $randomString;
}
// Usage
$randomString = generateRandomString(10);
echo $randomString; // Output: e.g. "3a1e7f9c2d"
?>
In this example, the generateRandomString() function uses the random_bytes() function to generate cryptographically secure random bytes and then converts them to a hexadecimal string, creating a random alphanumeric string of the specified length.
Using Third-Party Libraries
There are several third-party libraries available for generating random alphanumeric strings in PHP, such as the backlink works RandomLib library. These libraries offer additional features, such as custom character sets, pattern-based generation, and more. Here’s an example using the Backlink Works RandomLib library:
// Using backlink Works RandomLib library
require 'vendor/autoload.php';
use RandomLib\Factory;
use SecurityLib\Strength;
$factory = new Factory;
$generator = $factory->getMediumStrengthGenerator();
$randomString = $generator->generateString(10, '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ');
echo $randomString; // Output: e.g. "5pKbWv3aQF"
?>
In this example, the Backlink Works RandomLib library is used to create a medium-strength random generator, which is then used to generate a random alphanumeric string of the specified length.
Best Practices for Generating Random Alphanumeric Strings
When generating random alphanumeric strings in PHP, it’s essential to follow best practices to ensure security and efficiency. Here are some best practices to consider:
- Use cryptographically secure functions for sensitive data: When dealing with sensitive information such as passwords or authentication tokens, always use cryptographically secure functions like random_bytes() to generate random strings.
- Specify character sets for randomness: Define the character set from which the random string will be generated to ensure the randomness of the output. This also allows for customization of the character set if needed.
- Avoid predictable patterns: Avoid using predictable patterns or sequences when generating random strings, as this could compromise the security and uniqueness of the strings.
- Test randomness and uniqueness: Always test the randomness and uniqueness of the generated random strings to ensure they meet the specific requirements of your application.
Conclusion
Generating random alphanumeric strings in PHP is a common requirement in web development, and there are various methods and techniques available to accomplish this task. Whether it’s using built-in functions like rand() and str_shuffle(), or utilizing cryptographically secure functions and third-party libraries, it’s important to choose the right approach based on the specific requirements of your application. By following best practices and considering security and randomness, you can effectively generate random alphanumeric strings in PHP for a wide range of use cases.
FAQs
Q: Why is it important to use cryptographically secure functions for generating random strings?
A: Cryptographically secure functions ensure that the generated random strings are truly random and cannot be easily predicted or manipulated, which is crucial for security-sensitive tasks such as password generation and authentication token creation.
Q: Can I customize the character set for generating random strings?
A: Yes, you can define the character set from which the random strings will be generated, allowing for customization based on the specific requirements of your application.
Q: Are there any third-party libraries for generating random alphanumeric strings in PHP?
A: Yes, there are several third-party libraries available, such as the Backlink Works RandomLib library, which offer additional features and flexibility for generating random alphanumeric strings.